DynamoDB JavaScript SDK v2 v3: manage items – 4
In the last article we had saw how many type of request we can do, but now we are going to see the basic operation that we can do over the single data.
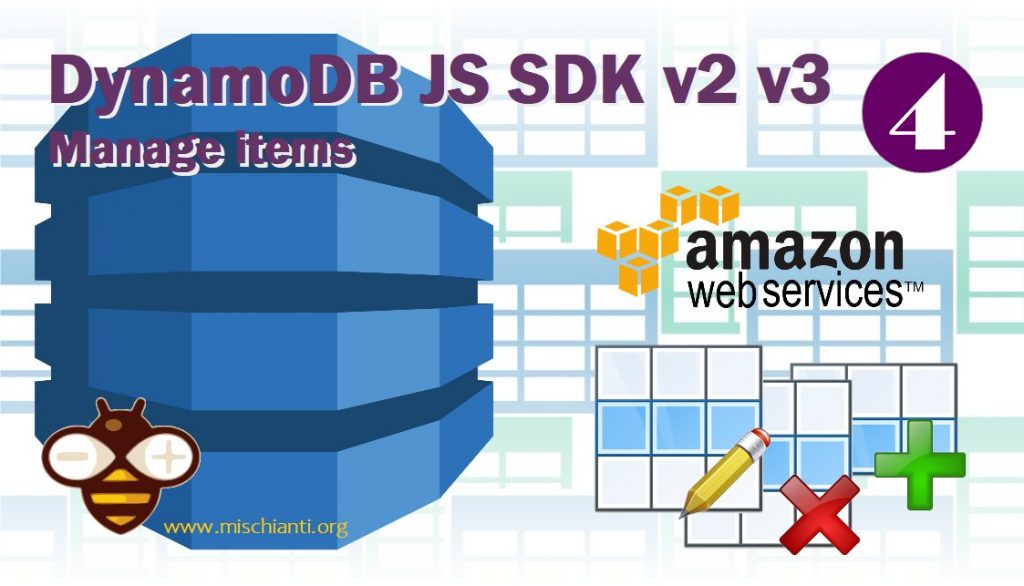
We also repeat the data addition methods seen in the last chapter.
Add an item
SDK v2
First we will see the asynchronous script, as in the previous parts.
To execute this script go in the folder dynamodb-examples\jsv2
and launch node item_add.js
/*
* DynamoDB Script Examples
* Add item with DynamoDB
* DB of selected region in AWS.config.update
*
* AUTHOR: Renzo Mischianti
*
* https://mischianti.org/
*
* The MIT License (MIT)
*
* Copyright (c) 2017 Renzo Mischianti www.mischianti.org All right reserved.
*
* You may copy, alter and reuse this code in any way you like, but please leave
* reference to www.mischianti.org in your comments if you redistribute this code.
*/
var AWS = require("aws-sdk");
AWS.config.update({
apiVersion: '2012-08-10',
region: "eu-west-1",
// endpoint: "http://localhost:8000",
// // accessKeyId default can be used while using the downloadable version of DynamoDB.
// // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead.
// accessKeyId: "9oiaf7",
// // secretAccessKey default can be used while using the downloadable version of DynamoDB.
// // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead.
// secretAccessKey: "yz5i9"
});
var ddb = new AWS.DynamoDB();
console.log("Start script!");
var params = {
TableName: "TestTableMischianti",
Item: {
"ItemId": {S: "mischianti"},
"ItemName": {S: "www.mischianti.org"},
"ValueStr": {S: "Mischianti main url"},
"ValueNum": {N: "1"},
}
};
ddb.putItem(params, function (err, data) {
if (err) {
console.error("Unable add item", JSON.stringify(params, null, 2), ". Error JSON:", JSON.stringify(err, null, 2));
} else {
console.log("PutItem succeeded:", JSON.stringify(params, null, 2), data);
}
});
console.log("End script!");
The result in console is
D:\Projects\AlexaProjects\dynamodb-management\dynamodb-examples\jsv2>node item_add.js
Start script!
End script!
