Arduino: manage GPS signal with GY NEO 6M and compatible devices
It is time for me (and you) to explore the Global Position System to manage some tracking system or a system to find your lost item.
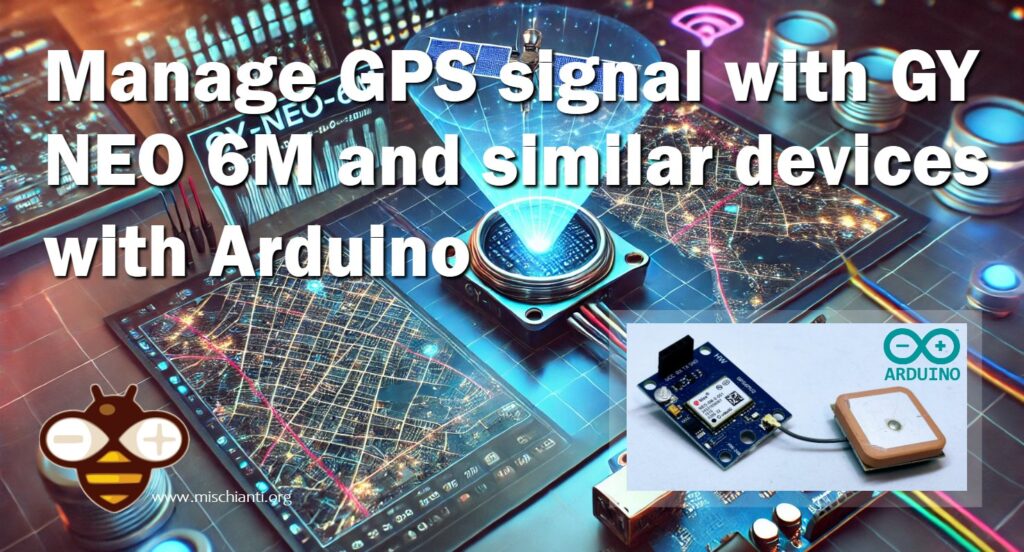
For this purpose, we are going to examine a widely used module named GY-NEO-6M.
You can find here GY-NEO-6M/F7M/8M V2
GY-NEO-6M
Neo 6m is a simple GPS receiver that uses a UART interface to communicate with the microcontroller. This simplifies a lot the interoperability and usage.
Specifications
Parameter | Specification |
---|---|
Receiver type | 50 Channels GPS L1 frequency, C/A Code SBAS: WAAS, EGNOS, MSAS |
Time-To-First-Fix1 | Cold Start: 27s Warm Start: 27s Hot Start: 1s Aided Starts: <3s |
Sensitivity | Tracking & Navigation: -161 dBm Reacquisition: -160dBm Cold Start (without aiding): -147dBm Hot Start: -156dBm |
Maximum Navigation update rate | 5Hz |
Horizontal position accuracy | GPS: 2.5m SBAS: 2.0m SBAS + PPP: < 1 m (2D, R50) SBAS + PPP: < 2 m (3D, R50) |
Configurable Timepulse frequency range | 0.25 Hz to 1 kHz |
Accuracy for Timepulse signal | RMS: 30ns 99%: <60ns Granularity: 21ns Compensated: 15ns |
Velocity accuracy | 0.1m/s |
Heading accuracy | 0.5degrees |
Operational Limits | Dynamics: =<4g Altitude: 50,000 m Velocity: 500m/s |
Datasheet
Wiring Arduino UNO
To connect the device to the Arduino UNO I’m going to use the SoftwareSerial, and I select pins 2 and 3.
The GY NEO 6M is 5v tolerant, so the wiring is very simple.
Arduino UNO | NEO 6M |
---|---|
5V | VCC |
GND | GND |
2 | Tx |
3 | Rx |
Example without library
To retrieve the data, you don’t need any library, the device stream continuously the data via the UART interface.
#include <SoftwareSerial.h>
// Choose two Arduino pins to use for software serial
int RXPin = 2;
int TXPin = 3;
//Default baud of NEO-6M is 9600
int GPSBaud = 9600;
// Create a software serial port called "gpsSerial"
SoftwareSerial gpsSerial(RXPin, TXPin);
void setup()
{
// Start the Arduino hardware serial port at 9600 baud
Serial.begin(9600);
// Start the software serial port at the GPS's default baud
gpsSerial.begin(GPSBaud);
}
void loop()
{
// Displays information when new sentence is available.
while (gpsSerial.available() > 0)
Serial.write(gpsSerial.read());
}
The result is this stream.
$GPRMC,,V,,,,,,,,,,N*53
$GPVTG,,,,,,,,,N*30
$GPGGA,,,,,,0,00,99.99,,,,,,*48
$GPGSA,A,1,,,,,,,,,,,,,99.99,99.99,99.99*30
$GPGSV,1,1,00*79
$GPGLL,,,,,,V,N*64
[...]
