BNO055: power modes, accelerometer and motion interrupt – 4
In this article, we are going to explore the power modes of BON055 and focus on accelerometer features and management.
The BNO055 is a System in Package (SiP), integrating a triaxial 14-bit accelerometer, a triaxial 16-bit gyroscope with a range of ±2000 degrees per second, a triaxial geomagnetic sensor, and a 32-bit cortex M0+ microcontroller running Bosch Sensortec sensor fusion software, in a single package.
Architecture
I take this image from the datasheet as a reminder that this sensor has 3 sensors and a microcontroller with “fusion software” to handle the data and give you an absolute position.
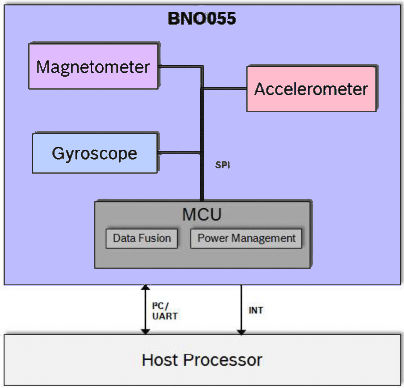
BNO055 pinouts
There are many different forms of this sensor, I choose the smallest and cheap.
Here the module Aliexpress
All these modules have the same features, but to enable them, you must do different operations.
This is the clone I use:
And here is the Adafruit one:
The sensor supports a 3.3v logic level, but the module can be powered by 5v.
Power Modes
The BNO055 support three different power modes: Normal mode, Low Power Mode, and Suspend mode.
Normal Mode
In normal mode, all sensors required for the selected operating mode are always switched ON. The MCU’s register map and internal peripherals are always operative in this mode.
The upper examples are all in this default modality.
Suspend Mode
In suspend mode, the system is paused, and all the sensors and the microcontroller are put into sleep mode. No values in the register map will be updated in this mode.
Here is a simple sketch that puts in suspension mode
bno055_set_powermode(POWER_MODE_SUSPEND);
and then restore the normal status.
bno055_set_powermode(POWER_MODE_NORMAL);
//Configuration to NDoF mode
bno055_set_operation_mode(OPERATION_MODE_NDOF);
The complete sketch:
/**
* An example that start bno055 in normal mode
* than suspend It and restore after 5 secs
*
* by Renzo Mischianti <www.mischianti.org>
*
* https://mischianti.org/
*/
#include "BNO055_support.h" //Contains the bridge code between the API and Arduino
#include <Wire.h>
//The device address is set to BNO055_I2C_ADDR2 in this example. You can change this in the BNO055.h file in the code segment shown below.
// /* bno055 I2C Address */
// #define BNO055_I2C_ADDR1 0x28
// #define BNO055_I2C_ADDR2 0x29
// #define BNO055_I2C_ADDR BNO055_I2C_ADDR2
//Pin assignments as tested on the Arduino Due.
//Vdd,Vddio : 3.3V
//GND : GND
//SDA/SCL : SDA/SCL
//PSO/PS1 : GND/GND (I2C mode)
//This structure contains the details of the BNO055 device that is connected. (Updated after initialization)
struct bno055_t myBNO;
struct bno055_euler myEulerData; //Structure to hold the Euler data
unsigned char accelCalibStatus = 0; //Variable to hold the calibration status of the Accelerometer
unsigned char magCalibStatus = 0; //Variable to hold the calibration status of the Magnetometer
unsigned char gyroCalibStatus = 0; //Variable to hold the calibration status of the Gyroscope
unsigned char sysCalibStatus = 0; //Variable to hold the calibration status of the System (BNO055's MCU)
unsigned long lastTime = 0;
/* Set the delay between fresh samples */
#define BNO055_SAMPLERATE_DELAY_MS (1000)
void setup() //This code is executed once
{
//Initialize I2C communication
Wire.begin();
//Initialization of the BNO055
BNO_Init(&myBNO); //Assigning the structure to hold information about the device
//Configuration to NDoF mode
bno055_set_operation_mode(OPERATION_MODE_NDOF);
delay(1);
//Initialize the Serial Port to view information on the Serial Monitor