PutItem succeeded: {
"TableName": "TestTableMischianti",
"Item": {
"ItemId": {
"S": "mischianti"
},
"ItemName": {
"S": "www.mischianti.org"
},
"ValueStr": {
"S": "Mischianti main url"
},
"ValueNum": {
"N": "1"
}
}
} {}
Check the parameter syntax
var params = {
TableName: "TestTableMischianti",
Item: {
"ItemId": {S: "mischianti"},
"ItemName": {S: "www.mischianti.org"},
"ValueStr": {S: "Mischianti main url"},
"ValueNum": {N: "1"},
}
};
we must specify the defined key (check the create table article), than you can add the values you need, the S
or N
value is the type of the data S --> String
and N --> Number
.
Now the asynchronous version (node item_add_async_await.js
).
/*
* DynamoDB Script Examples
* Add item with DynamoDB async await
* DB of selected region in AWS.config.update
*
* AUTHOR: Renzo Mischianti
*
* https://mischianti.org/
*
* The MIT License (MIT)
*
* Copyright (c) 2017 Renzo Mischianti www.mischianti.org All right reserved.
*
* You may copy, alter and reuse this code in any way you like, but please leave
* reference to www.mischianti.org in your comments if you redistribute this code.
*
*/
var AWS = require("aws-sdk");
AWS.config.update({
apiVersion: '2012-08-10',
region: "eu-west-1",
// endpoint: "http://localhost:8000",
// // accessKeyId default can be used while using the downloadable version of DynamoDB.
// // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead.
// accessKeyId: "9oiaf7",
// // secretAccessKey default can be used while using the downloadable version of DynamoDB.
// // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead.
// secretAccessKey: "yz5i9"
});
var ddb = new AWS.DynamoDB();
(async function () {
console.log("Start script!");
var params = {
TableName: "TestTableMischianti",
Item: {
"ItemId": {S: "mischianti"},
"ItemName": {S: "www.mischianti.org"},
"ValueStr": {S: "Mischianti main url"},
"ValueNum": {N: "1"},
}
};
try {
const data = await ddb.putItem(params).promise();
console.log("PutItem succeeded:", JSON.stringify(params, null, 2), data);
}catch (err) {
console.error("Unable add item", JSON.stringify(params, null, 2), ". Error JSON:", JSON.stringify(err, null, 2));
}
console.log("End script!");})();
As already described the difference was only the execution order.
SDK v3
In v3 version there are some change and Amazon advise to use async await (synchronous) with modularization of import. But we are going to restart from the last article syntax.
Now the asynchronous (discouraged mode) versione of the add script.
To execute this script go in the folder dynamodb-examples\jsv3
and launch node item_add.js
/*
* DynamoDB Script Examples v3
* Add item with DynamoDB
* DB of selected region in configDynamoDB
*
* AUTHOR: Renzo Mischianti
*
* https://mischianti.org/
*
* The MIT License (MIT)
*
* Copyright (c) 2017 Renzo Mischianti www.mischianti.org All right reserved.
*
* You may copy, alter and reuse this code in any way you like, but please leave
* reference to www.mischianti.org in your comments if you redistribute this code.
*
*/
const { DynamoDBClient, PutItemCommand } = require("@aws-sdk/client-dynamodb");
const configDynamoDB = {
version: 'latest',
region: "eu-west-1",
// endpoint: "http://localhost:8000",
// credentials: {
// // accessKeyId default can be used while using the downloadable version of DynamoDB.
// // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead.
// accessKeyId: "9oiaf7",
// // secretAccessKey default can be used while using the downloadable version of DynamoDB.
// // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead.
// secretAccessKey: "yz5i9"
//
// }
};
const dbClient = new DynamoDBClient(configDynamoDB);
const addElement = (params) => {
const command = new PutItemCommand(params);
return dbClient.send(command);
}
console.log("Start script!");
var params = {
TableName: "TestTableMischianti",
Item: {
"ItemId": {S: "mischianti"},
"ItemName": {S: "www.mischianti.org"},
"ValueStr": {S: "Mischianti main url"},
"ValueNum": {N: "1"},
}
};
const command = new PutItemCommand(params);
const data = dbClient.send(command)
.then(
data => console.log("PutItem succeeded:", JSON.stringify(params, null, 2), data)
).catch(
err => console.error("Unable add item", JSON.stringify(params, null, 2), ". Error JSON:", JSON.stringify(err, null, 2))
).finally(
() => console.log("END Of execution!")
