esp8266 self OTA update firmware from server with version check – 2
OTA (Over the Air) update is uploading firmware to an ESP module using a Wi-Fi connection rather than a serial port. Such functionality becomes extremely useful in case of limited or no physical access to the module.
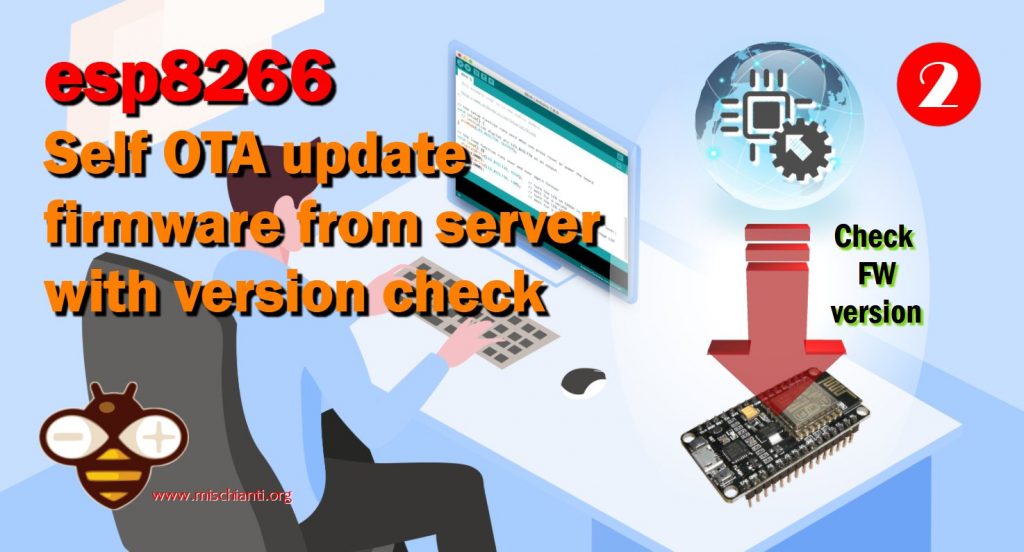
OTA may be done using:
- Arduino IDE
- Web Browser
- HTTP Server
First of all, read the tutorial “esp8266: flash firmware binary (.bin) compiled and signed“.
This article will explain the OTA update with an automatic REST request that starts the download and flash if the firmware if the version is not updated.
Conditionally OTA (version control) update with REST
For a more complex firmware update system, it is necessary to pass the version to be checked.
t_httpUpdate_return ret = ESPhttpUpdate.update(client, "192.168.1.70", 3000, "/update", FIRMWARE_VERSION);
The code above expects FIRMWARE_VERSION equal to 0.2.
This call transport a set of information in the header of the call, so if we check the received header by the update
GET
app.get('/update', (request, response) => {
console.log("List of headers", request.headers);
});
we can find these values
host = "192.168.1.70:3000"
user-agent = "ESP8266-http-Update"
connection = "close"
x-esp8266-chip-id = "7667217"
x-esp8266-sta-mac = "50:02:91:74:FE:11"
x-esp8266-ap-mac = "52:02:91:74:FE:11"
x-esp8266-free-space = "1716224"
x-esp8266-sketch-size = "380144"
x-esp8266-sketch-md5 = "43bc6ba7925555d32aca7222f731c0d9"
x-esp8266-chip-size = "4194304"
x-esp8266-sdk-version = "2.2.2-dev(38a443e)"
x-esp8266-mode = "sketch"
x-esp8266-version = "0.2"
content-length = "0"
In this case, we check only the, but some information like free space and SDK version can be very useful.
