esp8266 auto aggiornamenti OTA del firmware da server con controllo della versione – 2
L’aggiornamento OTA (Over the Air) è il processo di caricamento del firmware su un modulo ESP utilizzando una connessione Wi-Fi anziché una porta seriale. Tale funzionalità diventa estremamente utile in caso di accesso fisico limitato o nullo al modulo.
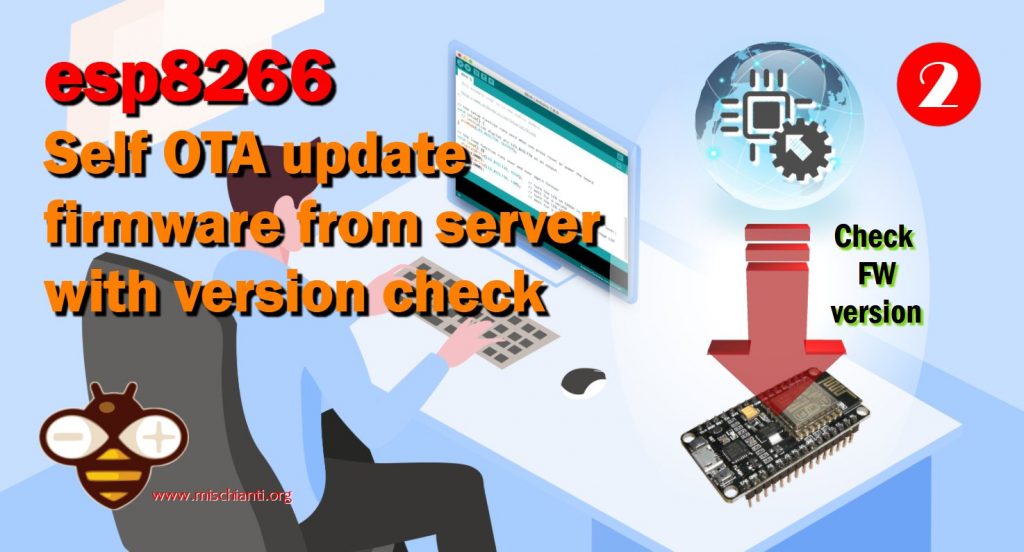
Gli aggiornamenti OTA possono essere effettuati utilizzando:
- Arduino IDE
- Web Browser
- Server HTTP
Prima di tutto leggi il tutorial “esp8266: flash del firmware binario(.bin) compilato e firmato”.
In questo articolo spiegheremo l’aggiornamento OTA con una richiesta REST automatica che avvia il download e flash se la versione del firmware non è aggiornata.
Aggiornamento condizionale OTA (controllo versione) con REST
Per un sistema di aggiornamento firmware più complesso è necessario passare la versione su cui effettuare la verifica.
t_httpUpdate_return ret = ESPhttpUpdate.update(client, "192.168.1.70", 3000, "/update", FIRMWARE_VERSION);
Il codice sopra prevede FIRMWARE_VERSION uguale a 0.2.
Questa chiamata trasporta una serie di informazioni nell’Header della chiamata, quindi se controllate l’Header ricevuta dalla GET update
app.get('/update', (request, response) => {
console.log("List of headers", request.headers);
});
possiamo trovare questi valori
host = "192.168.1.70:3000"
user-agent = "ESP8266-http-Update"
connection = "close"
x-esp8266-chip-id = "7667217"
x-esp8266-sta-mac = "50:02:91:74:FE:11"
x-esp8266-ap-mac = "52:02:91:74:FE:11"
x-esp8266-free-space = "1716224"
x-esp8266-sketch-size = "380144"
x-esp8266-sketch-md5 = "43bc6ba7925555d32aca7222f731c0d9"
x-esp8266-chip-size = "4194304"
x-esp8266-sdk-version = "2.2.2-dev(38a443e)"
x-esp8266-mode = "sketch"
x-esp8266-version = "0.2"
content-length = "0"
In questo caso controlliamo solo il x-esp8266-version
, ma alcune informazioni come lo spazio libero e la versione sdk possono essere molto utili.