Serial.begin(115200);
}
bool suspended = false;
bool reactivated = false;
void loop() //This code is looped forever
{
if ((millis() - lastTime) >= BNO055_SAMPLERATE_DELAY_MS) //To stream at 10Hz without using additional timers
{
lastTime = millis();
bno055_read_euler_hrp(&myEulerData); //Update Euler data into the structure
/* The WebSerial 3D Model Viewer expects data as heading, pitch, roll */
Serial.print(F("Orientation: "));
Serial.print(360-(float(myEulerData.h) / 16.00));
Serial.print(F(", "));
Serial.print(360-(float(myEulerData.p) / 16.00));
Serial.print(F(", "));
Serial.print(360-(float(myEulerData.r) / 16.00));
Serial.println(F(""));
bno055_get_accelcalib_status(&accelCalibStatus);
bno055_get_gyrocalib_status(&gyroCalibStatus);
bno055_get_syscalib_status(&sysCalibStatus);
bno055_get_magcalib_status(&magCalibStatus);
Serial.print(F("Calibration: "));
Serial.print(sysCalibStatus, DEC);
Serial.print(F(", "));
Serial.print(gyroCalibStatus, DEC);
Serial.print(F(", "));
Serial.print(accelCalibStatus, DEC);
Serial.print(F(", "));
Serial.print(magCalibStatus, DEC);
Serial.println(F(""));
}
if (millis() > 10000 && suspended == false) {
Serial.println("SUSPENDED!");
bno055_set_powermode(POWER_MODE_SUSPEND);
suspended = true;
}
if (millis() > 15000 && reactivated == false) {
Serial.println("REACTIVATED!");
bno055_set_powermode(POWER_MODE_NORMAL);
//Configuration to NDoF mode
bno055_set_operation_mode(OPERATION_MODE_NDOF);
reactivated = true;
}
}
And here is the result:
Orientation: 280.37, 344.56, 325.44
Calibration: 0, 3, 3, 3
Orientation: 265.69, 334.94, 399.44
Calibration: 0, 3, 3, 3
Orientation: 285.50, 364.25, 326.12
Calibration: 0, 3, 3, 3
Orientation: 272.62, 327.37, 422.31
Calibration: 0, 3, 3, 3
Orientation: 272.37, 333.19, 420.56
Calibration: 0, 3, 3, 3
Orientation: 272.75, 335.37, 419.87
Calibration: 0, 3, 3, 3
Orientation: 276.62, 356.37, 344.31
Calibration: 0, 3, 3, 3
SUSPENDED!
Orientation: 360.00, 360.00, 360.00
Calibration: 0, 0, 0, 0
Orientation: 360.00, 360.00, 360.00
Calibration: 0, 0, 0, 0
Orientation: 360.00, 360.00, 360.00
Calibration: 0, 0, 0, 0
Orientation: 360.00, 360.00, 360.00
Calibration: 0, 0, 0, 0
Orientation: 360.00, 360.00, 360.00
Calibration: 0, 0, 0, 0
REACTIVATED!
Orientation: 276.87, 358.00, 357.69
Calibration: 0, 3, 3, 3
Orientation: 274.69, 343.81, 393.12
Calibration: 0, 3, 3, 3
Orientation: 277.25, 345.75, 412.94
Low Power Mode
If no activity (i.e. no motion) is detected for a configurable duration (default 5 seconds), the BNO055 enters the low power mode. In this mode, only the accelerometer is active. Once motion is detected (i.e. the accelerometer signals an any-motion interrupt), the system is woken up, and normal mode is entered. The following settings are possible.
Description | Parameter | Value |
---|---|---|
Entering to sleep: NO Motion Interrupt | Detection Type | No Motion |
Detection Axis | ||
Params | Duration | |
Threshold |
Description | Parameter | Value |
---|---|---|
Waking up: Any Motion Interrupt | Detection Type | Detection Axis |
Params | Duration | |
Threshold |
Additionally, the interrupt pins can also be configured to provide HW interrupt to the host.
The BNO055 is, by default, configured to have optimum values for entering into sleep and waking up. To restore these values, trigger a system reset by a software reset.
There are some limitations to achieving the low power mode performance:
- Only No and Any motion interrupts are applicable, and High-G and slow motion interrupts are not applicable in low power mode.
- The low power mode is not applicable where the accelerometer is not employed.