/*
* Basic Sketch for automatic update of firmware at start
* with firmware version control
*
* Renzo Mischianti <www.mischianti.org>
*
* https://mischianti.org
*/
#include <Arduino.h>
#include <ESP8266WiFi.h>
#include <ESP8266WiFiMulti.h>
#include <ESP8266HTTPClient.h>
#include <ESP8266httpUpdate.h>
#ifndef APSSID
#define APSSID "<YOUR-SSID>"
#define APPSK "<YOUR-PASSWD>"
#endif
ESP8266WiFiMulti WiFiMulti;
#define FIRMWARE_VERSION "0.3"
void update_started() {
Serial.println("CALLBACK: HTTP update process started");
}
void update_finished() {
Serial.println("CALLBACK: HTTP update process finished");
}
void update_progress(int cur, int total) {
Serial.printf("CALLBACK: HTTP update process at %d of %d bytes...\n", cur, total);
}
void update_error(int err) {
Serial.printf("CALLBACK: HTTP update fatal error code %d\n", err);
}
void setup() {
Serial.begin(115200);
// Serial.setDebugOutput(true);
Serial.println();
Serial.println();
Serial.println();
for (uint8_t t = 4; t > 0; t--) {
Serial.printf("[SETUP] WAIT %d...\n", t);
Serial.flush();
delay(1000);
}
WiFi.mode(WIFI_STA);
WiFiMulti.addAP(APSSID, APPSK);
Serial.print(F("Firmware version "));
Serial.println(FIRMWARE_VERSION);
delay(2000);
}
void loop() {
// wait for WiFi connection
if ((WiFiMulti.run() == WL_CONNECTED)) {
WiFiClient client;
// The line below is optional. It can be used to blink the LED on the board during flashing
// The LED will be on during download of one buffer of data from the network. The LED will
// be off during writing that buffer to flash
// On a good connection the LED should flash regularly. On a bad connection the LED will be
// on much longer than it will be off. Other pins than LED_BUILTIN may be used. The second
// value is used to put the LED on. If the LED is on with HIGH, that value should be passed
ESPhttpUpdate.setLedPin(LED_BUILTIN, LOW);
// Add optional callback notifiers
ESPhttpUpdate.onStart(update_started);
ESPhttpUpdate.onEnd(update_finished);
ESPhttpUpdate.onProgress(update_progress);
ESPhttpUpdate.onError(update_error);
ESPhttpUpdate.rebootOnUpdate(false); // remove automatic update
Serial.println(F("Update start now!"));
// t_httpUpdate_return ret = ESPhttpUpdate.update(client, "http://192.168.1.70:3000/firmware/httpUpdateNew.bin");
// Or:
t_httpUpdate_return ret = ESPhttpUpdate.update(client, "192.168.1.70", 3000, "/update", FIRMWARE_VERSION);
switch (ret) {
case HTTP_UPDATE_FAILED:
Serial.printf("HTTP_UPDATE_FAILD Error (%d): %s\n", ESPhttpUpdate.getLastError(), ESPhttpUpdate.getLastErrorString().c_str());
Serial.println(F("Retry in 10secs!"));
delay(10000); // Wait 10secs
break;
case HTTP_UPDATE_NO_UPDATES:
Serial.println("HTTP_UPDATE_NO_UPDATES");
Serial.println("Your code is up to date!");
delay(10000); // Wait 10secs
break;
case HTTP_UPDATE_OK:
Serial.println("HTTP_UPDATE_OK");
delay(1000); // Wait a second and restart
ESP.restart();
break;
}
}
}
Update end point with version control
Now let’s add an updated endpoint with version management
const express = require('express');
const { networkInterfaces } = require('os');
const path = require('path');
const app = express();
const nets = networkInterfaces();
// Server port
const PORT = 3000;
app.get('/', (request, response) => response.send('Hello from www.mischianti.org!'));
let downloadCounter = 1;
const LAST_VERSION = 0.3;
app.get('/update', (request, response) => {
const version = (request.header("x-esp8266-version"))?parseFloat(request.header("x-esp8266-version")):Infinity;
if (version<LAST_VERSION){
// If you need an update go here
response.status(200).download(path.join(__dirname, 'firmware/httpUpdateNew.bin'), 'httpUpdateNew.bin', (err)=>{
if (err) {
console.error("Problem on download firmware: ", err)
}else{
downloadCounter++;
}
});
console.log('Your file has been downloaded '+downloadCounter+' times!')
}else{
response.status(304).send('No update needed!')