$GPRMC,133655.00,V,,,,,,,141022,,,N*7E
$GPVTG,,,,,,,,,N*30
$GPGGA,133655.00,,,,,0,00,99.99,,,,,,*61
$GPGSA,A,1,,,,,,,,,,,,,99.99,99.99,99.99*30
$GPGSV,1,1,03,07,,,20,09,,,27,11,,,27*76
$GPGLL,,,,,133655.00,V,N*4D
[...]
$GPRMC,134800.00,A,4322.44684,N,01231.03213,E,1.314,,141022,,,A*72
$GPVTG,,T,,M,1.314,N,2.434,K,A*25
$GPGGA,134800.00,4322.44684,N,01231.03213,E,1,04,4.83,434.4,M,44.0,M,,*51
$GPGSA,A,3,30,11,09,07,,,,,,,,,5.80,4.83,3.22*0E
$GPGSV,2,1,07,02,19,301,16,07,62,054,21,09,31,088,18,11,26,234,17*76
$GPGSV,2,2,07,14,23,159,,20,65,267,19,30,80,213,20*44
$GPGLL,4322.44684,N,01231.03213,E,134800.00,A,A*68
$GPRMC,134801.00,A,4322.44750,N,01231.03222,E,1.472,,141022,,,A*7E
$GPVTG,,T,,M,1.472,N,2.725,K,A*21
$GPGGA,134801.00,4322.44750,N,01231.03222,E,1,04,4.83,435.6,M,44.0,M,,*59
$GPGSA,A,3,30,11,09,07,,,,,,,,,5.80,4.83,3.22*0E
$GPGSV,2,1,07,02,19,301,17,07,62,054,22,09,31,088,20,11,26,234,17*7F
$GPGSV,2,2,07,14,23,159,,20,65,267,20,30,80,213,20*4E
$GPGLL,4322.44750,N,01231.03222,E,134801.00,A,A*63
The device can take a lot of time to get the signal, and It’s preferable to put It in an external environment.
But pay attention to these data; they are raw but very simple to transfer, so we’ll do some experiments in the future.
Device output
Here we are going to understand the NMEA-0183 sentences transmitted by GPS receivers. The NMEA standard provides quite a range of sentences, but many relate to non-GPS devices, and some others are GPS related but rarely used. We normally recommend the use of NMEA mode for new GPS applications to give maximum compatibility with all GPS receivers. Most GPS receivers also have a binary mode, but it is normally best to reserve the use of binary GPS protocols for applications that really require their use, such as those requiring position updates of greater than once per second.
$GPRMC Sentence (Position and time)
Recommended minimum specific GNSS data
Example (signal not acquired): $GPRMC,133655.00,V,,,,,,,141022,,,N*7E
Example (signal acquired): $GPRMC,134800.00,A,4322.44684,N,01231.03213,E,1.314,,141022,,,A*72
Field | Example | Comments |
---|---|---|
Sentence ID | $GPRMC | |
UTC Time | 134800.00 | hhmmss.sss |
Status | A | A = Valid, V = Invalid |
Latitude | 4322.44684 | ddmm.mmmm |
N/S Indicator | N | N = North, S = South |
Longitude | 01231.03213 | dddmm.mmmm |
E/W Indicator | E | E = East, W = West |
Speed over ground | 1.314 | Knots |
Course over ground | Degrees | |
UTC Date | 141022 | DDMMYY |
Magnetic variation | Degrees | |
Checksum | *72 | |
Terminator | CR/LF |
$GPVTG Sentence (Course over ground)
Course over ground and ground speed
Example (signal not acquired): $GPVTG,,,,,,,,,N*30
Example (signal acquired): $GPVTG,,T,,M,1.314,N,2.434,K,A*25
Field | Example | Comments |
---|---|---|
Sentence ID | $GPVTG | |
Course | Course in degrees | |
Reference | T | T = True heading |
Course | Course in degrees | |
Reference | M | M = Magnetic heading |
Speed | 1.314 | Horizontal speed |
Units | N | N = Knots |
Speed | 2.434 | Horizontal speed |
Units | K | K = KM/h |
Checksum | *25 | |
Terminator | CR/LF |
$GPGGA Sentence (Fix data)
Global positioning system fixed data
Example (signal not acquired): $GPGGA,,,,,,0,00,99.99,,,,,,*48
Example (signal acquired): $GPGGA,134800.00,4322.44684,N,01231.03213,E,1,04,4.83,434.4,M,44.0,M,,*51
Field | Example | Comments |
---|---|---|
Sentence ID | $GPGGA | |
UTC Time | 134800.00 | hhmmss.sss |
Latitude | 4322.44684 | ddmm.mmmm |
N/S Indicator | N | N = North, S = South |
Longitude | 01231.03213 | dddmm.mmmm |
E/W Indicator | E | E = East, W = West |
Position Fix | 1 | 0 = Invalid, 1 = Valid SPS, 2 = Valid DGPS, 3 = Valid PPS |
