Arduino SAMD NINA: WiFiNINA, firmware update and RGB led – 2
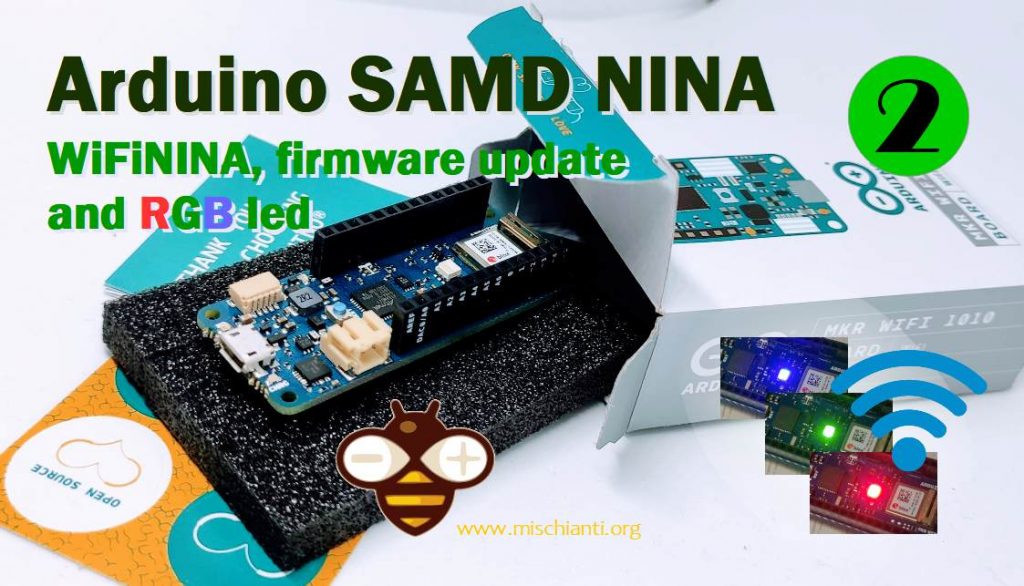
Import and use of WiFiNINA
This library allows you to use the Arduino UNO WiFi Rev.2, Arduino NANO 33 IoT, Arduino MKR 1010, and Arduino MKR VIDOR 4000 WiFi capabilities. It can serve as either a server accepting incoming connections or a client making outgoing ones. The library supports WEP, WPA2 Personal, and WPA2 Enterprise encryptions. This library support all the same methods as the original WiFi library plus the connectSSL()
. The WiFiNINA library is very similar to the Ethernet and the library WiFi, and many of the function calls are the same.
Download
You can simply download WiFiNINA lib from the libraries repository.
Now you have a new menu item also, WiFi101/ WiFiNINA firmware updater
.
Check firmware
It’s essential that the WiFiNINA version and NINA-W10 chip have the correct version; you can check that with an example sketch that you can find in File --> Examples --> WiFiNINA --> Tools --> CheckFirmwareVersion
open and upload It.
/*
* This example check if the firmware loaded on the NINA module
* is updated.
*
* Circuit:
* - Board with NINA module (Arduino MKR WiFi 1010, MKR VIDOR 4000 and UNO WiFi Rev.2)
*
* Created 17 October 2018 by Riccardo Rosario Rizzo
* This code is in the public domain.
*/
#include <SPI.h>
#include <WiFiNINA.h>
void setup() {
// Initialize serial
Serial.begin(9600);
while (!Serial) {
; // wait for serial port to connect. Needed for native USB port only
}
// Print a welcome message
Serial.println("WiFiNINA firmware check.");
Serial.println();
// check for the WiFi module:
if (WiFi.status() == WL_NO_MODULE) {
Serial.println("Communication with WiFi module failed!");
// don't continue
while (true);
}
// Print firmware version on the module
String fv = WiFi.firmwareVersion();
String latestFv;
Serial.print("Firmware version installed: ");
Serial.println(fv);
latestFv = WIFI_FIRMWARE_LATEST_VERSION;
// Print required firmware version
Serial.print("Latest firmware version available : ");
Serial.println(latestFv);
// Check if the latest version is installed
Serial.println();
if (fv >= latestFv) {
Serial.println("Check result: PASSED");
} else {
Serial.println("Check result: NOT PASSED");
Serial.println(" - The firmware version on the module do not match the");
Serial.println(" version required by the library, you may experience");
Serial.println(" issues or failures.");
}
}
void loop() {
// do nothing
}
If you have the wrong firmware version, you get this message.