);
console.log("End script!");
The console output become more verbose and we have additional information.
D:\Projects\AlexaProjects\dynamodb-management\dynamodb-examples\jsv3>node item_add.js
Start script!
End script!
PutItem succeeded: {
"TableName": "TestTableMischianti",
"Item": {
"ItemId": {
"S": "mischianti"
},
"ItemName": {
"S": "www.mischianti.org"
},
"ValueStr": {
"S": "Mischianti main url"
},
"ValueNum": {
"N": "1"
}
}
} { '$metadata':
{ httpStatusCode: 200,
httpHeaders:
{ server: 'Server',
date: 'Fri, 15 Jan 2021 21:38:39 GMT',
'content-type': 'application/x-amz-json-1.0',
'content-length': '2',
connection: 'keep-alive',
'x-amzn-requestid': '035UKDLIR2FGUQ66ISB3UG8K17VV4KQNSO5AEMVJF66Q9ASUAAJG',
'x-amz-crc32': '2745614147' },
requestId: '035UKDLIR2FGUQ66ISB3UG8K17VV4KQNSO5AEMVJF66Q9ASUAAJG',
attempts: 1,
totalRetryDelay: 0 },
Attributes: undefined,
ConsumedCapacity: undefined,
ItemCollectionMetrics: undefined }
END Of execution!
In detail we have l’http status code to identify the status of response (httpStatusCode: 200
), and for the developer it is now explicit that we work with REST calls.
Now the synchronous script (node item_add_async_await.js
)
/*
* DynamoDB Script Examples v3
* Add item with DynamoDB async await
* DB of selected region in configDynamoDB
*
* AUTHOR: Renzo Mischianti
*
* https://mischianti.org/
*
* The MIT License (MIT)
*
* Copyright (c) 2017 Renzo Mischianti www.mischianti.org All right reserved.
*
* You may copy, alter and reuse this code in any way you like, but please leave
* reference to www.mischianti.org in your comments if you redistribute this code.
*
*/
const { DynamoDBClient, PutItemCommand } = require("@aws-sdk/client-dynamodb");
const configDynamoDB = {
version: 'latest',
region: "eu-west-1",
// endpoint: "http://localhost:8000",
// credentials: {
// // accessKeyId default can be used while using the downloadable version of DynamoDB.
// // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead.
// accessKeyId: "9oiaf7",
// // secretAccessKey default can be used while using the downloadable version of DynamoDB.
// // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead.
// secretAccessKey: "yz5i9"
//
// }
};
const dbClient = new DynamoDBClient(configDynamoDB);
(async function () {
console.log("Start script!");
var params = {
TableName: "TestTableMischianti",
Item: {
"ItemId": {S: "mischianti"},
"ItemName": {S: "www.mischianti.org"},
"ValueStr": {S: "Mischianti main url"},
"ValueNum": {N: "1"},
}
};
try {
const command = new PutItemCommand(params);
const data = await dbClient.send(command);
console.log("PutItem succeeded:", JSON.stringify(params, null, 2), data);
} catch (err) {
console.error("Unable add item", JSON.stringify(params, null, 2), ". Error JSON:", JSON.stringify(err, null, 2));
}
console.log("End script!");
})();
Check the data
- You can find all the data in the DynamoDB section of the AWS console, go to this link (check the region).
https://eu-west-1.console.aws.amazon.com/dynamodb/home?region=eu-west-1#
- Click on Table/Tabelle;
- Select TestTableMischianti table;
- Click on element tab.
