DynamoDB JavaScript SDK v2 v3: aggiungere elementi con DB o DocumentClient – 3
Puoi utilizzare più di un metodo per gestire gli elementi DynamoDB, alcuni sono sconsigliati e altri possono essere utili.
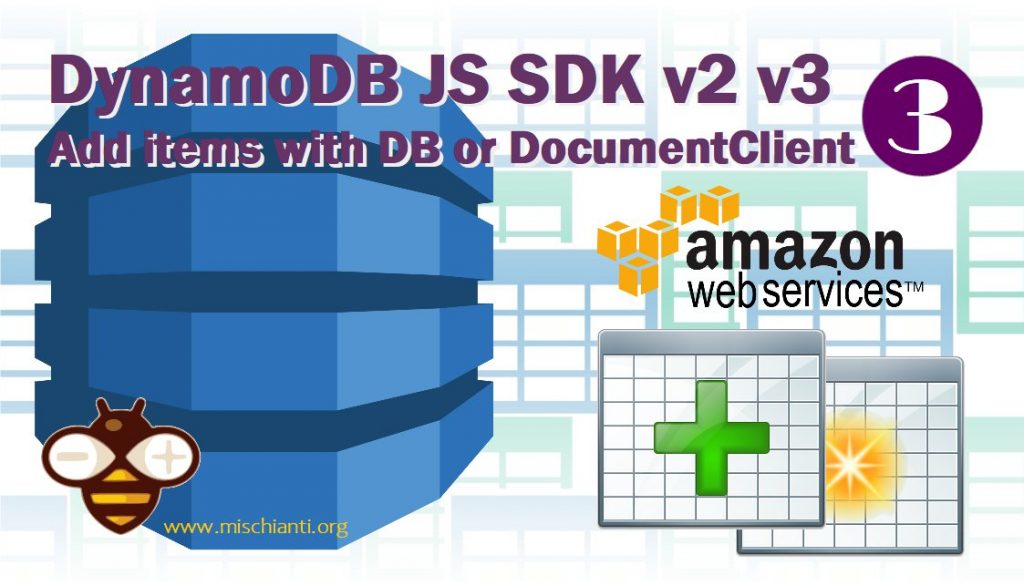
L’approccio logico e la struttura sono cambiati dall’SDK v2 a v3 e analizzeremo la possibilità con il secondo approccio e con la funzione asincrona e sincrona.
Aggiungere un elemento
SDK v2
Per prima cosa creeremo lo script asincrono, come abbiamo visto nelle parti precedenti.
Per eseguire questo script vai nella cartella dynamodb-examples\jsv2
e avvia node item_add.js
/*
* DynamoDB Script Examples
* Add item with DynamoDB
* DB of selected region in AWS.config.update
*
* AUTHOR: Renzo Mischianti
*
* https://mischianti.org/
*
* The MIT License (MIT)
*
* Copyright (c) 2017 Renzo Mischianti www.mischianti.org All right reserved.
*
* You may copy, alter and reuse this code in any way you like, but please leave
* reference to www.mischianti.org in your comments if you redistribute this code.
*/
var AWS = require("aws-sdk");
AWS.config.update({
apiVersion: '2012-08-10',
region: "eu-west-1",
// endpoint: "http://localhost:8000",
// // accessKeyId default can be used while using the downloadable version of DynamoDB.
// // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead.
// accessKeyId: "9oiaf7",
// // secretAccessKey default can be used while using the downloadable version of DynamoDB.
// // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead.
// secretAccessKey: "yz5i9"
});
var ddb = new AWS.DynamoDB();
console.log("Start script!");
var params = {
TableName: "TestTableMischianti",
Item: {
"ItemId": {S: "mischianti"},
"ItemName": {S: "www.mischianti.org"},
"ValueStr": {S: "Mischianti main url"},
"ValueNum": {N: "1"},
}
};
ddb.putItem(params, function (err, data) {
if (err) {
console.error("Unable add item", JSON.stringify(params, null, 2), ". Error JSON:", JSON.stringify(err, null, 2));
} else {
console.log("PutItem succeeded:", JSON.stringify(params, null, 2), data);
}
});
console.log("End script!");
Il risultato in console è
D:\Projects\AlexaProjects\dynamodb-management\dynamodb-examples\jsv2>node item_add.js
Start script!