Interrupts
bno055 interrupts
INT is configured as an interrupt pin for signaling an interrupt to the host. The interrupt trigger is configured as a raising edge and is latched onto the INT pin. Once an interrupt occurs, the INT pin is set to high and will remain high until it is reset by the host. This can be done by setting RST_INT in the SYS_TRIGGER register.
bno055_set_reset_int(1);
Interrupts can be enabled by setting the corresponding bit in the interrupt enable register (INT_EN) and disabled when it is cleared.
bno055_get_int_gyro_anymotion(&gyro_am);
bno055_set_int_gyro_anymotion(1);
bno055_get_int_gyro_highrate(&gyro_hr);
bno055_set_int_gyro_highrate(1);
bno055_get_int_accel_high_g(&accel_hg);
bno055_set_int_accel_high_g(1);
bno055_get_int_accel_anymotion(&accel_am);
bno055_set_int_accel_anymotion(1);
bno055_get_int_accel_nomotion(&accel_nm);
bno055_set_int_accel_nomotion(1);
Interrupt Pin Masking
bno055_get_intmsk_gyro_anymotion(1);
bno055_set_intmsk_gyro_anymotion(1);
bno055_get_intmsk_gyro_highrate(1);
bno055_set_intmsk_gyro_highrate(1);
bno055_get_intmsk_accel_high_g(1);
bno055_set_intmsk_accel_high_g(1);
bno055_get_intmsk_accel_anymotion(1);
bno055_set_intmsk_accel_anymotion(1);
bno055_get_intmsk_accel_nomotion(1);
bno055_set_intmsk_accel_nomotion(1);
Interrupts can be routed to the INT pin by setting the corresponding interrupt bit in the INT_MSK register.
Interrupt Status
Interrupt occurrences are stored in the interrupt status register (INT_STA). All bits in this register are cleared on reading.
bno055_get_interrupt_status_gyro_anymotion(&gyr_anymotion);
bno055_get_interrupt_status_gyro_highrate(&gyr_highrate);
bno055_get_interrupt_status_accel_highg(&acc_highg);
bno055_get_interrupt_status_accel_anymotion(&acc_anymotion);
bno055_get_interrupt_status_accel_nomotion(&acc_nomotion);
Interrupt Settings
Data Ready Interrupt (DRDY INT)
Data Ready Interrupt is a signal to the host about the data availability in the register map, so that host can read the sensor data immediately when the sensor data is available in the register map.
The following data-ready interrupts are available,
- Accelerometer or fusion data ready interrupt (ACC_BSX_DRDY)
- Magnetometer data ready interrupt (MAG_DRDY)
- Gyroscope data ready interrupt (GYR_DRDY)
In Non-fusion mode:
- In Non-fusion mode, the data ready interrupt is signaled based on individual sensor data rates configured to the sensors.
- The data ready interrupt “ACC_BSX_DRDY“ is set/triggered when the Accelerometer data is available in the register map at the configured data rate of the sensor in the operation mode where the Accelerometer is ON.
- The data ready interrupt “MAG_DRDY“ is set/triggered when the Magnetometer data is available in the register map at the configured data rate of the sensor in the operation mode where the Magnetometer is ON.
In fusion mode:
- In fusion mode, the data-ready interrupt is signaled based on the primary sensor data rate. The data ready interrupt “ACC_BSX_DRDY“ is set/triggered when the calibrated/fusion data is available in the register map at the primary rate of the sensor.
Data Ready Interrupt Behavior:
Once an interrupt occurs, the INT pin is set to high and will remain high until it is reset or read by the host. The INT is set to low and high immediately when multiple interrupts occur before the host reset or read.
Accelerometer Slow/No Motion Interrupt
Params | Value |
---|---|
Detection Type | No Motion |
Slow Motion | |
Interrupt Parameters | Threshold |
Duration | |
Axis selection | X-axis |
Y-axis | |
Z-axis |
The slow-motion/no-motion interrupt engine can be configured in two modes.
You can select slow motion with this command:
bno055_set_accel_slow_no_motion_enable(0);
or no motion with this:
bno055_set_accel_slow_no_motion_enable(1);
Slow-motion Interrupt is triggered when the measured slope of at least one enabled axis exceeds the programmable slope threshold for a programmable number of samples.
To get the current value of the threshold
unsigned char accel_slow_no_thr = 0;
bno055_get_accel_slow_no_threshold(&accel_slow_no_thr);
or to set
bno055_set_accel_slow_no_threshold(10);
Hence the engine behaves similarly to the any-motion interrupt but with a different set of parameters. In order to suppress false triggers, the interrupt is only generated (cleared) if a certain number of consecutive slope data points is larger (smaller) than the slope threshold given by the number = + 1.
In no-motion mode, an interrupt is generated if the slope on all selected axes remains smaller than a programmable threshold for a programmable delay time.
The scaling of the threshold value is identical to that of the slow-motion interrupt.