}
});
app.listen(PORT, () => {
const results = {}; // Or just '{}', an empty object
for (const name of Object.keys(nets)) {
for (const net of nets[name]) {
// Skip over non-IPv4 and internal (i.e. 127.0.0.1) addresses
if (net.family === 'IPv4' && !net.internal) {
if (!results[name]) {
results[name] = [];
}
results[name].push(net.address);
}
}
}
console.log('Listening on port '+PORT+'\n', results)
});
Now we generate a new compiled binary file with version 0.3 and put It on the server
[SETUP] WAIT 4...
[SETUP] WAIT 3...
[SETUP] WAIT 2...
[SETUP] WAIT 1...
Firmware version 0.2
Update start now!
CALLBACK: HTTP update process started
CALLBACK: HTTP update process at 0 of 307888 bytes...
CALLBACK: HTTP update process at 0 of 307888 bytes...
CALLBACK: HTTP update process at 4096 of 307888 bytes...
CALLBACK: HTTP update process at 8192 of 307888 bytes...
CALLBACK: HTTP update process at 12288 of 307888 bytes...
CALLBACK: HTTP update process at 16384 of 307888 bytes...
CALLBACK: HTTP update process at 20480 of 307888 bytes...
CALLBACK: HTTP update process at 24576 of 307888 bytes...
CALLBACK: HTTP update process at 28672 of 307888 bytes...
CALLBACK: HTTP update process at 32768 of 307888 bytes...
CALLBACK: HTTP update process at 36864 of 307888 bytes...
CALLBACK: HTTP update process at 40960 of 307888 bytes...
CALLBACK: HTTP update process at 45056 of 307888 bytes...
CALLBACK: HTTP update process at 49152 of 307888 bytes...
CALLBACK: HTTP update process at 53248 of 307888 bytes...
CALLBACK: HTTP update process at 57344 of 307888 bytes...
CALLBACK: HTTP update process at 61440 of 307888 bytes...
CALLBACK: HTTP update process at 65536 of 307888 bytes...
CALLBACK: HTTP update process at 69632 of 307888 bytes...
CALLBACK: HTTP update process at 73728 of 307888 bytes...
CALLBACK: HTTP update process at 77824 of 307888 bytes...
CALLBACK: HTTP update process at 81920 of 307888 bytes...
CALLBACK: HTTP update process at 86016 of 307888 bytes...
CALLBACK: HTTP update process at 90112 of 307888 bytes...
CALLBACK: HTTP update process at 94208 of 307888 bytes...
CALLBACK: HTTP update process at 98304 of 307888 bytes...
CALLBACK: HTTP update process at 102400 of 307888 bytes...
CALLBACK: HTTP update process at 106496 of 307888 bytes...
CALLBACK: HTTP update process at 110592 of 307888 bytes...
CALLBACK: HTTP update process at 114688 of 307888 bytes...
CALLBACK: HTTP update process at 118784 of 307888 bytes...
CALLBACK: HTTP update process at 122880 of 307888 bytes...
CALLBACK: HTTP update process at 126976 of 307888 bytes...
CALLBACK: HTTP update process at 131072 of 307888 bytes...
CALLBACK: HTTP update process at 135168 of 307888 bytes...
CALLBACK: HTTP update process at 139264 of 307888 bytes...
CALLBACK: HTTP update process at 143360 of 307888 bytes...
CALLBACK: HTTP update process at 147456 of 307888 bytes...
CALLBACK: HTTP update process at 151552 of 307888 bytes...
CALLBACK: HTTP update process at 155648 of 307888 bytes...
CALLBACK: HTTP update process at 159744 of 307888 bytes...
CALLBACK: HTTP update process at 163840 of 307888 bytes...
CALLBACK: HTTP update process at 167936 of 307888 bytes...
CALLBACK: HTTP update process at 172032 of 307888 bytes...
CALLBACK: HTTP update process at 176128 of 307888 bytes...
CALLBACK: HTTP update process at 180224 of 307888 bytes...
CALLBACK: HTTP update process at 184320 of 307888 bytes...
CALLBACK: HTTP update process at 188416 of 307888 bytes...
CALLBACK: HTTP update process at 192512 of 307888 bytes...
CALLBACK: HTTP update process at 196608 of 307888 bytes...
CALLBACK: HTTP update process at 200704 of 307888 bytes...
CALLBACK: HTTP update process at 204800 of 307888 bytes...
CALLBACK: HTTP update process at 208896 of 307888 bytes...