End script!
PutItem succeeded: {
"TableName": "TestTableMischianti",
"Item": {
"ItemId": {
"S": "mischianti"
},
"ItemName": {
"S": "www.mischianti.org"
},
"ValueStr": {
"S": "Mischianti main url"
},
"ValueNum": {
"N": "1"
}
}
} {}
Verifichiamo la sintassi dei parametri
var params = {
TableName: "TestTableMischianti",
Item: {
"ItemId": {S: "mischianti"},
"ItemName": {S: "www.mischianti.org"},
"ValueStr": {S: "Mischianti main url"},
"ValueNum": {N: "1"},
}
};
dobbiamo specificare la chiave di partizione (controlla l’articolo di creazione della tabella), quindi puoi aggiungere i valori che ti servono, il valore S
o N
corrisponde al tipo di dati S --> String
e N --> Number
.
Ora la versione asincrona ( node item_add_async_await.js
).
/*
* DynamoDB Script Examples
* Add item with DynamoDB async await
* DB of selected region in AWS.config.update
*
* AUTHOR: Renzo Mischianti
*
* https://mischianti.org/
*
* The MIT License (MIT)
*
* Copyright (c) 2017 Renzo Mischianti www.mischianti.org All right reserved.
*
* You may copy, alter and reuse this code in any way you like, but please leave
* reference to www.mischianti.org in your comments if you redistribute this code.
*
*/
var AWS = require("aws-sdk");
AWS.config.update({
apiVersion: '2012-08-10',
region: "eu-west-1",
// endpoint: "http://localhost:8000",
// // accessKeyId default can be used while using the downloadable version of DynamoDB.
// // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead.
// accessKeyId: "9oiaf7",
// // secretAccessKey default can be used while using the downloadable version of DynamoDB.
// // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead.
// secretAccessKey: "yz5i9"
});
var ddb = new AWS.DynamoDB();
(async function () {
console.log("Start script!");
var params = {
TableName: "TestTableMischianti",
Item: {
"ItemId": {S: "mischianti"},
"ItemName": {S: "www.mischianti.org"},
"ValueStr": {S: "Mischianti main url"},
"ValueNum": {N: "1"},
}
};
try {
const data = await ddb.putItem(params).promise();
console.log("PutItem succeeded:", JSON.stringify(params, null, 2), data);
}catch (err) {
console.error("Unable add item", JSON.stringify(params, null, 2), ". Error JSON:", JSON.stringify(err, null, 2));
}
console.log("End script!");})();
Come già descritto la differenza era solo nell’ordine di esecuzione.
DocumentClient
L’oggetto astratto document client semplifica la lettura e la scrittura dei dati su Amazon DynamoDB con l’SDK AWS per JavaScript. Ora puoi utilizzare oggetti JavaScript nativi senza annotarli con tipi di AttributeValue.
Amazon
Ora controlleremo la differenza dall’oggetto DocumentClient ( node item_add_doc_client.js
).
/*
* DynamoDB Script Examples
* Add item with DynamoDB DocumentClient
* DB of selected region in AWS.config.update
*
* AUTHOR: Renzo Mischianti
*
* https://mischianti.org/
*
* The MIT License (MIT)
*
* Copyright (c) 2017 Renzo Mischianti www.mischianti.org All right reserved.
*
* You may copy, alter and reuse this code in any way you like, but please leave
* reference to www.mischianti.org in your comments if you redistribute this code.
*
*/
var AWS = require("aws-sdk");
AWS.config.update({
apiVersion: '2012-08-10',
region: "eu-west-1",
// endpoint: "http://localhost:8000",
// // accessKeyId default can be used while using the downloadable version of DynamoDB.
// // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead.
// accessKeyId: "9oiaf7",
// // secretAccessKey default can be used while using the downloadable version of DynamoDB.
// // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead.