Satellites Used | 04 | Satellites being used (0-12) |
HDOP | 4.83 | Horizontal dilution of precision |
Altitude | 434.4 | Altitude in meters according to WGS-84 ellipsoid |
Altitude Units | M | M = Meters |
Geoid Seperation | 44.0 | Geoid seperation in meters according to WGS-84 ellipsoid |
Seperation Units | M | M = Meters |
DGPS Age | Age of DGPS data in seconds | |
DGPS Station ID | ||
Checksum | *51 | |
Terminator | CR/LF |
$GPGSA Sentence (Active satellites)
GNSS DOP and active satellites
Example (signal not acquired): $GPGSA,A,1,,,,,,,,,,,,,99.99,99.99,99.99*30
Example (signal acquired): $GPGSA,A,3,30,11,09,07,,,,,,,,,5.80,4.83,3.22*0E
Field | Example | Comments |
---|---|---|
Sentence ID | $GPGSA | |
Mode 1 | A | A = Auto 2D/3D, M = Forced 2D/3D |
Mode 1 | 3 | 1 = No fix, 2 = 2D, 3 = 3D |
Satellite used 1 | 30 | Satellite used on channel 1 |
Satellite used 2 | 11 | Satellite used on channel 2 |
Satellite used 3 | 09 | Satellite used on channel 3 |
Satellite used 4 | 07 | Satellite used on channel 4 |
Satellite used 5 | Satellite used on channel 5 | |
Satellite used 6 | Satellite used on channel 6 | |
Satellite used 7 | Satellite used on channel 7 | |
Satellite used 8 | Satellite used on channel 8 | |
Satellite used 9 | Satellite used on channel 9 | |
Satellite used 10 | Satellite used on channel 10 | |
Satellite used 11 | Satellite used on channel 11 | |
Satellite used 12 | Satellite used on channel 12 | |
PDOP | 5.80 | Position dilution of precision |
HDOP | 4.83 | Horizontal dilution of precision |
VDOP | 3.22 | Vertical dilution of precision |
Checksum | *0E | |
Terminator | CR/LF |
$GPGSV Sentence (Satellites in view)
GNSS satellites in view
Example (signal not acquired): $GPGSV,1,1,03,07,,,20,09,,,27,11,,,27*76
Example (signal acquired): $GPGSV,2,2,07,14,23,159,,20,65,267,19,30,80,213,20*44
Field | Example | Comments |
---|---|---|
Sentence ID | $GPGSV | |
Number of messages | 2 | Number of messages in complete message (1-3) |
Sequence number | 2 | Sequence number of this entry (1-3) |
Satellites in view | 07 | |
Satellite ID 1 | 14 | Range is 1-32 |
Elevation 1 | 23 | Elevation in degrees (0-90) |
Azimuth 1 | 159 | Azimuth in degrees (0-359) |
SNR 1 | Signal to noise ration in dBHZ (0-99) | |
Satellite ID 2 | 20 | Range is 1-32 |
Elevation 2 | 65 | Elevation in degrees (0-90) |
Azimuth 2 | 267 | Azimuth in degrees (0-359) |
SNR 2 | 19 | Signal to noise ration in dBHZ (0-99) |
Satellite ID 3 | 30 | Range is 1-32 |
Elevation 3 | 80 | Elevation in degrees (0-90) |
Azimuth 3 | 213 | Azimuth in degrees (0-359) |
SNR 3 | 20 | Signal to noise ration in dBHZ (0-99) |
Checksum | *44 | |
Terminator | CR/LF |
$GPGLL Sentence (Position)
Geographic position – latitude / longitude
Example (signal not acquired): $GPGLL,,,,,,V,N*64
Example (signal acquired): $GPGLL,4322.44684,N,01231.03213,E,134800.00,A,A*68
Field | Example | Comments |
---|---|---|
Sentence ID | $GPGLL | |
Latitude | 4322.44684 | ddmm.mmmm |
N/S Indicator | N | N = North, S = South |
Longitude | 01231.03213 | dddmm.mmmm |
E/W Indicator | E | E = East, W = West |
UTC Time | 134800.00 | hhmmss.sss |
Status | A | A = Valid, V = Invalid |
Checksum | *68 | |
Terminator | CR/LF |
Output examples
At the beginning you receive a message like this
$GPRMC,,V,,,,,,,,,,N*53
$GPVTG,,,,,,,,,N*30
$GPGGA,,,,,,0,00,99.99,,,,,,*48
$GPGSA,A,1,,,,,,,,,,,,,99.99,99.99,99.99*30
$GPGSV,1,1,00*79
$GPGLL,,,,,,V,N*64
$GPRMC,,V,,,,,,,,,,N*53
$GPVTG,,,,,,,,,N*30
$GPGGA,,,,,,0,00,99.99,,,,,,*48
$GPGSA,A,1,,,,,,,,,,,,,99.99,99.99,99.99*30
$GPGSV,1,1,00*79
$GPGLL,,,,,,V,N*64
The device did not blink, meaning no satellite was found.