/*
* Basic Sketch for automatic update of firmware at start
* with firmware version control
*
* Renzo Mischianti <www.mischianti.org>
*
* https://mischianti.org
*/
#include <Arduino.h>
#include <ESP8266WiFi.h>
#include <ESP8266WiFiMulti.h>
#include <ESP8266HTTPClient.h>
#include <ESP8266httpUpdate.h>
#ifndef APSSID
#define APSSID "<YOUR-SSID>"
#define APPSK "<YOUR-PASSWD>"
#endif
ESP8266WiFiMulti WiFiMulti;
#define FIRMWARE_VERSION "0.3"
void update_started() {
Serial.println("CALLBACK: HTTP update process started");
}
void update_finished() {
Serial.println("CALLBACK: HTTP update process finished");
}
void update_progress(int cur, int total) {
Serial.printf("CALLBACK: HTTP update process at %d of %d bytes...\n", cur, total);
}
void update_error(int err) {
Serial.printf("CALLBACK: HTTP update fatal error code %d\n", err);
}
void setup() {
Serial.begin(115200);
// Serial.setDebugOutput(true);
Serial.println();
Serial.println();
Serial.println();
for (uint8_t t = 4; t > 0; t--) {
Serial.printf("[SETUP] WAIT %d...\n", t);
Serial.flush();
delay(1000);
}
WiFi.mode(WIFI_STA);
WiFiMulti.addAP(APSSID, APPSK);
Serial.print(F("Firmware version "));
Serial.println(FIRMWARE_VERSION);
delay(2000);
}
void loop() {
// wait for WiFi connection
if ((WiFiMulti.run() == WL_CONNECTED)) {
WiFiClient client;
// The line below is optional. It can be used to blink the LED on the board during flashing
// The LED will be on during download of one buffer of data from the network. The LED will
// be off during writing that buffer to flash
// On a good connection the LED should flash regularly. On a bad connection the LED will be
// on much longer than it will be off. Other pins than LED_BUILTIN may be used. The second
// value is used to put the LED on. If the LED is on with HIGH, that value should be passed
ESPhttpUpdate.setLedPin(LED_BUILTIN, LOW);
// Add optional callback notifiers
ESPhttpUpdate.onStart(update_started);
ESPhttpUpdate.onEnd(update_finished);
ESPhttpUpdate.onProgress(update_progress);
ESPhttpUpdate.onError(update_error);
ESPhttpUpdate.rebootOnUpdate(false); // remove automatic update
Serial.println(F("Update start now!"));
// t_httpUpdate_return ret = ESPhttpUpdate.update(client, "http://192.168.1.70:3000/firmware/httpUpdateNew.bin");
// Or:
t_httpUpdate_return ret = ESPhttpUpdate.update(client, "192.168.1.70", 3000, "/update", FIRMWARE_VERSION);
switch (ret) {
case HTTP_UPDATE_FAILED:
Serial.printf("HTTP_UPDATE_FAILD Error (%d): %s\n", ESPhttpUpdate.getLastError(), ESPhttpUpdate.getLastErrorString().c_str());
Serial.println(F("Retry in 10secs!"));
delay(10000); // Wait 10secs
break;
case HTTP_UPDATE_NO_UPDATES:
Serial.println("HTTP_UPDATE_NO_UPDATES");
Serial.println("Your code is up to date!");
delay(10000); // Wait 10secs
break;
case HTTP_UPDATE_OK:
Serial.println("HTTP_UPDATE_OK");
delay(1000); // Wait a second and restart
ESP.restart();
break;
}
}
}
Aggiornare l’end-point con il controllo della versione
Ora aggiungiamo un end-point di aggiornamento con la gestione della versione
const express = require('express');
const { networkInterfaces } = require('os');
const path = require('path');
const app = express();
const nets = networkInterfaces();
// Server port
const PORT = 3000;
app.get('/', (request, response) => response.send('Hello from www.mischianti.org!'));
let downloadCounter = 1;
const LAST_VERSION = 0.3;
app.get('/update', (request, response) => {
const version = (request.header("x-esp8266-version"))?parseFloat(request.header("x-esp8266-version")):Infinity;
if (version<LAST_VERSION){
// If you need an update go here
response.status(200).download(path.join(__dirname, 'firmware/httpUpdateNew.bin'), 'httpUpdateNew.bin', (err)=>{
if (err) {
console.error("Problem on download firmware: ", err)
}else{
downloadCounter++;
}
});
console.log('Your file has been downloaded '+downloadCounter+' times!')