WiFiNINA firmware check.
Firmware version installed: 1.3.0
Latest firmware version available : 1.4.2
Check result: NOT PASSED
- The firmware version on the module do not match the
version required by the library, you may experience
issues or failures.
Update firmware
If you have the wrong firmware version, you must upload the last.
To do that, you must get another example sketch from File --> Examples --> WiFiNINA --> Tools --> FirmwareUpdater
/*
FirmwareUpdater - Firmware Updater for the
Arduino MKR WiFi 1010, Arduino MKR Vidor 4000, and Arduino UNO WiFi Rev.2.
Copyright (c) 2018 Arduino SA. All rights reserved.
This library is free software; you can redistribute it and/or
modify it under the terms of the GNU Lesser General Public
License as published by the Free Software Foundation; either
version 2.1 of the License, or (at your option) any later version.
This library is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
Lesser General Public License for more details.
You should have received a copy of the GNU Lesser General Public
License along with this library; if not, write to the Free Software
Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA
*/
#include "ESP32BootROM.h"
typedef struct __attribute__((__packed__)) {
uint8_t command;
uint32_t address;
uint32_t arg1;
uint16_t payloadLength;
// payloadLenght bytes of data follows...
} UartPacket;
static const int MAX_PAYLOAD_SIZE = 1024;
#define CMD_READ_FLASH 0x01
#define CMD_WRITE_FLASH 0x02
#define CMD_ERASE_FLASH 0x03
#define CMD_MD5_FLASH 0x04
#define CMD_MAX_PAYLOAD_SIZE 0x50
#define CMD_HELLO 0x99
void setup() {
Serial.begin(1000000);
if (!ESP32BootROM.begin(921600)) {
Serial.println("Unable to communicate with ESP32 boot ROM!");
while (1);
}
}
void receivePacket(UartPacket *pkt, uint8_t *payload) {
// Read command
uint8_t *p = reinterpret_cast<uint8_t *>(pkt);
uint16_t l = sizeof(UartPacket);
while (l > 0) {
int c = Serial.read();
if (c == -1)
continue;
*p++ = c;
l--;
}
// Convert parameters from network byte order to cpu byte order
pkt->address = fromNetwork32(pkt->address);
pkt->arg1 = fromNetwork32(pkt->arg1);
pkt->payloadLength = fromNetwork16(pkt->payloadLength);
// Read payload
l = pkt->payloadLength;
while (l > 0) {
int c = Serial.read();
if (c == -1)
continue;
*payload++ = c;
l--;
}
}
// Allocated statically so the compiler can tell us
// about the amount of used RAM
static UartPacket pkt;
static uint8_t payload[MAX_PAYLOAD_SIZE];
void loop() {
receivePacket(&pkt, payload);
if (pkt.command == CMD_HELLO) {
if (pkt.address == 0x11223344 && pkt.arg1 == 0x55667788)
Serial.print("v10000");
}
if (pkt.command == CMD_MAX_PAYLOAD_SIZE) {
uint16_t res = toNetwork16(MAX_PAYLOAD_SIZE);
Serial.write(reinterpret_cast<uint8_t *>(&res), sizeof(res));
}
if (pkt.command == CMD_READ_FLASH) {
// not supported!
Serial.println("ER");
}
if (pkt.command == CMD_WRITE_FLASH) {
uint32_t len = pkt.payloadLength;
if (!ESP32BootROM.dataFlash(payload, len)) {
Serial.print("ER");
} else {
Serial.print("OK");
}
}
if (pkt.command == CMD_ERASE_FLASH) {
uint32_t address = pkt.address;
uint32_t len = pkt.arg1;
if (!ESP32BootROM.beginFlash(address, len, MAX_PAYLOAD_SIZE)) {
Serial.print("ER");
} else {
Serial.print("OK");
}
}
if (pkt.command == CMD_MD5_FLASH) {
uint32_t address = pkt.address;
uint32_t len = pkt.arg1;
if (!ESP32BootROM.endFlash(1)) {
Serial.print("ER");
} else {
ESP32BootROM.end();
uint8_t md5[16];
if (!ESP32BootROM.begin(921600)) {
Serial.print("ER");
} else if (!ESP32BootROM.md5Flash(address, len, md5)) {
Serial.print("ER");
} else {
Serial.print("OK");
Serial.write(md5, sizeof(md5));
}
}
}
}
after getting the sketch you must upload.
Now you can click on WiFi101/ WiFiNINA firmware updater
.