CALLBACK: HTTP update process at 212992 of 307888 bytes...
CALLBACK: HTTP update process at 217088 of 307888 bytes...
CALLBACK: HTTP update process at 221184 of 307888 bytes...
CALLBACK: HTTP update process at 225280 of 307888 bytes...
CALLBACK: HTTP update process at 229376 of 307888 bytes...
CALLBACK: HTTP update process at 233472 of 307888 bytes...
CALLBACK: HTTP update process at 237568 of 307888 bytes...
CALLBACK: HTTP update process at 241664 of 307888 bytes...
CALLBACK: HTTP update process at 245760 of 307888 bytes...
CALLBACK: HTTP update process at 249856 of 307888 bytes...
CALLBACK: HTTP update process at 253952 of 307888 bytes...
CALLBACK: HTTP update process at 258048 of 307888 bytes...
CALLBACK: HTTP update process at 262144 of 307888 bytes...
CALLBACK: HTTP update process at 266240 of 307888 bytes...
CALLBACK: HTTP update process at 270336 of 307888 bytes...
CALLBACK: HTTP update process at 274432 of 307888 bytes...
CALLBACK: HTTP update process at 278528 of 307888 bytes...
CALLBACK: HTTP update process at 282624 of 307888 bytes...
CALLBACK: HTTP update process at 286720 of 307888 bytes...
CALLBACK: HTTP update process at 290816 of 307888 bytes...
CALLBACK: HTTP update process at 294912 of 307888 bytes...
CALLBACK: HTTP update process at 299008 of 307888 bytes...
CALLBACK: HTTP update process at 303104 of 307888 bytes...
CALLBACK: HTTP update process at 307200 of 307888 bytes...
CALLBACK: HTTP update process at 307888 of 307888 bytes...
CALLBACK: HTTP update process at 307888 of 307888 bytes...
CALLBACK: HTTP update process at 307888 of 307888 bytes...
CALLBACK: HTTP update process finished
HTTP_UPDATE_OK
ets Jan 8 2013,rst cause:2, boot mode:(3,6)
load 0x4010f000, len 3460, room 16
tail 4
chksum 0xcc
load 0x3fff20b8, len 40, room 4
tail 4
chksum 0xc9
csum 0xc9
v0004b2b0
@cp:B0
ld
[SETUP] WAIT 4...
[SETUP] WAIT 3...
[SETUP] WAIT 2...
[SETUP] WAIT 1...
Firmware version 0.3
Update start now!
HTTP_UPDATE_NO_UPDATES
Your code is up to date!
Conditionally FileSystem OTA (version control) update with REST
In the same manner, we can manage filesystem updates. We are going fast now because It’s the same firmware update logic.
Generate FileSystem binary file
To manage the filesystem, we can use the same way, but first, you must read one of the following guides:
WeMos D1 mini (esp8266), integrated SPIFFS Filesystem (deprecated)
WeMos D1 mini (esp8266), integrated LittleFS Filesystem (recommended)
Now we are going to add data
directory to the sketch folder, and we create a file version.txt
with this content
0.2
and use the plugin to upload.
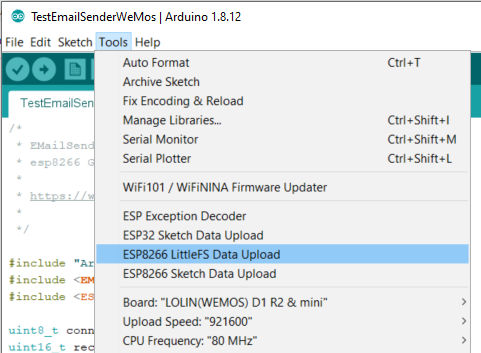
In the console, we find the right command to generate this file.