}else{
response.status(304).send('No update needed!')
}
});
app.listen(PORT, () => {
const results = {}; // Or just '{}', an empty object
for (const name of Object.keys(nets)) {
for (const net of nets[name]) {
// Skip over non-IPv4 and internal (i.e. 127.0.0.1) addresses
if (net.family === 'IPv4' && !net.internal) {
if (!results[name]) {
results[name] = [];
}
results[name].push(net.address);
}
}
}
console.log('Listening on port '+PORT+'\n', results)
});
Ora generiamo un nuovo file binario compilato con la versione 0.3 e lo mettiamo sul server
[SETUP] WAIT 4...
[SETUP] WAIT 3...
[SETUP] WAIT 2...
[SETUP] WAIT 1...
Firmware version 0.2
Update start now!
CALLBACK: HTTP update process started
CALLBACK: HTTP update process at 0 of 307888 bytes...
CALLBACK: HTTP update process at 0 of 307888 bytes...
CALLBACK: HTTP update process at 4096 of 307888 bytes...
CALLBACK: HTTP update process at 8192 of 307888 bytes...
CALLBACK: HTTP update process at 12288 of 307888 bytes...
CALLBACK: HTTP update process at 16384 of 307888 bytes...
CALLBACK: HTTP update process at 20480 of 307888 bytes...
CALLBACK: HTTP update process at 24576 of 307888 bytes...
CALLBACK: HTTP update process at 28672 of 307888 bytes...
CALLBACK: HTTP update process at 32768 of 307888 bytes...
CALLBACK: HTTP update process at 36864 of 307888 bytes...
CALLBACK: HTTP update process at 40960 of 307888 bytes...
CALLBACK: HTTP update process at 45056 of 307888 bytes...
CALLBACK: HTTP update process at 49152 of 307888 bytes...
CALLBACK: HTTP update process at 53248 of 307888 bytes...
CALLBACK: HTTP update process at 57344 of 307888 bytes...
CALLBACK: HTTP update process at 61440 of 307888 bytes...
CALLBACK: HTTP update process at 65536 of 307888 bytes...
CALLBACK: HTTP update process at 69632 of 307888 bytes...
CALLBACK: HTTP update process at 73728 of 307888 bytes...
CALLBACK: HTTP update process at 77824 of 307888 bytes...
CALLBACK: HTTP update process at 81920 of 307888 bytes...
CALLBACK: HTTP update process at 86016 of 307888 bytes...
CALLBACK: HTTP update process at 90112 of 307888 bytes...
CALLBACK: HTTP update process at 94208 of 307888 bytes...
CALLBACK: HTTP update process at 98304 of 307888 bytes...
CALLBACK: HTTP update process at 102400 of 307888 bytes...
CALLBACK: HTTP update process at 106496 of 307888 bytes...
CALLBACK: HTTP update process at 110592 of 307888 bytes...
CALLBACK: HTTP update process at 114688 of 307888 bytes...
CALLBACK: HTTP update process at 118784 of 307888 bytes...
CALLBACK: HTTP update process at 122880 of 307888 bytes...
CALLBACK: HTTP update process at 126976 of 307888 bytes...
CALLBACK: HTTP update process at 131072 of 307888 bytes...
CALLBACK: HTTP update process at 135168 of 307888 bytes...
CALLBACK: HTTP update process at 139264 of 307888 bytes...
CALLBACK: HTTP update process at 143360 of 307888 bytes...
CALLBACK: HTTP update process at 147456 of 307888 bytes...
CALLBACK: HTTP update process at 151552 of 307888 bytes...
CALLBACK: HTTP update process at 155648 of 307888 bytes...
CALLBACK: HTTP update process at 159744 of 307888 bytes...
CALLBACK: HTTP update process at 163840 of 307888 bytes...
CALLBACK: HTTP update process at 167936 of 307888 bytes...
CALLBACK: HTTP update process at 172032 of 307888 bytes...
CALLBACK: HTTP update process at 176128 of 307888 bytes...