Select the correct device port, try Test connection; if all is ok, select the last firmware version and click on Update Firmware.
As you can see, you can also add a certified domain to the NINA-W101, so they become trusted.
Simple WiFi connection with WiFiNINA
Here is a simple sketch to connect your device with WiFiNINA.
/*
* WiFiNINA usage
* Connect to WiFi
*
* www.mischianti.org
*/
#include <WiFiNINA.h>
const char* ssid = "SSID"; // The SSID (name) of the Wi-Fi network you want to connect to
const char* password = "PASSWORD"; // The password of the Wi-Fi network
void setup() {
Serial.begin(115200); // Start the Serial communication to send messages to the computer
delay(10);
Serial.println('\n');
WiFi.begin(ssid, password); // Connect to the network
Serial.print("Connecting to ");
Serial.print(ssid); Serial.print(" .");
while (WiFi.status() != WL_CONNECTED) { // Wait for the Wi-Fi to connect
delay(1000);
Serial.print('.');
}
Serial.println('\n');
Serial.println("Connection established!");
Serial.print("IP address:\t");
Serial.println(WiFi.localIP()); // Send the IP address of the ESP8266 to the computer
}
void loop() { }
Manage RGB Led with WiFiNINA
WiFiNINA library also contains the code to manage the RGB LED present on the MKR device, and It uses the SPI protocol of the IC to send the value to the three pins Red, Green, and Blue.
In the library, the management of the pin is wrapped with a digitalWrite clone command and an analogWrite clone.
Here is a sketch with digitalWrite
that change the color
/*
* Here a simple sketch to test the use of RGB LED.
* Change the leds color.
* by Mischianti Renzo <https://mischianti.org>
*
* https://mischianti.org/
*
*/
#include <WiFiNINA.h>
#include <utility/wifi_drv.h>
#define RGB_LED_GREEN 25
#define RGB_LED_RED 26
#define RGB_LED_BLUE 27
void setup()
{
WiFiDrv::pinMode(RGB_LED_GREEN, OUTPUT); //GREEN
WiFiDrv::pinMode(RGB_LED_RED, OUTPUT); //RED
WiFiDrv::pinMode(RGB_LED_BLUE, OUTPUT); //BLUE
}
uint8_t currentLedCounter = 0;
uint8_t leds[] = {RGB_LED_GREEN, RGB_LED_RED, RGB_LED_BLUE};
void loop()
{
WiFiDrv::digitalWrite(leds[currentLedCounter], LOW);
currentLedCounter++;
if (currentLedCounter>2){
currentLedCounter = 0;
}
WiFiDrv::digitalWrite(leds[currentLedCounter], HIGH);
delay(1000);
}
Here is a fade color change with analogWrite
/*
* Here a simple sketch to test the use of RGB LED.
* Fade the leds color.
* by Mischianti Renzo <https://mischianti.org>
*
* https://mischianti.org/
*
*/
#include <WiFiNINA.h>
#include <utility/wifi_drv.h>
#define RGB_LED_GREEN 25
#define RGB_LED_RED 26
#define RGB_LED_BLUE 27
void setup()
{
WiFiDrv::pinMode(RGB_LED_GREEN, OUTPUT); //GREEN
WiFiDrv::pinMode(RGB_LED_RED, OUTPUT); //RED
WiFiDrv::pinMode(RGB_LED_BLUE, OUTPUT); //BLUE
}
uint8_t counter = 0;
uint8_t currentLedCounter = 0;
uint8_t leds[] = {RGB_LED_GREEN, RGB_LED_RED, RGB_LED_BLUE};
int8_t progress = 1;
void loop()
{
if (counter<1) {
progress = 1;
currentLedCounter++;
if (currentLedCounter>2){
currentLedCounter = 0;
}
}
if (counter>254) {
progress = -1;
}
WiFiDrv::analogWrite(leds[currentLedCounter], counter);
counter = counter + progress;
delay(5);
}
Here the result
Thanks
- Arduino SAMD NINA: pinout, specs and Arduino IDE configuration
- Arduino SAMD NINA: WiFiNINA, firmware update, and RGB led
- Arduino SAMD (NANO 33 and MKR): SPI flash memory FAT FS
- i2c Arduino SAMD MKR: additional interface SERCOM, network and address scanner
- Arduino MKR SAMD: FAT filesystem on external SPI flash memory
- Connecting the EByte E70 to Arduino SAMD (Nano 33, MKR…) devices and a simple sketch example