// secretAccessKey: "yz5i9"
});
var docClient = new AWS.DynamoDB.DocumentClient();
console.log("Start script!");
var params = {
TableName: "TestTableMischianti",
Item: {
"ItemId": "mischianti",
"ItemName": "www.mischianti.org",
"ValueStr": "Mischianti main url",
"ValueNum": 1,
}
};
docClient.put(params, function(err, data) {
if (err) {
console.error("Unable add item", JSON.stringify(params, null, 2), ". Error JSON:", JSON.stringify(err, null, 2));
} else {
console.log("PutItem succeeded:", JSON.stringify(params, null, 2), data);
}
});
console.log("End script!");
E qui il risultato della console.
D:\Projects\AlexaProjects\dynamodb-management\dynamodb-examples\jsv2>node item_add_doc_client.js
Start script!
End script!
PutItem succeeded: {
"TableName": "TestTableMischianti",
"Item": {
"ItemId": "mischianti",
"ItemName": "www.mischianti.org",
"ValueStr": "Mischianti main url",
"ValueNum": 1
}
} {}
La differenza è abbastanza semplice, devi guardare la sezione dei parametri
var params = {
TableName: "TestTableMischianti",
Item: {
"ItemId": "mischianti",
"ItemName": "www.mischianti.org",
"ValueStr": "Mischianti main url",
"ValueNum": 1,
}
};
Nessun tipo è definito, il tipo viene dedotto dai dati stessi.
Anche per questo tipo di chiamata può essere una chiamata sincrona ( node item_add_doc_client_async_await.js
).
/*
* DynamoDB Script Examples
* Add item with DynamoDB DocumentClient async await
* DB of selected region in AWS.config.update
*
* AUTHOR: Renzo Mischianti
*
* https://mischianti.org/
*
* The MIT License (MIT)
*
* Copyright (c) 2017 Renzo Mischianti www.mischianti.org All right reserved.
*
* You may copy, alter and reuse this code in any way you like, but please leave
* reference to www.mischianti.org in your comments if you redistribute this code.
*
*/
var AWS = require("aws-sdk");
AWS.config.update({
apiVersion: '2012-08-10',
region: "eu-west-1",
// endpoint: "http://localhost:8000",
// // accessKeyId default can be used while using the downloadable version of DynamoDB.
// // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead.
// accessKeyId: "9oiaf7",
// // secretAccessKey default can be used while using the downloadable version of DynamoDB.
// // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead.
// secretAccessKey: "yz5i9"
});
var docClient = new AWS.DynamoDB.DocumentClient();
(async function () {
console.log("Start script!");
var params = {
TableName: "TestTableMischianti",
Item: {
"ItemId": "mischianti",
"ItemName": "www.mischianti.org",
"ValueStr": "Mischianti main url",
"ValueNum": 1,
}
};
try {
const data = await docClient.put(params).promise();
console.log("PutItem succeeded:", JSON.stringify(params, null, 2), data);
}catch (err) {
console.error("Unable add item", JSON.stringify(params, null, 2), ". Error JSON:", JSON.stringify(err, null, 2));
}
console.log("End script!");
})();
Il risultato della console è lo stesso.
D:\Projects\AlexaProjects\dynamodb-management\dynamodb-examples\jsv2>node item_add_doc_client_async_await.js
Start script!
PutItem succeeded: {
"TableName": "TestTableMischianti",
"Item": {
"ItemId": "mischianti",
"ItemName": "www.mischianti.org",
"ValueStr": "Mischianti main url",
"ValueNum": 1
}
} {}
End script!
SDK v3
Nella versione v3 ci sono alcune modifiche e Amazon consiglia di utilizzare async await (sincrono) con modularizzazione degli import. Ma ricominciamo dalla sintassi dell’ultimo articolo.
Ora la versione asincrona (modalità sconsigliata) dello script di aggiunta.
Per eseguire questo script vai nella cartella dynamodb-examples\jsv3
e avvia node item_add.js
/*
* DynamoDB Script Examples v3
* Add item with DynamoDB
* DB of selected region in configDynamoDB
*
* AUTHOR: Renzo Mischianti
*
* https://mischianti.org/
*
* The MIT License (MIT)
*
* Copyright (c) 2017 Renzo Mischianti www.mischianti.org All right reserved.