CALLBACK: HTTP update process at 180224 of 307888 bytes...
CALLBACK: HTTP update process at 184320 of 307888 bytes...
CALLBACK: HTTP update process at 188416 of 307888 bytes...
CALLBACK: HTTP update process at 192512 of 307888 bytes...
CALLBACK: HTTP update process at 196608 of 307888 bytes...
CALLBACK: HTTP update process at 200704 of 307888 bytes...
CALLBACK: HTTP update process at 204800 of 307888 bytes...
CALLBACK: HTTP update process at 208896 of 307888 bytes...
CALLBACK: HTTP update process at 212992 of 307888 bytes...
CALLBACK: HTTP update process at 217088 of 307888 bytes...
CALLBACK: HTTP update process at 221184 of 307888 bytes...
CALLBACK: HTTP update process at 225280 of 307888 bytes...
CALLBACK: HTTP update process at 229376 of 307888 bytes...
CALLBACK: HTTP update process at 233472 of 307888 bytes...
CALLBACK: HTTP update process at 237568 of 307888 bytes...
CALLBACK: HTTP update process at 241664 of 307888 bytes...
CALLBACK: HTTP update process at 245760 of 307888 bytes...
CALLBACK: HTTP update process at 249856 of 307888 bytes...
CALLBACK: HTTP update process at 253952 of 307888 bytes...
CALLBACK: HTTP update process at 258048 of 307888 bytes...
CALLBACK: HTTP update process at 262144 of 307888 bytes...
CALLBACK: HTTP update process at 266240 of 307888 bytes...
CALLBACK: HTTP update process at 270336 of 307888 bytes...
CALLBACK: HTTP update process at 274432 of 307888 bytes...
CALLBACK: HTTP update process at 278528 of 307888 bytes...
CALLBACK: HTTP update process at 282624 of 307888 bytes...
CALLBACK: HTTP update process at 286720 of 307888 bytes...
CALLBACK: HTTP update process at 290816 of 307888 bytes...
CALLBACK: HTTP update process at 294912 of 307888 bytes...
CALLBACK: HTTP update process at 299008 of 307888 bytes...
CALLBACK: HTTP update process at 303104 of 307888 bytes...
CALLBACK: HTTP update process at 307200 of 307888 bytes...
CALLBACK: HTTP update process at 307888 of 307888 bytes...
CALLBACK: HTTP update process at 307888 of 307888 bytes...
CALLBACK: HTTP update process at 307888 of 307888 bytes...
CALLBACK: HTTP update process finished
HTTP_UPDATE_OK
ets Jan 8 2013,rst cause:2, boot mode:(3,6)
load 0x4010f000, len 3460, room 16
tail 4
chksum 0xcc
load 0x3fff20b8, len 40, room 4
tail 4
chksum 0xc9
csum 0xc9
v0004b2b0
@cp:B0
ld
[SETUP] WAIT 4...
[SETUP] WAIT 3...
[SETUP] WAIT 2...
[SETUP] WAIT 1...
Firmware version 0.3
Update start now!
HTTP_UPDATE_NO_UPDATES
Your code is up to date!
Aggiornamento condizionale FileSystem OTA (controllo versione) con REST
Allo stesso modo possiamo gestire l’aggiornamento del filesystem. Stiamo andando veloci ora, perché è la stessa logica dell’aggiornamento del firmware.
Genera file binario FileSystem
Per caricare un contenuto nel filesystem devi installare il relativo plugin, selezionane uno
WeMos D1 mini (esp8266), filesystem SPIFFS integrato (deprecato)
WeMos D1 mini (esp8266), LittleFS Filesystem integrato (consigliato)
Ora aggiungeremo la directory data
alla cartella degli sketch e creeremo un file version.txt
con questo contenuto
0.2
e usa il plugin per caricare.
Nella console troviamo il comando giusto per generare questo file.