After a while, probably, the output changed like this.
$GPRMC,133049.00,V,,,,,,,141022,,,N*75
$GPVTG,,,,,,,,,N*30
$GPGGA,133049.00,,,,,0,00,99.99,,,,,,*6A
$GPGSA,A,1,,,,,,,,,,,,,99.99,99.99,99.99*30
$GPGSV,1,1,01,07,,,22*7F
$GPGLL,,,,,133049.00,V,N*46
New data appear 133049.00
. If you check on the tables, you discover that It’s the UTC time (13:30:49.000), and 141022
the date (14/10/2022).
The next step is to start receiving some GPS information like this.
$GPRMC,133534.00,V,,,,,,,141022,,,N*7A
$GPVTG,,,,,,,,,N*30
$GPGGA,133534.00,,,,,0,00,99.99,,,,,,*65
$GPGSA,A,1,,,,,,,,,,,,,99.99,99.99,99.99*30
$GPGSV,1,1,02,07,,,21,09,,,23*77
$GPGLL,,,,,133534.00,V,N*49
In the GPGSV sentence, you can check that two satellites are found.
So when we find sufficient satellites, some positional information can be retrieved.
$GPRMC,133719.00,V,,,,,,,141022,,,N*77
$GPVTG,,,,,,,,,N*30
$GPGGA,133719.00,,,,,0,03,4.50,,,,,,*5A
$GPGSA,A,1,07,11,09,,,,,,,,,,4.61,4.50,1.00*0D
$GPGSV,2,1,05,07,66,057,16,09,34,085,28,11,29,237,28,14,19,161,*78
$GPGSV,2,2,05,20,64,278,19*49
$GPGLL,,,,,133719.00,V,N*44
And so, after a while, arrives a full set of information.
$GPRMC,134800.00,A,4322.44684,N,01231.03213,E,1.314,,141022,,,A*72
$GPVTG,,T,,M,1.314,N,2.434,K,A*25
$GPGGA,134800.00,4322.44684,N,01231.03213,E,1,04,4.83,434.4,M,44.0,M,,*51
$GPGSA,A,3,30,11,09,07,,,,,,,,,5.80,4.83,3.22*0E
$GPGSV,2,1,07,02,19,301,16,07,62,054,21,09,31,088,18,11,26,234,17*76
$GPGSV,2,2,07,14,23,159,,20,65,267,19,30,80,213,20*44
$GPGLL,4322.44684,N,01231.03213,E,134800.00,A,A*68
$GPRMC,134801.00,A,4322.44750,N,01231.03222,E,1.472,,141022,,,A*7E
$GPVTG,,T,,M,1.472,N,2.725,K,A*21
$GPGGA,134801.00,4322.44750,N,01231.03222,E,1,04,4.83,435.6,M,44.0,M,,*59
$GPGSA,A,3,30,11,09,07,,,,,,,,,5.80,4.83,3.22*0E
$GPGSV,2,1,07,02,19,301,17,07,62,054,22,09,31,088,20,11,26,234,17*7F
$GPGSV,2,2,07,14,23,159,,20,65,267,20,30,80,213,20*4E
$GPGLL,4322.44750,N,01231.03222,E,134801.00,A,A*63
Library
As usual, there are a lot of libraries to parse the GPS sentence, but one of these is surely the most used, TinyGPSPlus.
Basic example
Here is the “classic” example to retrieve the most important information from the device using the TinyGPSPlus library.
#include <TinyGPSPlus.h>
#include <SoftwareSerial.h>
/*
This sample code demonstrates the normal use of a TinyGPSPlus (TinyGPSPlus) object.
It requires the use of SoftwareSerial, and assumes that you have a
9600-baud serial GPS device hooked up on pins 4(rx) and 3(tx).
