Send SMS using the email service
Guest post created with ♥ by Arthur 🙂|
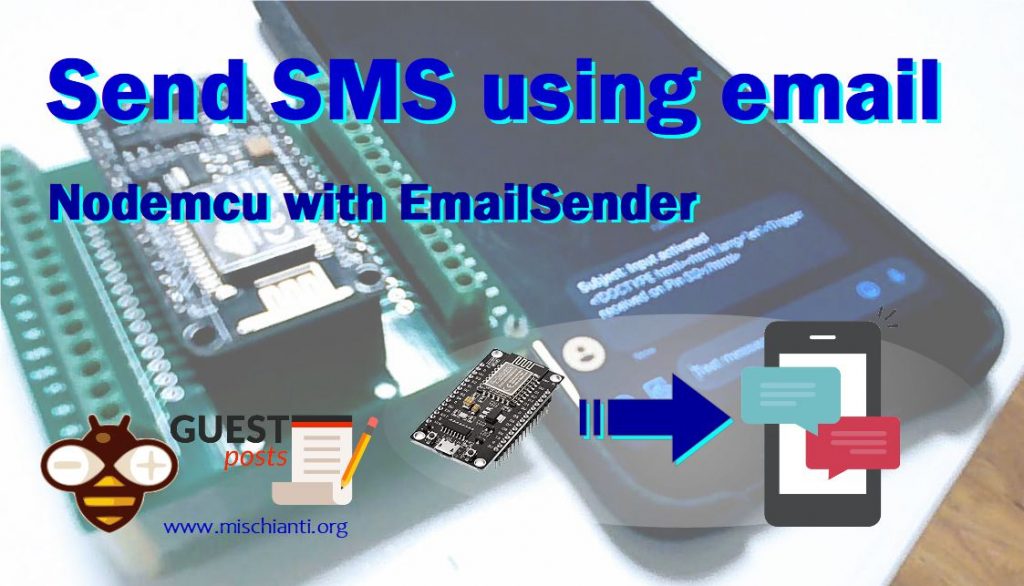
Introduction
In this guide I will explain how to also use the EMailSender library to send messages to phone numbers as well as email addresses.
This was tested on an ESP8266 on Board version 2.7.4, using the EMailSender library version 2.1.5.
You can find the board on Amazon
Here is the current setup for my ESP8266 listed in Arduino IDE:
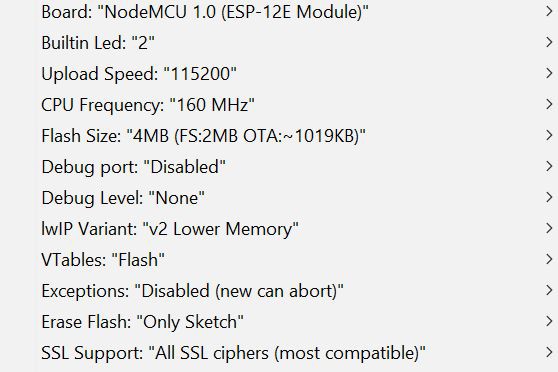
You can download the EMailSender library through the Arduino IDE by going through the library manager and then searching for “EMailSender” which will come up with this result:

Or you can go to the github page for this library, and then follow these steps.
- Click the green “Code” button.
- Download ZIP.
- Extract the folder and rename it to “EMailSender”, and place it inside the
Arduino/libraries folder. - Double check in “library manager” that “EMailSender” is installed.
Explanation of numbers
This library works sending emails to phone numbers because phone numbers essentially act as an email service.
Example: A number that is using the Google Fi network that is (123) 456-7890 would be written in the email format as:
I haven’t tested whether you need the +1 country code, but on this website or this website, you can see all the various cell phone providers and the format for the email, it does say that it only takes a 10 digit phone number for the “number” part of the email address. So the country code before the area code will not work in this scenario.
Code
const char* arrayOfEmail[] = {"emailaddress@gmail.com","1234567890@msg.fi.google.com"};
EmailSender emailSend("testemail@gmail.com","password123");
Here is an example code to send emails to two addresses, one to an gmail address and another to your phone number using the Google Fi network.
The next line is the gmail account that is used to send the emails, and the password associated with that account.
void sendEmail()
{
EmailSender::EmailMessage message;
message.subject = "Input activated";
message.message = "Trigger recevied on Pin D2";
message.mime = MIME_TEXT_PLAIN;
EmailSender::Response resp = emailSend.send(arrayOfEmail, 2, message);
Serial.println("Sending status: ");
Serial.println(resp.status);
Serial.println(resp.code);
Serial.println(resp.desc);
}
Here is the example function that can be used to send emails to those two addresses listed above.