[LittleFS] data : D:\Projects\Arduino\sloeber-workspace-OTA\ArduinoOTAesp8266_basic_fs_http_update\data
[LittleFS] size : 2024
[LittleFS] page : 256
[LittleFS] block : 8192
/version.txt
[LittleFS] upload : C:\Users\renzo\AppData\Local\Temp\arduino_build_649994/ArduinoOTAesp8266_basic_fs_http_update.mklittlefs.bin
[LittleFS] address : 0x200000
[LittleFS] reset : --before default_reset --after hard_reset
[LittleFS] port : COM17
[LittleFS] speed : 921600
[LittleFS] python : C:\Users\renzo\AppData\Local\Arduino15\packages\esp8266\tools\python3\3.7.2-post1\python3.exe
[LittleFS] uploader : C:\Users\renzo\AppData\Local\Arduino15\packages\esp8266\hardware\esp8266\3.0.2\tools\upload.py
esptool.py v3.0
Serial port COM17
Connecting....
Chip is ESP8266EX
Features: WiFi
Crystal is 26MHz
MAC: 68:c6:3a:a6:32:e5
Uploading stub...
Running stub...
Stub running...
Changing baud rate to 460800
Changed.
Configuring flash size...
Auto-detected Flash size: 4MB
Compressed 2072576 bytes to 2279...
Writing at 0x00200000... (100 %)
Wrote 2072576 bytes (2279 compressed) at 0x00200000 in 0.1 seconds (effective 312864.3 kbit/s)...
Hash of data verified.
Leaving...
Hard resetting via RTS pin...
[LittleFS] data : D:\Projects\Arduino\sloeber-workspace-OTA\ArduinoOTAesp8266_basic_fs_http_update\data
[LittleFS] size : 2024
[LittleFS] page : 256
[LittleFS] block : 8192
/version.txt
[LittleFS] upload : C:\Users\renzo\AppData\Local\Temp\arduino_build_649994/ArduinoOTAesp8266_basic_fs_http_update.mklittlefs.bin
[LittleFS] address : 0x200000
[LittleFS] reset : --before default_reset --after hard_reset
[LittleFS] port : COM17
[LittleFS] speed : 921600
[LittleFS] python : C:\Users\renzo\AppData\Local\Arduino15\packages\esp8266\tools\python3\3.7.2-post1\python3.exe
[LittleFS] uploader : C:\Users\renzo\AppData\Local\Arduino15\packages\esp8266\hardware\esp8266\3.0.2\tools\upload.py
esptool.py v3.0
Serial port COM17
Ora possiamo prendere molte informazioni, come il file
[LittleFS] upload : C:\Users\renzo\AppData\Local\Temp\arduino_build_649994/ArduinoOTAesp8266_basic_fs_http_update.mklittlefs.bin
Creare sketch
Adesso andiamo a modificare lo sketch già utilizzato per il firmware.
/*
* Sketch for update of filesystem with version control
*
* Renzo Mischianti <www.mischianti.org>
*
* https://mischianti.org
*/
#include <Arduino.h>
#include "LittleFS.h"
#include <ESP8266WiFi.h>
#include <ESP8266WiFiMulti.h>
#include <ESP8266HTTPClient.h>
#include <ESP8266httpUpdate.h>
#ifndef APSSID
#define APSSID "<YOUR-SSID>"
#define APPSK "<YOUR-PASSWD>"
#endif
ESP8266WiFiMulti WiFiMulti;
#define FIRMWARE_VERSION "0.2"
String FILESYSTEM_VERSION = "0.0";
void update_started() {
Serial.println("CALLBACK: HTTP update process started");
}
void update_finished() {
Serial.println("CALLBACK: HTTP update process finished");
}
void update_progress(int cur, int total) {
Serial.printf("CALLBACK: HTTP update process at %d of %d bytes...\n", cur, total);
}
void update_error(int err) {
Serial.printf("CALLBACK: HTTP update fatal error code %d\n", err);
}
void setup() {
Serial.begin(115200);
// Serial.setDebugOutput(true);
Serial.println();
Serial.println();
Serial.println();
for (uint8_t t = 4; t > 0; t--) {
Serial.printf("[SETUP] WAIT %d...\n", t);
Serial.flush();
delay(1000);
}
WiFi.mode(WIFI_STA);
WiFiMulti.addAP(APSSID, APPSK);
Serial.print(F("Firmware version "));
Serial.println(FIRMWARE_VERSION);
Serial.print(F("Inizializing FS..."));
if (LittleFS.begin()){
Serial.println(F("done."));
}else{