*/
static const int RXPin = 2, TXPin = 3;
static const uint32_t GPSBaud = 9600;
// The TinyGPSPlus object
TinyGPSPlus gps;
// The serial connection to the GPS device
SoftwareSerial ss(RXPin, TXPin);
void setup()
{
Serial.begin(9600);
ss.begin(GPSBaud);
Serial.println(F("FullExample.ino"));
Serial.println(F("An extensive example of many interesting TinyGPSPlus features"));
Serial.print(F("Testing TinyGPSPlus library v. ")); Serial.println(TinyGPSPlus::libraryVersion());
Serial.println(F("by Mikal Hart"));
Serial.println();
Serial.println(F("Sats HDOP Latitude Longitude Fix Date Time Date Alt Course Speed Card Distance Course Card Chars Sentences Checksum"));
Serial.println(F(" (deg) (deg) Age Age (m) --- from GPS ---- ---- to London ---- RX RX Fail"));
Serial.println(F("----------------------------------------------------------------------------------------------------------------------------------------"));
}
void loop()
{
static const double LONDON_LAT = 51.508131, LONDON_LON = -0.128002;
printInt(gps.satellites.value(), gps.satellites.isValid(), 5);
printFloat(gps.hdop.hdop(), gps.hdop.isValid(), 6, 1);
printFloat(gps.location.lat(), gps.location.isValid(), 11, 6);
printFloat(gps.location.lng(), gps.location.isValid(), 12, 6);
printInt(gps.location.age(), gps.location.isValid(), 5);
printDateTime(gps.date, gps.time);
printFloat(gps.altitude.meters(), gps.altitude.isValid(), 7, 2);
printFloat(gps.course.deg(), gps.course.isValid(), 7, 2);
printFloat(gps.speed.kmph(), gps.speed.isValid(), 6, 2);
printStr(gps.course.isValid() ? TinyGPSPlus::cardinal(gps.course.deg()) : "*** ", 6);
unsigned long distanceKmToLondon =
(unsigned long)TinyGPSPlus::distanceBetween(
gps.location.lat(),
gps.location.lng(),
LONDON_LAT,
LONDON_LON) / 1000;
printInt(distanceKmToLondon, gps.location.isValid(), 9);
double courseToLondon =
TinyGPSPlus::courseTo(
gps.location.lat(),
gps.location.lng(),
LONDON_LAT,
LONDON_LON);
printFloat(courseToLondon, gps.location.isValid(), 7, 2);
const char *cardinalToLondon = TinyGPSPlus::cardinal(courseToLondon);
printStr(gps.location.isValid() ? cardinalToLondon : "*** ", 6);
printInt(gps.charsProcessed(), true, 6);
printInt(gps.sentencesWithFix(), true, 10);
printInt(gps.failedChecksum(), true, 9);
Serial.println();
smartDelay(1000);
if (millis() > 5000 && gps.charsProcessed() < 10)
Serial.println(F("No GPS data received: check wiring"));
}
// This custom version of delay() ensures that the gps object
// is being "fed".
static void smartDelay(unsigned long ms)
{
unsigned long start = millis();
do
{
while (ss.available())
gps.encode(ss.read());
} while (millis() - start < ms);
}
static void printFloat(float val, bool valid, int len, int prec)
{
if (!valid)
{
while (len-- > 1)
Serial.print('*');
Serial.print(' ');
}
else
{
Serial.print(val, prec);
int vi = abs((int)val);
int flen = prec + (val < 0.0 ? 2 : 1); // . and -
flen += vi >= 1000 ? 4 : vi >= 100 ? 3 : vi >= 10 ? 2 : 1;
for (int i=flen; i<len; ++i)
Serial.print(' ');
}
smartDelay(0);
}
static void printInt(unsigned long val, bool valid, int len)
{
char sz[32] = "*****************";
if (valid)
sprintf(sz, "%ld", val);
sz[len] = 0;
for (int i=strlen(sz); i<len; ++i)
sz[i] = ' ';
if (len > 0)
sz[len-1] = ' ';
Serial.print(sz);
smartDelay(0);
}
static void printDateTime(TinyGPSDate &d, TinyGPSTime &t)
{
if (!d.isValid())
{
Serial.print(F("********** "));
}
else
{
char sz[32];
sprintf(sz, "%02d/%02d/%02d ", d.month(), d.day(), d.year());
Serial.print(sz);
}
if (!t.isValid())
{
Serial.print(F("******** "));
}
else
{
char sz[32];
sprintf(sz, "%02d:%02d:%02d ", t.hour(), t.minute(), t.second());
Serial.print(sz);
}
printInt(d.age(), d.isValid(), 5);
smartDelay(0);
}
static void printStr(const char *str, int len)
{
int slen = strlen(str);
for (int i=0; i<len; ++i)
Serial.print(i<slen ? str[i] : ' ');
smartDelay(0);
}
The output at the beginning is this.