To get the duration, you can use this command:
unsigned char accel_am_dur = 0;
bno055_get_accel_anymotion_duration(&accel_am_dur);
and to set, you can use
bno055_set_accel_anymotion_duration(3); // to set 4secs
However, in no-motion mode, register slo_no_mot_dur defines the delay time before the no-motion interrupt is triggered.
Value | Delay |
---|---|
0 | 1 s |
1 | 2 s |
2 | 3 s |
… | … |
14 | 15 s |
15 | 16 s |
16 | 40 s |
17 | 48 s |
18 | 56 s |
19 | 64 s. |
20 | 72 s |
21 | 80 s |
32 | 88 s |
33 | 96 s |
34 | 104 s |
… | … |
62 | 328 s |
63 | 336 s |
Accelerometer Any Motion Interrupt
Params | Value |
---|---|
Interrupt Parameters | Threshold |
Duration | |
Axis selection | X-axis |
Y-axis | |
Z-axis |
The any-motion interrupt uses the slope between successive acceleration signals to detect changes in motion. An interrupt is generated when the slope (absolute value of acceleration difference) exceeds a preset threshold. It is cleared as soon as the slope falls below the threshold.
Any motion detection can be enabled (disabled) for each axis separately. The criteria for any motion detection are fulfilled, and the slope interrupt is generated if the slope of any of the enabled axes exceeds the threshold for a set of consecutive times. As soon as the slopes of all enabled axes fall or stay below this threshold for a set of consecutive times, the interrupt is cleared unless the interrupt signal is latched.
As described, no-motion interrupts are the basic interrupt in LOW POWER mode; by default, no-motion and any motion are enabled by default, but not the interrupt bit mask to redirect the interrupt on the INT pin also.
Interrupt on registers
So if we are going to execute this simple sketch:
/**
* bno055 simple interrupt management
* enable only register interrupt for any motion and
* no motion in LOW POWER mode
* no bit mask for interrupt pin
*
* by Renzo Mischianti <www.mischianti.org>
*
* https://mischianti.org/
*/
#include "BNO055_support.h" //Contains the bridge code between the API and Arduino
#include <Wire.h>
//The device address is set to BNO055_I2C_ADDR2 in this example. You can change this in the BNO055.h file in the code segment shown below.
// /* bno055 I2C Address */
// #define BNO055_I2C_ADDR1 0x28
// #define BNO055_I2C_ADDR2 0x29
// #define BNO055_I2C_ADDR BNO055_I2C_ADDR2
//Pin assignments as tested on the Arduino Due.
//Vdd,Vddio : 3.3V
//GND : GND
//SDA/SCL : SDA/SCL
//PSO/PS1 : GND/GND (I2C mode)
//This structure contains the details of the BNO055 device that is connected. (Updated after initialization)
struct bno055_t myBNO;
struct bno055_euler myEulerData; //Structure to hold the Euler data
unsigned long lastTime = 0;
/* Set the delay between fresh samples */
#define BNO055_SAMPLERATE_DELAY_MS (1000)
void getIntInterruptEnabled();
void getIntInterruptBitMaskEnabled();
void getInterruptStatusEnabled(bool synthetic = false);
void printSlowMotionNoMotionParameters();
void setup() //This code is executed once
{
//Initialize I2C communication
Wire.begin();
//Initialization of the BNO055
BNO_Init(&myBNO); //Assigning the structure to hold information about the device
// bno055_set_reset_sys(1);
delay(1500);
bno055_set_powermode(POWER_MODE_LOW_POWER);
//Configuration to NDoF mode
bno055_set_operation_mode(OPERATION_MODE_NDOF);
// Activate SLOW MOTION
// bno055_set_accel_slow_no_motion_enable(0);
// Activate NO MOTION (default)
bno055_set_accel_slow_no_motion_enable(1);
delay(1);
//Initialize the Serial Port to view information on the Serial Monitor
Serial.begin(115200);
delay(1000);
getIntInterruptEnabled();
getIntInterruptBitMaskEnabled();
printSlowMotionNoMotionParameters();
}
bool suspended = false;
bool reactivated = false;
void loop() //This code is looped forever
{
if ((millis() - lastTime) >= BNO055_SAMPLERATE_DELAY_MS) //To stream at 10Hz without using additional timers
{
lastTime = millis();
bno055_read_euler_hrp(&myEulerData); //Update Euler data into the structure
Serial.print(millis()/1000); Serial.print("Secs - ");
/* The WebSerial 3D Model Viewer expects data as heading, pitch, roll */