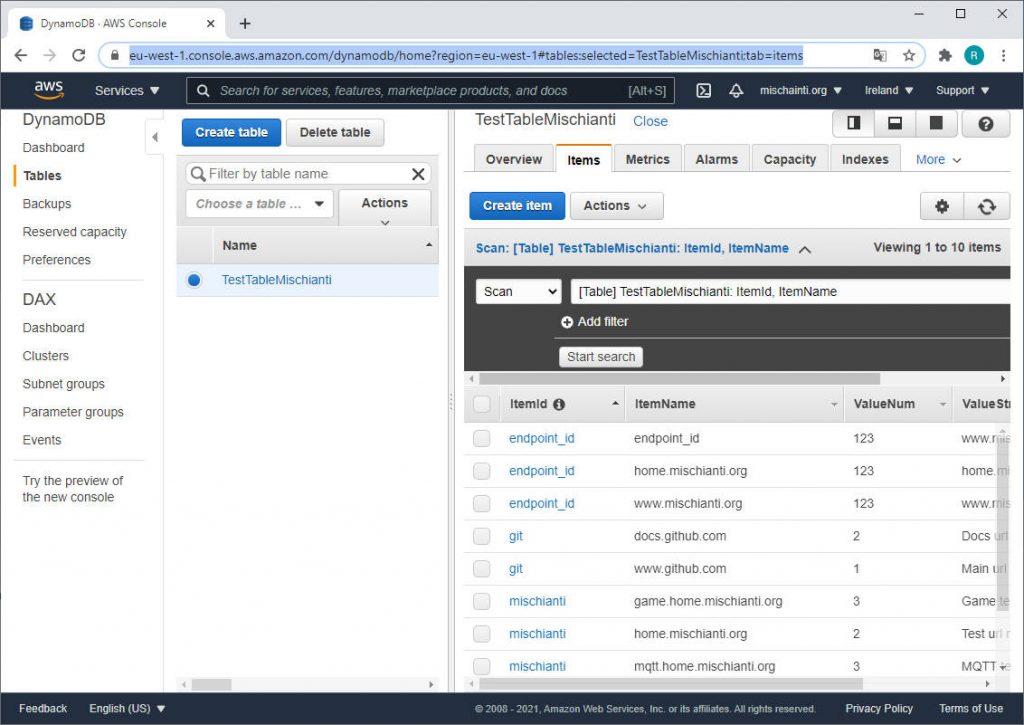
Delete items
The logic is the same for all command, so now we are going more fast.
SDK v2
Here the synchronous script (node item_delete.js
).
/*
* DynamoDB Script Examples
* Delete item with DynamoDB
* DB of selected region in AWS.config.update
*
* AUTHOR: Renzo Mischianti
*
* https://mischianti.org/
*
* The MIT License (MIT)
*
* Copyright (c) 2017 Renzo Mischianti www.mischianti.org All right reserved.
*
* You may copy, alter and reuse this code in any way you like, but please leave
* reference to www.mischianti.org in your comments if you redistribute this code.
*
*/
var AWS = require("aws-sdk");
AWS.config.update({
apiVersion: '2012-08-10',
region: "eu-west-1",
// endpoint: "http://localhost:8000",
// // accessKeyId default can be used while using the downloadable version of DynamoDB.
// // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead.
// accessKeyId: "9oiaf7",
// // secretAccessKey default can be used while using the downloadable version of DynamoDB.
// // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead.
// secretAccessKey: "yz5i9"
});
var ddb = new AWS.DynamoDB();
console.log("Start script!");
var params = {
TableName: "TestTableMischianti",
Key: {
"ItemId": {S: "mischianti"},
"ItemName": {S: "www.mischianti.org"}
}
};
ddb.deleteItem(params, function (err, data) {
if (err) {
console.error("Unable delete item", JSON.stringify(params, null, 2), ". Error JSON:", JSON.stringify(err, null, 2));
} else {
console.log("DeleteItem succeeded:", JSON.stringify(params, null, 2), data);
}
});
console.log("End script!")
You can see that in the params we must specify all the declared key, you can’t omit one key.
var params = {
TableName: "TestTableMischianti",
Key: {
"ItemId": {S: "mischianti"},
"ItemName": {S: "www.mischianti.org"}
}
};
and here the console output.