You call the “arrayOfEmail” variable that contains the two addresses, the following number “2” says to just send an email to both addresses.
message.subject = "Input activated";
message.mime = MIME_TEXT_PLAIN;
message.message = "Trigger received on Pin D2";
When you send a text message using the default example, it sends HTML code in the text message as well, and does not look as clean. So therefore, you should add this line between message.subject and message.message. The message then comes out cleaner when sent, and does not include the HTML code that shows up if you do not include message.mime.
EmailSender::Response resp = emailSend.send(arrayOfEmail, 1, 1, message);
Putting an extra number after that first number indicates to send a regular email to the first address and a Carbon Copy (CC) email to the second address.
EmailSender::Response resp = emailSend.send(arrayOfEmail, 1, 1, 1, message);
If you had three email address or phone numbers to send a message to, you could use this example which shows three “1”s. The first place is sent as a regular email, a CC email to the second address, and a Blind Carbon Copy (BCC) to the last address. BCC is also written as CCn per Renzo’s tutorial.
// Connect to Wi-Fi
connection_state = WiFiConnect(ssid, password);
if(!connection_state) // if not connected to WiFi
Awaits(); // function that constantly tries to connect
It would be good practice to place any code that uses the WiFi or ethernet to connect to the ethernet near the end of the setup() part of the code, just so that you don’t get any errors.
void loop() {
if (pin2 = LOW) {
sendEmail();
}
// ... other loop code
}
Here is an example to send an email whenever pin2 is set to LOW (or 0V), it is placed in the loop function so it will send an email to those addresses we used earlier whenever the pin is triggered.
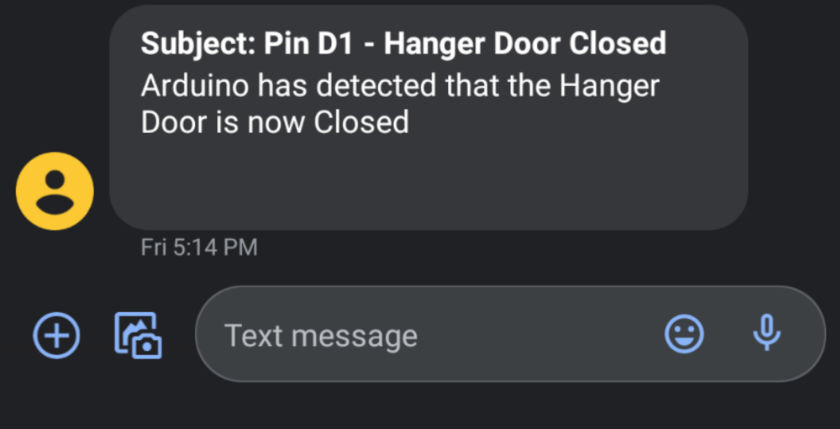
Pin2 in this example is set to receive an input of some sort, which could be anything: a button, a magnetic contact, anything that can trigger a state change of the pin to LOW (0V).
Conclusion
That wraps up the tutorial for sending emails to a phone number. This can be extremely useful in cases when you don’t have an email address you want to be watching and instead want to watch your text messaging app of your choice when the trigger is activated.
Note: When the email is sent to a phone number as well as another phone number or email address, it sets it up as a group conversation (MMS), so if your phone provider does not have this feature, it might end up in errors or you just won’t see the email message.
Super cool!! Great information!
Hi sir- your article is very interesting to me.
My question to you is how to build up my sms number if my phone number in Israel
is 97252-7811756.
I thing that my wi fi provider is the PARTNER company but i am not sure.
I use the sparkun esp8266 thing board.
Hi Dan,
I don’t know, but I think you must check the provider how It works.
Bye Renzo
Your library is fantastic.
I was using the version available on Arduino IDE, but it is old and do not recognize RP2040.
Example for RP2040 with and without SSL should be nice.
I really enjoy the new version and I am analyzing the Gmail error “Could not connect to mail server” since I am not using SSL at this first moment.
Good work!
Hi Fernando,
you use w5500? Or wifi?
Bye Renzo
It says here that EmailSender does not name a type error? How to solve it?
Hi Marc,
you must give us some other information and a complete stack trace.
Use the forum in the EMailSender library section.
Bye Renzo