Serial.print(F("Orientation: "));
Serial.print(360-(float(myEulerData.h) / 16.00));
Serial.print(F(", "));
Serial.print(360-(float(myEulerData.p) / 16.00));
Serial.print(F(", "));
Serial.print(360-(float(myEulerData.r) / 16.00));
Serial.print(F(" - "));
getInterruptStatusEnabled(true);
}
}
/*
* Print the interrupt enabled
*/
void getIntInterruptEnabled() {
unsigned char gyro_am = 0;
unsigned char gyro_hr = 0;
unsigned char accel_hg = 0;
unsigned char accel_am = 0;
unsigned char accel_nm = 0;
bno055_get_int_gyro_anymotion(&gyro_am);
bno055_get_int_gyro_highrate(&gyro_hr);
bno055_get_int_accel_high_g(&accel_hg);
bno055_get_int_accel_anymotion(&accel_am);
bno055_get_int_accel_nomotion(&accel_nm);
Serial.println( "---- Interrupt enabled ----" );
Serial.print( "Gyro Any Motion " );
Serial.println( gyro_am );
Serial.print( "Gyro High Rate " );
Serial.println( gyro_hr );
Serial.print( "Accel High G " );
Serial.println( accel_hg );
Serial.print( "Accel Any Motion " );
Serial.println( accel_am );
Serial.print( "Accel No Motion " );
Serial.println( accel_nm );
Serial.println( "---------------------------" );
}
/*
* Print the interrupt to send on interrupt pin
*/
void getIntInterruptBitMaskEnabled() {
unsigned char gyro_am = 0;
unsigned char gyro_hr = 0;
unsigned char accel_hg = 0;
unsigned char accel_am = 0;
unsigned char accel_nm = 0;
bno055_get_intmsk_gyro_anymotion(&gyro_am);
bno055_get_intmsk_gyro_highrate(&gyro_hr);
bno055_get_intmsk_accel_high_g(&accel_hg);
bno055_get_intmsk_accel_anymotion(&accel_am);
bno055_get_intmsk_accel_nomotion(&accel_nm);
Serial.println( "---- Interrupt bitmask ----" );
Serial.print( "Gyro Any Motion " );
Serial.println( gyro_am );
Serial.print( "Gyro High Rate " );
Serial.println( gyro_hr );
Serial.print( "Accel High G " );
Serial.println( accel_hg );
Serial.print( "Accel Any Motion " );
Serial.println( accel_am );
Serial.print( "Accel No Motion " );
Serial.println( accel_nm );
Serial.println( "---------------------------" );
}
/*
* Print the interrupt status
*/
void getInterruptStatusEnabled(bool synthetic) {
// 37 INT_STA 0x00 ACC_NM ACC_AM ACC_HIGH_G GYR_DRDY GYR_HIGH_RATE GYRO_AM MAG_DRDY ACC_BSX_DRDY
#pragma pack(push, 1)
struct StatusInterrupt {
byte accel_bsx_drdy : 1;
byte mag_drdy : 1;
byte gyro_am : 1;
byte gyro_high_rate : 1;
byte gyro_drdy : 1;
byte accel_high_g : 1;
byte accel_am : 1;
byte accel_nm : 1;
};
#pragma pack(pop)
StatusInterrupt registerByte = {0};
// Read all tht INT_STA addr to get all interrupt status in one read
// after the read the register is cleared
BNO055_RETURN_FUNCTION_TYPE res = bno055_read_register(BNO055_INT_STA_ADDR, (unsigned char *)(& registerByte), 1);
if (res == 0) {
if (synthetic){
Serial.print("Anm Aam Ahg Grd Ggr Gam Mrd Abr --> ");
Serial.print( registerByte.accel_nm );
Serial.print( registerByte.accel_am );
Serial.print( registerByte.accel_high_g );
Serial.print( registerByte.gyro_drdy );
Serial.print( registerByte.gyro_high_rate );
Serial.print( registerByte.gyro_am );
Serial.print( registerByte.mag_drdy );
Serial.println( registerByte.accel_bsx_drdy );
}else{
Serial.print( "Gyro Any Motion " );
Serial.println( registerByte.gyro_am );
Serial.print( "Gyro High Rate " );
Serial.println( registerByte.gyro_high_rate );
Serial.print( "Accel High G " );
Serial.println( registerByte.accel_high_g );
Serial.print( "Accel Any Motion " );
Serial.println( registerByte.accel_am );
Serial.print( "Accel No Motion " );
Serial.println( registerByte.accel_nm );
}
} else {
Serial.println("Error status!");
}
}
void printSlowMotionNoMotionParameters(){
unsigned char accel_slow_no_thr = 0;
bno055_get_accel_slow_no_threshold(&accel_slow_no_thr);
Serial.print("Slow motion/no motion threshold: ");
Serial.println(accel_slow_no_thr);
unsigned char accel_am_dur = 0;
bno055_get_accel_anymotion_duration(&accel_am_dur);
Serial.print("Slow motion/no motion duration: ");
Serial.println(accel_am_dur);
}
Here is the Serial output; the interrupt enabled on POWER_MODE_LOW_POWER are Any motion and No Motion as written in the paragraph that describes low power mode.