An extensive example of many interesting TinyGPSPlus features
Testing TinyGPSPlus library v. 1.0.2
by Mikal Hart
Sats HDOP Latitude Longitude Fix Date Time Date Alt Course Speed Card Distance Course Card Chars Sentences Checksum
(deg) (deg) Age Age (m) --- from GPS ---- ---- to London ---- RX RX Fail
----------------------------------------------------------------------------------------------------------------------------------------
0 100.0 ********** *********** **** 00/00/2000 00:00:00 561 ****** ****** ***** *** ******** ****** *** 225 0 0
0 100.0 ********** *********** **** 00/00/2000 00:00:00 640 ****** ****** ***** *** ******** ****** *** 387 0 0
0 100.0 ********** *********** **** 00/00/2000 00:00:00 717 ****** ****** ***** *** ******** ****** *** 549 0 0
0 100.0 ********** *********** **** 00/00/2000 00:00:00 796 ****** ****** ***** *** ******** ****** *** 711 0 0
0 100.0 ********** *********** **** 00/00/2000 00:00:00 873 ****** ****** ***** *** ******** ****** *** 873 0 0
0 100.0 ********** *********** **** 00/00/2000 00:00:00 952 ****** ****** ***** *** ******** ****** *** 1070 0 0
0 100.0 ********** *********** **** 00/00/2000 00:00:00 54 ****** ****** ***** *** ******** ****** *** 1313 0 0
0 100.0 ********** *********** **** 00/00/2000 00:00:00 132 ****** ****** ***** *** ******** ****** *** 1521 0 0
0 100.0 ********** *********** **** 00/00/2000 00:00:00 187 ****** ****** ***** *** ******** ****** *** 1683 0 0
0 100.0 ********** *********** **** 00/00/2000 00:00:00 266 ****** ****** ***** *** ******** ****** *** 1845 0 0
0 100.0 ********** *********** **** 00/00/2000 00:00:00 344 ****** ****** ***** *** ******** ****** *** 2007 0 0
0 100.0 ********** *********** **** 00/00/2000 00:00:00 421 ****** ****** ***** *** ******** ****** *** 2169 0 0
0 100.0 ********** *********** **** 00/00/2000 00:00:00 501 ****** ****** ***** *** ******** ****** *** 2331 0 0
0 100.0 ********** *********** **** 00/00/2000 00:00:00 578 ****** ****** ***** *** ******** ****** *** 2493 0 0
0 100.0 ********** *********** **** 00/00/2000 00:00:00 657 ****** ****** ***** *** ******** ****** *** 2655 0 0
0 100.0 ********** *********** **** 00/00/2000 00:00:00 735 ****** ****** ***** *** ******** ****** *** 2817 0 0
0 100.0 ********** *********** **** 00/00/2000 00:00:00 813 ****** ****** ***** *** ******** ****** *** 2979 0 0
0 100.0 ********** *********** **** 00/00/2000 00:00:00 891 ****** ****** ***** *** ******** ****** *** 3141 0 0
0 100.0 ********** *********** **** 00/00/2000 00:00:00 968 ****** ****** ***** *** ******** ****** *** 3354 0 0
0 100.0 ********** *********** **** 00/00/2000 00:00:00 72 ****** ****** ***** *** ******** ****** *** 3598 0 0
0 100.0 ********** *********** **** 00/00/2000 00:00:00 151 ****** ****** ***** *** ******** ****** *** 3805 0 0
0 100.0 ********** *********** **** 00/00/2000 00:00:00 206 ****** ****** ***** *** ******** ****** *** 3983 0 0
0 100.0 ********** *********** **** 00/00/2000 00:00:00 284 ****** ****** ***** *** ******** ****** *** 4153 0 0
0 100.0 ********** *********** **** 00/00/2000 00:00:00 360 ****** ****** ***** *** ******** ****** *** 4323 0 0
0 100.0 ********** *********** **** 00/00/2000 00:00:00 438 ****** ****** ***** *** ******** ****** *** 4493 0 0
0 100.0 ********** *********** **** 00/00/2000 00:00:00 518 ****** ****** ***** *** ******** ****** *** 4655 0 0
0 100.0 ********** *********** **** 00/00/2000 00:00:00 595 ****** ****** ***** *** ******** ****** *** 4817 0 0
0 100.0 ********** *********** **** 00/00/2000 00:00:00 674 ****** ****** ***** *** ******** ****** *** 4979 0 0
0 100.0 ********** *********** **** 00/00/2000 00:00:00 751 ****** ****** ***** *** ******** ****** *** 5141 0 0
0 100.0 ********** *********** **** 00/00/2000 00:00:00 830 ****** ****** ***** *** ******** ****** *** 5303 0 0
0 100.0 ********** *********** **** 00/00/2000 00:00:00 907 ****** ****** ***** *** ******** ****** *** 5465 0 0
0 100.0 ********** *********** **** 00/00/2000 00:00:00 1010 ****** ****** ***** *** ******** ****** *** 5703 0 0
0 100.0 ********** *********** **** 00/00/2000 00:00:00 91 ****** ****** ***** *** ******** ****** *** 5939 0 0
When the date is retrieved.