D:\Projects\AlexaProjects\dynamodb-management\dynamodb-examples\jsv2>node item_delete.js
Start script!
End script!
DeleteItem succeeded: {
"TableName": "TestTableMischianti",
"Key": {
"ItemId": {
"S": "mischianti"
},
"ItemName": {
"S": "www.mischianti.org"
}
}
} {}
No result is exposed.
And now the asynchronous script (node item_delete_async_await.js
).
/*
* DynamoDB Script Examples
* Delete item with DynamoDB
* DB of selected region in AWS.config.update
*
* AUTHOR: Renzo Mischianti
*
* https://mischianti.org/
*
* The MIT License (MIT)
*
* Copyright (c) 2017 Renzo Mischianti www.mischianti.org All right reserved.
*
* You may copy, alter and reuse this code in any way you like, but please leave
* reference to www.mischianti.org in your comments if you redistribute this code.
*/
var AWS = require("aws-sdk");
AWS.config.update({
apiVersion: '2012-08-10',
region: "eu-west-1",
// endpoint: "http://localhost:8000",
// // accessKeyId default can be used while using the downloadable version of DynamoDB.
// // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead.
// accessKeyId: "9oiaf7",
// // secretAccessKey default can be used while using the downloadable version of DynamoDB.
// // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead.
// secretAccessKey: "yz5i9"
});
var ddb = new AWS.DynamoDB();
(async function () {
console.log("Start script!");
var params = {
TableName: "TestTableMischianti",
Key: {
"ItemId": {S: "mischianti"},
"ItemName": {S: "www.mischianti.org"}
}
};
try {
const data = await ddb.deleteItem(params).promise();
console.log("DeleteItem succeeded:", JSON.stringify(params, null, 2), data);
}catch (err) {
console.error("Unable delete item", JSON.stringify(params, null, 2), ". Error JSON:", JSON.stringify(err, null, 2));
}
console.log("End script!");
})();
The output in console change only for the order of log.
SDK v3
To execute this script go in the folder dynamodb-examples\jsv3
and launch node item_delete_async_await.js
/*
* DynamoDB Script Examples
* Delete item with DynamoDB
* DB of selected region in AWS.config.update
*
* AUTHOR: Renzo Mischianti
*
* https://mischianti.org/
*
* The MIT License (MIT)
*
* Copyright (c) 2017 Renzo Mischianti www.mischianti.org All right reserved.
*
* You may copy, alter and reuse this code in any way you like, but please leave
* reference to www.mischianti.org in your comments if you redistribute this code.
*/
var AWS = require("aws-sdk");
AWS.config.update({
apiVersion: '2012-08-10',
region: "eu-west-1",
// endpoint: "http://localhost:8000",
// // accessKeyId default can be used while using the downloadable version of DynamoDB.
// // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead.
// accessKeyId: "9oiaf7",
// // secretAccessKey default can be used while using the downloadable version of DynamoDB.
// // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead.
// secretAccessKey: "yz5i9"
});
var ddb = new AWS.DynamoDB();
(async function () {
console.log("Start script!");
var params = {
TableName: "TestTableMischianti",
Key: {
"ItemId": {S: "mischianti"},
"ItemName": {S: "www.mischianti.org"}
}
};
try {
const data = await ddb.deleteItem(params).promise();
console.log("DeleteItem succeeded:", JSON.stringify(params, null, 2), data);
}catch (err) {
console.error("Unable delete item", JSON.stringify(params, null, 2), ". Error JSON:", JSON.stringify(err, null, 2));
}
console.log("End script!");
})();
with this console output
D:\Projects\AlexaProjects\dynamodb-management\dynamodb-examples\jsv3>node item_delete_async_await.js
Start script!