[LittleFS] data : D:\Projects\Arduino\sloeber-workspace-OTA\ArduinoOTAesp8266_basic_fs_http_update\data
[LittleFS] size : 2024
[LittleFS] page : 256
[LittleFS] block : 8192
/version.txt
[LittleFS] upload : C:\Users\renzo\AppData\Local\Temp\arduino_build_649994/ArduinoOTAesp8266_fs_http_update.mklittlefs.bin
[LittleFS] address : 0x200000
[LittleFS] reset : --before default_reset --after hard_reset
[LittleFS] port : COM17
[LittleFS] speed : 921600
[LittleFS] python : C:\Users\renzo\AppData\Local\Arduino15\packages\esp8266\tools\python3\3.7.2-post1\python3.exe
[LittleFS] uploader : C:\Users\renzo\AppData\Local\Arduino15\packages\esp8266\hardware\esp8266\3.0.2\tools\upload.py
esptool.py v3.0
Serial port COM17
Connecting....
Chip is ESP8266EX
Features: WiFi
Crystal is 26MHz
MAC: 68:c6:3a:a6:32:e5
Uploading stub...
Running stub...
Stub running...
Changing baud rate to 460800
Changed.
Configuring flash size...
Auto-detected Flash size: 4MB
Compressed 2072576 bytes to 2279...
Writing at 0x00200000... (100 %)
Wrote 2072576 bytes (2279 compressed) at 0x00200000 in 0.1 seconds (effective 312864.3 kbit/s)...
Hash of data verified.
Leaving...
Hard resetting via RTS pin...
[LittleFS] data : D:\Projects\Arduino\sloeber-workspace-OTA\ArduinoOTAesp8266_basic_fs_http_update\data
[LittleFS] size : 2024
[LittleFS] page : 256
[LittleFS] block : 8192
/version.txt
[LittleFS] upload : C:\Users\renzo\AppData\Local\Temp\arduino_build_649994/ArduinoOTAesp8266_fs_http_update.mklittlefs.bin
[LittleFS] address : 0x200000
[LittleFS] reset : --before default_reset --after hard_reset
[LittleFS] port : COM17
[LittleFS] speed : 921600
[LittleFS] python : C:\Users\renzo\AppData\Local\Arduino15\packages\esp8266\tools\python3\3.7.2-post1\python3.exe
[LittleFS] uploader : C:\Users\renzo\AppData\Local\Arduino15\packages\esp8266\hardware\esp8266\3.0.2\tools\upload.py
esptool.py v3.0
Serial port COM17
Now we can grab a lot of information; first, the file
[LittleFS] upload : C:\Users\renzo\AppData\Local\Temp\arduino_build_649994/ArduinoOTAesp8266_fs_http_update.mklittlefs.bin
Create sketch
Now we are going to modify the sketch already used for firmware.
/*
* Sketch for update of filesystem with version control
*
* Renzo Mischianti <www.mischianti.org>
*
* https://mischianti.org
*/
#include <Arduino.h>
#include "LittleFS.h"
#include <ESP8266WiFi.h>
#include <ESP8266WiFiMulti.h>
#include <ESP8266HTTPClient.h>
#include <ESP8266httpUpdate.h>
#ifndef APSSID
#define APSSID "<YOUR-SSID>"
#define APPSK "<YOUR-PASSWD>"
#endif
ESP8266WiFiMulti WiFiMulti;
#define FIRMWARE_VERSION "0.2"
String FILESYSTEM_VERSION = "0.0";
void update_started() {
Serial.println("CALLBACK: HTTP update process started");
}
void update_finished() {
Serial.println("CALLBACK: HTTP update process finished");
}
void update_progress(int cur, int total) {
Serial.printf("CALLBACK: HTTP update process at %d of %d bytes...\n", cur, total);
}
void update_error(int err) {
Serial.printf("CALLBACK: HTTP update fatal error code %d\n", err);
}
void setup() {
Serial.begin(115200);
// Serial.setDebugOutput(true);
Serial.println();
Serial.println();
Serial.println();
for (uint8_t t = 4; t > 0; t--) {
Serial.printf("[SETUP] WAIT %d...\n", t);
Serial.flush();
delay(1000);
}
WiFi.mode(WIFI_STA);
WiFiMulti.addAP(APSSID, APPSK);
Serial.print(F("Firmware version "));
Serial.println(FIRMWARE_VERSION);
Serial.print(F("Inizializing FS..."));
if (LittleFS.begin()){
Serial.println(F("done."));
}else{
Serial.println(F("fail."));
}
Serial.print(F("FileSystem version "));
File versionFile = LittleFS.open(F("/version.txt"), "r");
if (versionFile) {
FILESYSTEM_VERSION = versionFile.readString();
versionFile.close();
}
Serial.println(FILESYSTEM_VERSION);
delay(2000);
}
void loop() {
// wait for WiFi connection