*
* You may copy, alter and reuse this code in any way you like, but please leave
* reference to www.mischianti.org in your comments if you redistribute this code.
*
*/
const { DynamoDBClient, PutItemCommand } = require("@aws-sdk/client-dynamodb");
const configDynamoDB = {
version: 'latest',
region: "eu-west-1",
// endpoint: "http://localhost:8000",
// credentials: {
// // accessKeyId default can be used while using the downloadable version of DynamoDB.
// // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead.
// accessKeyId: "9oiaf7",
// // secretAccessKey default can be used while using the downloadable version of DynamoDB.
// // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead.
// secretAccessKey: "yz5i9"
//
// }
};
const dbClient = new DynamoDBClient(configDynamoDB);
const addElement = (params) => {
const command = new PutItemCommand(params);
return dbClient.send(command);
}
console.log("Start script!");
var params = {
TableName: "TestTableMischianti",
Item: {
"ItemId": {S: "mischianti"},
"ItemName": {S: "www.mischianti.org"},
"ValueStr": {S: "Mischianti main url"},
"ValueNum": {N: "1"},
}
};
const command = new PutItemCommand(params);
const data = dbClient.send(command)
.then(
data => console.log("PutItem succeeded:", JSON.stringify(params, null, 2), data)
).catch(
err => console.error("Unable add item", JSON.stringify(params, null, 2), ". Error JSON:", JSON.stringify(err, null, 2))
).finally(
() => console.log("END Of execution!")
);
console.log("End script!");
L’ output della console diventa più dettagliato e abbiamo informazioni aggiuntive.
D:\Projects\AlexaProjects\dynamodb-management\dynamodb-examples\jsv3>node item_add.js
Start script!
End script!
PutItem succeeded: {
"TableName": "TestTableMischianti",
"Item": {
"ItemId": {
"S": "mischianti"
},
"ItemName": {
"S": "www.mischianti.org"
},
"ValueStr": {
"S": "Mischianti main url"
},
"ValueNum": {
"N": "1"
}
}
} { '$metadata':
{ httpStatusCode: 200,
httpHeaders:
{ server: 'Server',
date: 'Fri, 15 Jan 2021 21:38:39 GMT',
'content-type': 'application/x-amz-json-1.0',
'content-length': '2',
connection: 'keep-alive',
'x-amzn-requestid': '035UKDLIR2FGUQ66ISB3UG8K17VV4KQNSO5AEMVJF66Q9ASUAAJG',
'x-amz-crc32': '2745614147' },
requestId: '035UKDLIR2FGUQ66ISB3UG8K17VV4KQNSO5AEMVJF66Q9ASUAAJG',
attempts: 1,
totalRetryDelay: 0 },
Attributes: undefined,
ConsumedCapacity: undefined,
ItemCollectionMetrics: undefined }
END Of execution!
In dettaglio abbiamo il codice http di stato per identificare lo stato della response ( httpStatusCode: 200
), e per lo sviluppatore ora è esplicito che lavoriamo con chiamate REST.
Se vuoi puoi importare direttamente tutto il pacchetto (sconsigliato) così torni alla modalità v2 in termini di gestione dei pacchetti, qui l’esempio.
node item_add_all_package.js
/*
* DynamoDB Script Examples v3
* Add item with DynamoDB Import main package instead single module
* DB of selected region in configDynamoDB
*
* AUTHOR: Renzo Mischianti
*
* https://mischianti.org/
*
* The MIT License (MIT)
*
* Copyright (c) 2017 Renzo Mischianti www.mischianti.org All right reserved.
*
* You may copy, alter and reuse this code in any way you like, but please leave
* reference to www.mischianti.org in your comments if you redistribute this code.
*/
const CDDB = require("@aws-sdk/client-dynamodb");
const configDynamoDB = {
version: 'latest',
region: "eu-west-1",
// endpoint: "http://localhost:8000",
// credentials: {
// // accessKeyId default can be used while using the downloadable version of DynamoDB.
// // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead.
// accessKeyId: "9oiaf7",
// // secretAccessKey default can be used while using the downloadable version of DynamoDB.
// // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead.
// secretAccessKey: "yz5i9"
//
// }
};
const dbClient = new CDDB.DynamoDBClient(configDynamoDB);
const addElement = (params) => {
const command = new PutItemCommand(params);
return dbClient.send(command);
}
console.log("Start script!");
var params = {
TableName: "TestTableMischianti",
Item: {
"ItemId": {S: "mischianti"},
"ItemName": {S: "www.mischianti.org"},
"ValueStr": {S: "Mischianti main url"},
"ValueNum": {N: "1"},
}
};
const command = new CDDB.PutItemCommand(params);
const data = dbClient.send(command)
.then(
data => console.log("PutItem succeeded:", JSON.stringify(params, null, 2), data)
).catch(
err => console.error("Unable add item", JSON.stringify(params, null, 2), ". Error JSON:", JSON.stringify(err, null, 2))
).finally(
() => console.log("END Of execution!")
);
console.log("End script!");
Naturalmente non lo farai, ma non è proibito.
Ora lo script sincrono (node item_add_async_await.js
)
/*
* DynamoDB Script Examples v3
* Add item with DynamoDB async await
* DB of selected region in configDynamoDB
*
* AUTHOR: Renzo Mischianti
*
* https://mischianti.org/
*
* The MIT License (MIT)
*
* Copyright (c) 2017 Renzo Mischianti www.mischianti.org All right reserved.
*
* You may copy, alter and reuse this code in any way you like, but please leave
* reference to www.mischianti.org in your comments if you redistribute this code.
*
*/
const { DynamoDBClient, PutItemCommand } = require("@aws-sdk/client-dynamodb");
const configDynamoDB = {
version: 'latest',
region: "eu-west-1",
// endpoint: "http://localhost:8000",
// credentials: {
// // accessKeyId default can be used while using the downloadable version of DynamoDB.
// // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead.
// accessKeyId: "9oiaf7",
// // secretAccessKey default can be used while using the downloadable version of DynamoDB.
// // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead.
// secretAccessKey: "yz5i9"
//
// }
};
const dbClient = new DynamoDBClient(configDynamoDB);
(async function () {
console.log("Start script!");
var params = {
TableName: "TestTableMischianti",
Item: {
"ItemId": {S: "mischianti"},
"ItemName": {S: "www.mischianti.org"},
"ValueStr": {S: "Mischianti main url"},
"ValueNum": {N: "1"},
}
};
try {
const command = new PutItemCommand(params);
const data = await dbClient.send(command);
console.log("PutItem succeeded:", JSON.stringify(params, null, 2), data);
} catch (err) {
console.error("Unable add item", JSON.stringify(params, null, 2), ". Error JSON:", JSON.stringify(err, null, 2));
}
console.log("End script!");
})();
Puoi anche impedire la noiosa creazione del comando quindi chiamarlo con il comando send, il pacchetto DynamoDB ha tutto il comando al suo interno con dichiarazione implicita dell’oggetto commands.
const ddb = new DynamoDB(configDynamoDB);
[...]
const data = await ddb.putItem(params);
Ma questo approccio è sconsigliato perché riduce la modularizzazione, tutte le dichiarazioni di comando vengono importate e le dimensioni del pacchetto aumentano.
Ma qui un esempio con questo metodo.
/*
* DynamoDB Script Examples v3
* Add item with DynamoDB async await with
* implicit command declaration
* DB of selected region in configDynamoDB
*
* AUTHOR: Renzo Mischianti
*
* https://mischianti.org/
*
* The MIT License (MIT)
*
* Copyright (c) 2017 Renzo Mischianti www.mischianti.org All right reserved.
*
* You may copy, alter and reuse this code in any way you like, but please leave
* reference to www.mischianti.org in your comments if you redistribute this code.
*
*/
const { DynamoDB } = require("@aws-sdk/client-dynamodb");
const configDynamoDB = {
version: 'latest',
region: "eu-west-1",
// endpoint: "http://localhost:8000",
// credentials: {
// // accessKeyId default can be used while using the downloadable version of DynamoDB.
// // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead.