DeleteItem succeeded: {
"TableName": "TestTableMischianti",
"Key": {
"ItemId": {
"S": "mischianti"
},
"ItemName": {
"S": "www.mischianti.org"
}
}
} { '$metadata':
{ httpStatusCode: 200,
httpHeaders:
{ server: 'Server',
date: 'Wed, 27 Jan 2021 08:01:21 GMT',
'content-type': 'application/x-amz-json-1.0',
'content-length': '2',
connection: 'keep-alive',
'x-amzn-requestid': 'GGMGF3FS2QKQHPR35SGABV9VEJVV4KQNSO5AEMVJF66Q9ASUAAJG',
'x-amz-crc32': '2745614147' },
requestId: 'GGMGF3FS2QKQHPR35SGABV9VEJVV4KQNSO5AEMVJF66Q9ASUAAJG',
attempts: 1,
totalRetryDelay: 0 },
Attributes: undefined,
ConsumedCapacity: undefined,
ItemCollectionMetrics: undefined }
End script!
Get Items
It’s time to retrieve the item we had inserted, you can get that information by filtering for all the declared key.
SDK v2
To execute this script go in the folder dynamodb-examples\jsv2
and launch node item_get.js
/*
* DynamoDB Script Examples
* Get item with DynamoDB
* DB of selected region in AWS.config.update
*
* AUTHOR: Renzo Mischianti
*
* https://mischianti.org/
*
* The MIT License (MIT)
*
* Copyright (c) 2017 Renzo Mischianti www.mischianti.org All right reserved.
*
* You may copy, alter and reuse this code in any way you like, but please leave
* reference to www.mischianti.org in your comments if you redistribute this code.
*/
var AWS = require("aws-sdk");
AWS.config.update({
apiVersion: '2012-08-10',
region: "eu-west-1",
// endpoint: "http://localhost:8000",
// // accessKeyId default can be used while using the downloadable version of DynamoDB.
// // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead.
// accessKeyId: "9oiaf7",
// // secretAccessKey default can be used while using the downloadable version of DynamoDB.
// // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead.
// secretAccessKey: "yz5i9"
});
var ddb = new AWS.DynamoDB();
console.log("Start script!");
var params = {
TableName: "TestTableMischianti",
Key: {
"ItemId": {S: "mischianti"},
"ItemName": {S: "www.mischianti.org"},
}
};
ddb.getItem(params, function (err, data) {
if (err) {
console.error("Unable ti get item", JSON.stringify(params, null, 2), ". Error JSON:", JSON.stringify(err, null, 2));
} else {
console.log("Get Item succeeded:", JSON.stringify(data, null, 2));
}
});
console.log("End script!");
It’s quite simple, you only must specify the table and the key in the params
var params = {
TableName: "TestTableMischianti",
Key: {
"ItemId": {S: "mischianti"},
"ItemName": {S: "www.mischianti.org"},
}
};
at the same way you can use a synchronous script item_get_async_await.js
:
/*
* DynamoDB Script Examples
* Get item with DynamoDB async await
* DB of selected region in AWS.config.update
*
* AUTHOR: Renzo Mischianti
*
* https://mischianti.org/
*
* The MIT License (MIT)
*
* Copyright (c) 2017 Renzo Mischianti www.mischianti.org All right reserved.
*
* You may copy, alter and reuse this code in any way you like, but please leave
* reference to www.mischianti.org in your comments if you redistribute this code.
*
*/
var AWS = require("aws-sdk");
AWS.config.update({
apiVersion: '2012-08-10',
region: "eu-west-1",
// endpoint: "http://localhost:8000",
// // accessKeyId default can be used while using the downloadable version of DynamoDB.
// // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead.
// accessKeyId: "9oiaf7",
// // secretAccessKey default can be used while using the downloadable version of DynamoDB.
// // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead.
// secretAccessKey: "yz5i9"
});
var ddb = new AWS.DynamoDB();
(async function () {
console.log("Start script!");
var params = {
TableName: "TestTableMischianti",
Key: {
"ItemId": {S: "mischianti"},
"ItemName": {S: "www.mischianti.org"},
}
};
try {
const data = await ddb.getItem(params).promise();
console.log("Get Item succeeded:", JSON.stringify(data, null, 2));
}catch (err) {
console.error("Unable ti get item", JSON.stringify(params, null, 2), ". Error JSON:", JSON.stringify(err, null, 2));
}
console.log("End script!");
})();
The output in console is
D:\Projects\AlexaProjects\dynamodb-management\dynamodb-examples\jsv2>node item_get_async_await.js
Start script!