if ((WiFiMulti.run() == WL_CONNECTED)) {
WiFiClient client;
// The line below is optional. It can be used to blink the LED on the board during flashing
// The LED will be on during download of one buffer of data from the network. The LED will
// be off during writing that buffer to flash
// On a good connection the LED should flash regularly. On a bad connection the LED will be
// on much longer than it will be off. Other pins than LED_BUILTIN may be used. The second
// value is used to put the LED on. If the LED is on with HIGH, that value should be passed
ESPhttpUpdate.setLedPin(LED_BUILTIN, LOW);
// Add optional callback notifiers
ESPhttpUpdate.onStart(update_started);
ESPhttpUpdate.onEnd(update_finished);
ESPhttpUpdate.onProgress(update_progress);
ESPhttpUpdate.onError(update_error);
ESPhttpUpdate.rebootOnUpdate(false); // remove automatic update
Serial.println(F("Update start now!"));
// t_httpUpdate_return ret = ESPhttpUpdate.update(client, "http://192.168.1.70:3000/firmware/httpUpdateNew.bin");
// Or:
t_httpUpdate_return ret = ESPhttpUpdate.updateFS(client, "http://192.168.1.70:3000/updateFS", FILESYSTEM_VERSION);
switch (ret) {
case HTTP_UPDATE_FAILED:
Serial.printf("HTTP_UPDATE_FAILD Error (%d): %s\n", ESPhttpUpdate.getLastError(), ESPhttpUpdate.getLastErrorString().c_str());
Serial.println(F("Retry in 10secs!"));
delay(10000); // Wait 10secs
break;
case HTTP_UPDATE_NO_UPDATES:
Serial.println("HTTP_UPDATE_NO_UPDATES");
Serial.println("Your code is up to date!");
delay(10000); // Wait 10secs
break;
case HTTP_UPDATE_OK:
Serial.println("HTTP_UPDATE_OK");
delay(1000); // Wait a second and restart
ESP.restart();
break;
}
}
}
I retrieve the version of the filesystem from version.txt
file and put It on FILESYSTEM_VERSION
variable.
Serial.print(F("Inizializing FS..."));
if (LittleFS.begin()){
Serial.println(F("done."));
}else{
Serial.println(F("fail."));
}
Serial.print(F("FileSystem version "));
File versionFile = LittleFS.open(F("/version.txt"), "r");
if (versionFile) {
FILESYSTEM_VERSION = versionFile.readString();
versionFile.close();
}
Serial.println(FILESYSTEM_VERSION);
Create the endpoint on the server
Now we are going to create a new endpoint /updateFS
in GET that checks the filesystem version retrieved from version.txt
with the variable LAST_FS_VERSION
.
const express = require('express');
const { networkInterfaces } = require('os');
const path = require('path');
const app = express();
const nets = networkInterfaces();
// Server port
const PORT = 3000;
app.get('/', (request, response) => response.send('Hello from www.mischianti.org!'));
let downloadCounter = 1;
const LAST_VERSION = 0.3;
app.get('/update', (request, response) => {
const version = (request.header("x-esp8266-version"))?parseFloat(request.header("x-esp8266-version")):Infinity;
if (version<LAST_VERSION){
// If you need an update go here
response.status(200).download(path.join(__dirname, 'firmware/httpUpdateNew.bin'), 'httpUpdateNew.bin', (err)=>{
if (err) {
console.error("Problem on download firmware: ", err)
}else{
downloadCounter++;
}
});
console.log('Your file has been downloaded '+downloadCounter+' times!')
}else{
response.status(304).send('No update needed!')
}
});
let downloadFSCounter = 1;
const LAST_FS_VERSION = 0.3;
app.get('/updateFS', (request, response) => {
const version = (request.header("x-esp8266-version"))?parseFloat(request.header("x-esp8266-version")):Infinity;
if (version<LAST_FS_VERSION){
// If you need an update go here
response.status(200).download(path.join(__dirname, 'filesystem/httpUpdateNewFS.bin'), 'httpUpdateNewFS.bin', (err)=>{
if (err) {
console.error("Problem on download filesystem: ", err)
}else{
downloadFSCounter++;
}
});
console.log('Your file FS has been downloaded '+downloadFSCounter+' times!')