Serial.println(F("fail."));
}
Serial.print(F("FileSystem version "));
File versionFile = LittleFS.open(F("/version.txt"), "r");
if (versionFile) {
FILESYSTEM_VERSION = versionFile.readString();
versionFile.close();
}
Serial.println(FILESYSTEM_VERSION);
delay(2000);
}
void loop() {
// wait for WiFi connection
if ((WiFiMulti.run() == WL_CONNECTED)) {
WiFiClient client;
// The line below is optional. It can be used to blink the LED on the board during flashing
// The LED will be on during download of one buffer of data from the network. The LED will
// be off during writing that buffer to flash
// On a good connection the LED should flash regularly. On a bad connection the LED will be
// on much longer than it will be off. Other pins than LED_BUILTIN may be used. The second
// value is used to put the LED on. If the LED is on with HIGH, that value should be passed
ESPhttpUpdate.setLedPin(LED_BUILTIN, LOW);
// Add optional callback notifiers
ESPhttpUpdate.onStart(update_started);
ESPhttpUpdate.onEnd(update_finished);
ESPhttpUpdate.onProgress(update_progress);
ESPhttpUpdate.onError(update_error);
ESPhttpUpdate.rebootOnUpdate(false); // remove automatic update
Serial.println(F("Update start now!"));
// t_httpUpdate_return ret = ESPhttpUpdate.update(client, "http://192.168.1.70:3000/firmware/httpUpdateNew.bin");
// Or:
t_httpUpdate_return ret = ESPhttpUpdate.updateFS(client, "http://192.168.1.70:3000/updateFS", FILESYSTEM_VERSION);
switch (ret) {
case HTTP_UPDATE_FAILED:
Serial.printf("HTTP_UPDATE_FAILD Error (%d): %s\n", ESPhttpUpdate.getLastError(), ESPhttpUpdate.getLastErrorString().c_str());
Serial.println(F("Retry in 10secs!"));
delay(10000); // Wait 10secs
break;
case HTTP_UPDATE_NO_UPDATES:
Serial.println("HTTP_UPDATE_NO_UPDATES");
Serial.println("Your code is up to date!");
delay(10000); // Wait 10secs
break;
case HTTP_UPDATE_OK:
Serial.println("HTTP_UPDATE_OK");
delay(1000); // Wait a second and restart
ESP.restart();
break;
}
}
}
Recupero la versione del filesystem dal file version.txt
e la metto sulla variabile FILESYSTEM_VERSION
.
Serial.print(F("Inizializing FS..."));
if (LittleFS.begin()){
Serial.println(F("done."));
}else{
Serial.println(F("fail."));
}
Serial.print(F("FileSystem version "));
File versionFile = LittleFS.open(F("/version.txt"), "r");
if (versionFile) {
FILESYSTEM_VERSION = versionFile.readString();
versionFile.close();
}
Serial.println(FILESYSTEM_VERSION);
Creare l’end-point sul server
Ora creeremo un nuovo end-point /updateFS
in GET che controlla la versione del filesystem ottenuto dal file version.txt
tramite la variabile LAST_FS_VERSION
.
const express = require('express');
const { networkInterfaces } = require('os');
const path = require('path');
const app = express();
const nets = networkInterfaces();
// Server port
const PORT = 3000;
app.get('/', (request, response) => response.send('Hello from www.mischianti.org!'));
let downloadCounter = 1;
const LAST_VERSION = 0.3;
app.get('/update', (request, response) => {
const version = (request.header("x-esp8266-version"))?parseFloat(request.header("x-esp8266-version")):Infinity;
if (version<LAST_VERSION){
// If you need an update go here
response.status(200).download(path.join(__dirname, 'firmware/httpUpdateNew.bin'), 'httpUpdateNew.bin', (err)=>{
if (err) {
console.error("Problem on download firmware: ", err)
}else{
downloadCounter++;
}
});
console.log('Your file has been downloaded '+downloadCounter+' times!')
}else{
response.status(304).send('No update needed!')