0 100.0 ********** *********** **** 10/19/2022 10:42:50 224 ****** ****** ***** *** ******** ****** *** 16039 0 0
0 100.0 ********** *********** **** 10/19/2022 10:42:51 168 ****** ****** ***** *** ******** ****** *** 16059 0 0
0 100.0 ********** *********** **** 10/19/2022 10:42:52 382 ****** ****** ***** *** ******** ****** *** 16079 0 0
0 100.0 ********** *********** **** 10/19/2022 10:42:53 462 ****** ****** ***** *** ******** ****** *** 16100 0 0
0 100.0 ********** *********** **** 10/19/2022 10:42:54 539 ****** ****** ***** *** ******** ****** *** 16120 0 0
0 100.0 ********** *********** **** 10/19/2022 10:42:55 617 ****** ****** ***** *** ******** ****** *** 16140 0 0
0 100.0 ********** *********** **** 10/19/2022 10:42:56 693 ****** ****** ***** *** ******** ****** *** 16160 0 0
0 100.0 ********** *********** **** 10/19/2022 10:42:57 610 ****** ****** ***** *** ******** ****** *** 16181 0 0
0 100.0 ********** *********** **** 10/19/2022 10:42:58 850 ****** ****** ***** *** ******** ****** *** 16202 0 0
0 100.0 ********** *********** **** 10/19/2022 10:42:59 927 ****** ****** ***** *** ******** ****** *** 16224 0 0
0 100.0 ********** *********** **** 10/19/2022 10:43:01 32 ****** ****** ***** *** ******** ****** *** 16254 0 0
0 100.0 ********** *********** **** 10/19/2022 10:43:02 113 ****** ****** ***** *** ******** ****** *** 16281 0 0
0 100.0 ********** *********** **** 10/19/2022 10:43:03 32 ****** ****** ***** *** ******** ****** *** 16294 0 0
And finally, when GPS data was retrieved.
5 1.5 43.374248 12.517117 762 10/19/2022 12:13:56 878 420.50 328.72 0.02 NNW 1309 318.10 NW 15926 1924 0
5 1.5 43.374248 12.517118 1 10/19/2022 12:13:58 16 420.40 328.72 0.33 NNW 1309 318.10 NW 15932 1927 0
5 1.5 43.374248 12.517124 42 10/19/2022 12:13:59 171 420.10 328.72 0.57 NNW 1309 318.10 NW 15938 1930 0
5 1.5 43.374248 12.517124 178 10/19/2022 12:14:00 308 419.90 328.72 0.28 NNW 1309 318.10 NW 15944 1932 0
5 1.5 43.374248 12.517126 294 10/19/2022 12:14:01 410 419.70 328.72 0.30 NNW 1309 318.10 NW 15949 1934 0
5 1.5 43.374244 12.517125 361 10/19/2022 12:14:02 477 419.60 328.72 0.15 NNW 1309 318.10 NW 15953 1936 0
5 1.5 43.374244 12.517123 440 10/19/2022 12:14:03 555 419.60 328.72 0.54 NNW 1309 318.10 NW 15958 1938 0
5 1.5 43.374244 12.517125 514 10/19/2022 12:14:04 630 419.30 328.72 0.06 NNW 1309 318.10 NW 15962 1940 0
5 1.5 43.374244 12.517127 592 10/19/2022 12:14:05 708 419.10 328.72 0.15 NNW 1309 318.10 NW 15967 1942 0
5 1.5 43.374244 12.517127 670 10/19/2022 12:14:06 784 419.20 328.72 0.11 NNW 1309 318.10 NW 15972 1944 0
5 1.5 43.374244 12.517127 748 10/19/2022 12:14:07 863 419.30 328.72 0.28 NNW 1309 318.10 NW 15976 1946 0
5 1.5 43.374244 12.517126 873 10/19/2022 12:14:08 1001 419.40 328.72 0.20 NNW 1309 318.10 NW 15982 1949 0
5 1.5 43.374244 12.517127 17 10/19/2022 12:14:10 154 419.50 328.72 0.30 NNW 1309 318.10 NW 15988 1952 0
5 1.5 43.374244 12.517125 167 10/19/2022 12:14:11 296 419.50 328.72 0.46 NNW 1309 318.10 NW 15994 1954 0
5 1.5 43.374244 12.517127 294 10/19/2022 12:14:12 408 419.30 328.72 0.07 NNW 1309 318.10 NW 15999 1956 0
5 1.5 43.374244 12.517127 344 10/19/2022 12:14:13 460 419.30 328.72 0.15 NNW 1309 318.10 NW 16004 1958 0
5 1.5 43.374244 12.517130 425 10/19/2022 12:14:14 541 419.10 328.72 0.19 NNW 1309 318.10 NW 16008 1960 0
5 1.5 43.374244 12.517133 504 10/19/2022 12:14:15 618 418.80 328.72 0.41 NNW 1309 318.10 NW 16013 1962 0
Simplified example
Another simple sketch can be this, retrieve only the basic information.