Get Item succeeded: {
"Item": {
"ValueStr": {
"S": "Mischianti main url"
},
"ItemName": {
"S": "www.mischianti.org"
},
"ItemId": {
"S": "mischianti"
},
"ValueNum": {
"N": "1"
}
}
}
End script!
You can find the data under Item
key.
SDK v3
To execute this script go in the folder dynamodb-examples\jsv3
and launch node item_get.js
/*
* DynamoDB Script Examples v3
* Get item with DynamoDB async await
* DB of selected region in configDynamoDB
*
* AUTHOR: Renzo Mischianti
*
* https://mischianti.org/
*
* The MIT License (MIT)
*
* Copyright (c) 2017 Renzo Mischianti www.mischianti.org All right reserved.
*
* You may copy, alter and reuse this code in any way you like, but please leave
* reference to www.mischianti.org in your comments if you redistribute this code.
*
*/
const { DynamoDBClient, GetItemCommand } = require("@aws-sdk/client-dynamodb");
const configDynamoDB = {
version: 'latest',
region: "eu-west-1",
// endpoint: "http://localhost:8000",
// credentials: {
// // accessKeyId default can be used while using the downloadable version of DynamoDB.
// // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead.
// accessKeyId: "9oiaf7",
// // secretAccessKey default can be used while using the downloadable version of DynamoDB.
// // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead.
// secretAccessKey: "yz5i9"
//
// }
};
const dbClient = new DynamoDBClient(configDynamoDB);
(async function () {
console.log("Start script!");
var params = {
TableName: "TestTableMischianti",
Key: {
"ItemId": {S: "mischianti"},
"ItemName": {S: "www.mischianti.org"}
}
};
try {
const command = new GetItemCommand(params);
const data = await dbClient.send(command);
console.log("GetItem succeeded:", JSON.stringify(params, null, 2), JSON.stringify(data, null, 2));
}catch (err) {
console.error("Unable get item", JSON.stringify(params, null, 2), ". Error JSON:", JSON.stringify(err, null, 2));
}
console.log("End script!");
})();
The console output is
D:\Projects\AlexaProjects\dynamodb-management\dynamodb-examples\jsv3>node item_get_async_await.js
Start script!
GetItem succeeded: {
"TableName": "TestTableMischianti",
"Key": {
"ItemId": {
"S": "mischianti"
},
"ItemName": {
"S": "www.mischianti.org"
}
}
} {
"$metadata": {
"httpStatusCode": 200,
"httpHeaders": {
"server": "Server",
"date": "Wed, 27 Jan 2021 08:33:59 GMT",
"content-type": "application/x-amz-json-1.0",
"content-length": "136",
"connection": "keep-alive",
"x-amzn-requestid": "26ORUJ7O63QIV916K5S3D0OLLBVV4KQNSO5AEMVJF66Q9ASUAAJG",
"x-amz-crc32": "1542980662"
},
"requestId": "26ORUJ7O63QIV916K5S3D0OLLBVV4KQNSO5AEMVJF66Q9ASUAAJG",
"attempts": 1,
"totalRetryDelay": 0
},
"Item": {
"ValueStr": {
"S": "Mischianti main url"
},
"ItemName": {
"S": "www.mischianti.org"
},
"ItemId": {
"S": "mischianti"
},
"ValueNum": {
"N": "1"
}
}
}
End script!
The data is still under the Item key.
Thanks
- DynamoDB JavaScript SDK v2 v3: prerequisite and SDK v2 v3 introduction
- DynamoDB JavaScript SDK v2 v3: manage tables
- DynamoDB JavaScript SDK v2 v3: add items with DB or DocumentClient
- DynamoDB JavaScript SDK v2 v3: manage items
- DynamoDB JavaScript SDK v2 v3: scan table data and pagination
- DynamoDB JavaScript SDK v2 v3: query