}
});
let downloadFSCounter = 1;
const LAST_FS_VERSION = 0.3;
app.get('/updateFS', (request, response) => {
const version = (request.header("x-esp8266-version"))?parseFloat(request.header("x-esp8266-version")):Infinity;
if (version<LAST_FS_VERSION){
// If you need an update go here
response.status(200).download(path.join(__dirname, 'filesystem/httpUpdateNewFS.bin'), 'httpUpdateNewFS.bin', (err)=>{
if (err) {
console.error("Problem on download filesystem: ", err)
}else{
downloadFSCounter++;
}
});
console.log('Your file FS has been downloaded '+downloadFSCounter+' times!')
}else{
response.status(304).send('No FS update needed!')
}
});
app.listen(PORT, () => {
const results = {}; // Or just '{}', an empty object
for (const name of Object.keys(nets)) {
for (const net of nets[name]) {
// Skip over non-IPv4 and internal (i.e. 127.0.0.1) addresses
if (net.family === 'IPv4' && !net.internal) {
if (!results[name]) {
results[name] = [];
}
results[name].push(net.address);
}
}
}
console.log('Listening on port '+PORT+'\n', results)
});
Ora modificheremo il file version.txt
con la versione 0.2, quindi lo caricheremo sul dispositivo:
- In OTAServer crea la cartella filesystem,
- Cambia la versione in
0.3
inversion.txt
, rigenera senza caricare e copia il fileArduinoOTAesp8266_fs_update.mklittlefs.bin
nella cartella - Rinomina in
httpUpdateNewFS.bin
.
Il risultato nell’output seriale diventa così.
[SETUP] WAIT 4...
[SETUP] WAIT 3...
[SETUP] WAIT 2...
[SETUP] WAIT 1...
Firmware version 0.2
Inizializing FS...done.
FileSystem version 0.2
Update start now!
CALLBACK: HTTP update process started
CALLBACK: HTTP update process at 0 of 2072576 bytes...
CALLBACK: HTTP update process at 0 of 2072576 bytes...
CALLBACK: HTTP update process at 4096 of 2072576 bytes...
CALLBACK: HTTP update process at 8192 of 2072576 bytes...
CALLBACK: HTTP update process at 12288 of 2072576 bytes...
CALLBACK: HTTP update process at 16384 of 2072576 bytes...
CALLBACK: HTTP update process at 20480 of 2072576 bytes...
CALLBACK: HTTP update process at 24576 of 2072576 bytes...
[...]
CALLBACK: HTTP update process at 2031616 of 2072576 bytes...
CALLBACK: HTTP update process at 2035712 of 2072576 bytes...
CALLBACK: HTTP update process at 2039808 of 2072576 bytes...
CALLBACK: HTTP update process at 2043904 of 2072576 bytes...
CALLBACK: HTTP update process at 2048000 of 2072576 bytes...
CALLBACK: HTTP update process at 2052096 of 2072576 bytes...
CALLBACK: HTTP update process at 2056192 of 2072576 bytes...
CALLBACK: HTTP update process at 2060288 of 2072576 bytes...
CALLBACK: HTTP update process at 2064384 of 2072576 bytes...
CALLBACK: HTTP update process at 2068480 of 2072576 bytes...
CALLBACK: HTTP update process at 2072576 of 2072576 bytes...
CALLBACK: HTTP update process at 2072576 of 2072576 bytes...
CALLBACK: HTTP update process at 2072576 of 2072576 bytes...
CALLBACK: HTTP update process finished
HTTP_UPDATE_OK
ets Jan 8 2013,rst cause:2, boot mode:(3,6)
load 0x4010f000, len 3460, room 16
tail 4
chksum 0xcc
load 0x3fff20b8, len 40, room 4
tail 4
chksum 0xc9
csum 0xc9
v000650f0
~ld
[SETUP] WAIT 4...
[SETUP] WAIT 3...
[SETUP] WAIT 2...
[SETUP] WAIT 1...
Firmware version 0.2
Inizializing FS...done.
FileSystem version 0.3
Update start now!
HTTP_UPDATE_NO_UPDATES
Your code is up to date!
Grazie
- Firmware and OTA update
- Gestione del firmware
- Aggiornamenti OTA con Arduino IDE
- Aggiornamenti OTA con Web Browser
- Aggiornamenti OTA automatico da server HTTP
- Aggiornamenti firmware non standard