#include "Arduino.h"
#include "SoftwareSerial.h"
#include <TinyGPS++.h>
static const int RXPin = 2, TXPin = 3;
static const uint32_t GPSBaud = 9600;
// The TinyGPSPlus object
TinyGPSPlus gps;
// The serial connection to the GPS device
SoftwareSerial gpsSerial(RXPin, TXPin);
//The setup function is called once at startup of the sketch
void setup()
{
Serial.begin(9600);
Serial.println(PSTR("\nOne Wire Half Duplex Serial Tester"));
gpsSerial.begin(9600, EspSoftwareSerial::SWSERIAL_8N1, 18, 18, false, 256);
gpsSerial.enableIntTx(false);
}
void loop()
{
while (gpsSerial.available()) // check for gps data
{
// Serial.write(gpsSerial.read());
// Serial.println(gpsSerial.read());
if (gps.encode(gpsSerial.read())) // encode gps data
{
Serial.print("SATS: ");
Serial.println(gps.satellites.value());
Serial.print("LAT: ");
Serial.println(gps.location.lat(), 6);
Serial.print("LONG: ");
Serial.println(gps.location.lng(), 6);
Serial.print("ALT: ");
Serial.println(gps.altitude.meters());
Serial.print("SPEED: ");
Serial.println(gps.speed.mps());
Serial.print("Date: ");
Serial.print(gps.date.day()); Serial.print("/");
Serial.print(gps.date.month()); Serial.print("/");
Serial.println(gps.date.year());
Serial.print("Hour: ");
Serial.print(gps.time.hour()); Serial.print(":");
Serial.print(gps.time.minute()); Serial.print(":");
Serial.println(gps.time.second());
Serial.println("---------------------------");
delay(4000);
}
//else {
// Serial.println("ERROR!");
// }
}
}
The result is this.
---------------------------
SATS: 0
LAT: 0.000000
LONG: 0.000000
ALT: 0.00
SPEED: 0.00
Date: 0/0/2000
Hour: 0:0:0
---------------------------
SATS: 0
LAT: 0.000000
LONG: 0.000000
ALT: 0.00
SPEED: 0.00
Date: 0/0/2000
Hour: 0:0:0
---------------------------
When acquire the coordinate.
---------------------------
SATS: 8
LAT: 43.374256
LONG: 12.517083
ALT: 411.30
SPEED: 0.04
Date: 16/4/2024
Hour: 7:26:39
---------------------------
SATS: 8
LAT: 43.374256
LONG: 12.517083
ALT: 411.30
SPEED: 0.04
Date: 16/4/2024
Hour: 7:26:39
---------------------------
Thanks
- Arduino Remote/wireless Programming
- BMP280, DHT11 and DHT22, DHT12, Dallas Temperature ds18b20, Thermistor
- ATtiny Programmer Board (ArduinoUNO As ISP)
- Send email with esp8266 and Arduino (Library v1.x)
- How to use SD card with esp8266 and Arduino
- Ebyte LoRa E32 device for Arduino, esp32 or esp8266: WOR (wake on radio) microcontroller and new Arduino shield
- Manage JSON file with Arduino, esp32 and esp8266
- How to interface Arduino, esp8266 or esp32 to RS-485
- Send emails with attachments (v2.x library): Arduino Ethernet
- WebSocket
- Arduino AVR: compiled binary (.hex) from command line and GUI tool
- Arduino: fast external SPI Flash memory
- GY-291 ADXL345 i2c spi accelerometer with interrupt for esp32, esp8266, stm32 and Arduino
- i2c Arduino: how to create network, parameters and address scanner
- GY-273 QMC5883L clone HMC5883L magnetometer for Arduino, esp8266 and esp32
- WiFi remote debugging of an Arduino with DT-06
- Program Arduino UNO Remotely via WiFi with DT-06 ESP-Link Firmware
- Introduction to Remote Programming of Arduino UNO via WiFi with ESP8266
- Remote WiFi debugging on Arduino Using ESP8266 (NodeMCU and ESP01) with ESP-LINK Firmware
- Arduino: manage GPS signal with GY NEO 6M and similar devices