// accessKeyId: "9oiaf7",
// // secretAccessKey default can be used while using the downloadable version of DynamoDB.
// // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead.
// secretAccessKey: "yz5i9"
//
// }
};
const ddb = new DynamoDB(configDynamoDB);
(async function () {
console.log("Start script!");
var params = {
TableName: "TestTableMischianti",
Item: {
"ItemId": {S: "mischianti"},
"ItemName": {S: "www.mischianti.org"},
"ValueStr": {S: "Mischianti main url"},
"ValueNum": {N: "1"},
}
};
try {
const data = await ddb.putItem(params);
console.log("PutItem succeeded:", JSON.stringify(params, null, 2), data);
} catch (err) {
console.error("Unable add item", JSON.stringify(params, null, 2), ". Error JSON:", JSON.stringify(err, null, 2));
}
console.log("End script!");
})();
Controllare i dati
- Puoi trovare tutti i dati nella sezione DynamoDB della console AWS, vai a questo link (controlla la regione).
https://eu-west-1.console.aws.amazon.com/dynamodb/home?region=eu-west-1#
- Fare clic su Tabella/Tabelle;
- Seleziona la tabella TestTableMischianti;
- Fare clic sulla scheda dell’elemento.
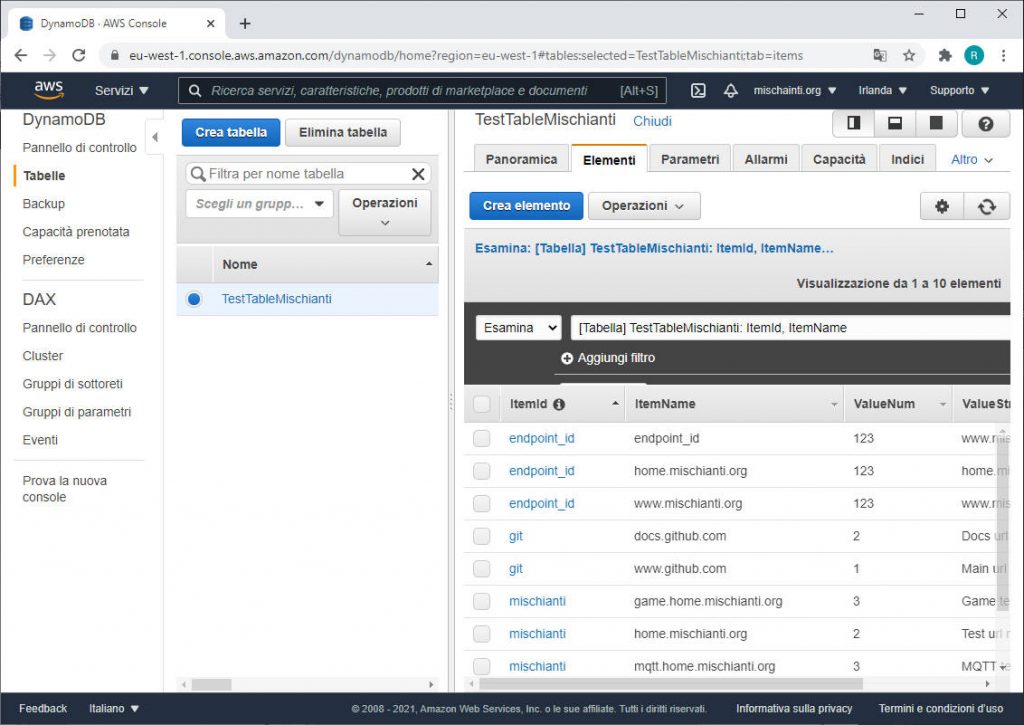
Grazie
- DynamoDB JavaScript SDK v2 v3: prerequisiti ed introduzione
- DynamoDB JavaScript SDK v2 v3: gestione delle tabelle
- DynamoDB JavaScript SDK v2 v3: aggiungere elementi con DB o DocumentClient
- DynamoDB JavaScript SDK v2 v3: gestione degli elementi
- DynamoDB JavaScript SDK v2 v3: scansionare i dati delle tabelle e paginazione
- DynamoDB JavaScript SDK v2 v3: query