}else{
response.status(304).send('No FS update needed!')
}
});
app.listen(PORT, () => {
const results = {}; // Or just '{}', an empty object
for (const name of Object.keys(nets)) {
for (const net of nets[name]) {
// Skip over non-IPv4 and internal (i.e. 127.0.0.1) addresses
if (net.family === 'IPv4' && !net.internal) {
if (!results[name]) {
results[name] = [];
}
results[name].push(net.address);
}
}
}
console.log('Listening on port '+PORT+'\n', results)
});
Now we are going to modify the file version.txt
with version 0.2 then upload to the device
- In OTAServer create the folder filesystem,
- Change version to
0.3
inversion.txt
, regenerate without upload, and copy the fileArduinoOTAesp8266_fs_update.mklittlefs.bin
to the folder - Rename in
httpUpdateNewFS.bin
.
The result in serial output becomes like so.
[SETUP] WAIT 4...
[SETUP] WAIT 3...
[SETUP] WAIT 2...
[SETUP] WAIT 1...
Firmware version 0.2
Inizializing FS...done.
FileSystem version 0.2
Update start now!
CALLBACK: HTTP update process started
CALLBACK: HTTP update process at 0 of 2072576 bytes...
CALLBACK: HTTP update process at 0 of 2072576 bytes...
CALLBACK: HTTP update process at 4096 of 2072576 bytes...
CALLBACK: HTTP update process at 8192 of 2072576 bytes...
CALLBACK: HTTP update process at 12288 of 2072576 bytes...
CALLBACK: HTTP update process at 16384 of 2072576 bytes...
CALLBACK: HTTP update process at 20480 of 2072576 bytes...
CALLBACK: HTTP update process at 24576 of 2072576 bytes...
[...]
CALLBACK: HTTP update process at 2031616 of 2072576 bytes...
CALLBACK: HTTP update process at 2035712 of 2072576 bytes...
CALLBACK: HTTP update process at 2039808 of 2072576 bytes...
CALLBACK: HTTP update process at 2043904 of 2072576 bytes...
CALLBACK: HTTP update process at 2048000 of 2072576 bytes...
CALLBACK: HTTP update process at 2052096 of 2072576 bytes...
CALLBACK: HTTP update process at 2056192 of 2072576 bytes...
CALLBACK: HTTP update process at 2060288 of 2072576 bytes...
CALLBACK: HTTP update process at 2064384 of 2072576 bytes...
CALLBACK: HTTP update process at 2068480 of 2072576 bytes...
CALLBACK: HTTP update process at 2072576 of 2072576 bytes...
CALLBACK: HTTP update process at 2072576 of 2072576 bytes...
CALLBACK: HTTP update process at 2072576 of 2072576 bytes...
CALLBACK: HTTP update process finished
HTTP_UPDATE_OK
ets Jan 8 2013,rst cause:2, boot mode:(3,6)
load 0x4010f000, len 3460, room 16
tail 4
chksum 0xcc
load 0x3fff20b8, len 40, room 4
tail 4
chksum 0xc9
csum 0xc9
v000650f0
~ld
[SETUP] WAIT 4...
[SETUP] WAIT 3...
[SETUP] WAIT 2...
[SETUP] WAIT 1...
Firmware version 0.2
Inizializing FS...done.
FileSystem version 0.3
Update start now!
HTTP_UPDATE_NO_UPDATES
Your code is up to date!
Thanks
- Firmware management
- OTA update with Arduino IDE
- OTA update with Web Browser
- Self OTA uptate from HTTP server
- Non standard Firmware update
Hello Renzo.
Can I update firmware from a folder on an internet website, instead of using a local server ?
In case I can, I just upload it ?
Yo did an excellent work !
Best regards, Gabriel
Hi Gabriel,
you can use an internet website, but you must use a protocol to transfer the file like HTTP, or better HTTPS.
You must create a server (simple web server like NGINX) or you can use a hosting that offers apache or similar.
Bye Renzo