---- Interrupt enabled ----
Gyro Any Motion 0
Gyro High Rate 0
Accel High G 0
Accel Any Motion 1
Accel No Motion 1
---------------------------
---- Interrupt bitmask ----
Gyro Any Motion 0
Gyro High Rate 0
Accel High G 0
Accel Any Motion 0
Accel No Motion 0
---------------------------
Slow motion/no motion threshold: 10
Slow motion/no motion duration: 3
2Secs - Orientation: 168.00, 363.25, 354.19 - Anm Aam Ahg Grd Ggr Gam Mrd Abr --> 00000000
3Secs - Orientation: 168.00, 363.19, 354.12 - Anm Aam Ahg Grd Ggr Gam Mrd Abr --> 00000000
4Secs - Orientation: 168.00, 363.19, 354.12 - Anm Aam Ahg Grd Ggr Gam Mrd Abr --> 00000000
5Secs - Orientation: 173.62, 363.00, 353.94 - Anm Aam Ahg Grd Ggr Gam Mrd Abr --> 01000000
6Secs - Orientation: 163.19, 362.94, 353.25 - Anm Aam Ahg Grd Ggr Gam Mrd Abr --> 01000000
7Secs - Orientation: 163.19, 362.94, 353.25 - Anm Aam Ahg Grd Ggr Gam Mrd Abr --> 00000000
8Secs - Orientation: 163.19, 362.94, 353.25 - Anm Aam Ahg Grd Ggr Gam Mrd Abr --> 00000000
9Secs - Orientation: 163.19, 362.94, 353.25 - Anm Aam Ahg Grd Ggr Gam Mrd Abr --> 00000000
10Secs - Orientation: 163.19, 362.94, 353.25 - Anm Aam Ahg Grd Ggr Gam Mrd Abr --> 00000000
11Secs - Orientation: 163.19, 362.94, 353.25 - Anm Aam Ahg Grd Ggr Gam Mrd Abr --> 00000000
12Secs - Orientation: 163.19, 362.94, 353.25 - Anm Aam Ahg Grd Ggr Gam Mrd Abr --> 00000000
13Secs - Orientation: 163.19, 362.94, 353.25 - Anm Aam Ahg Grd Ggr Gam Mrd Abr --> 10000000
14Secs - Orientation: 163.19, 362.94, 353.25 - Anm Aam Ahg Grd Ggr Gam Mrd Abr --> 00000000
15Secs - Orientation: 163.19, 362.94, 353.25 - Anm Aam Ahg Grd Ggr Gam Mrd Abr --> 00000000
16Secs - Orientation: 163.19, 362.94, 353.25 - Anm Aam Ahg Grd Ggr Gam Mrd Abr --> 00000000
When I shake the bno055, It raises the pin of Any motion 2 times,
Anm Aam Ahg Grd Ggr Gam Mrd Abr --> 01000000
then I stop moving It, and after 6secs, no motion interrupt is raised.
Anm Aam Ahg Grd Ggr Gam Mrd Abr --> 10000000
The sketch was very simple, but as you can see, I must redò the function that read the status of interrupt, the implementation of the library
bno055_get_interrupt_status_gyro_anymotion(&gyr_anymotion);
bno055_get_interrupt_status_gyro_highrate(&gyr_highrate);
bno055_get_interrupt_status_accel_highg(&acc_highg);
bno055_get_interrupt_status_accel_anymotion(&acc_anymotion);
bno055_get_interrupt_status_accel_nomotion(&acc_nomotion);
It doesn’t turn out very efficient because you can only read an interrupt parameter; after the first read of the register, all data are cleared, and you cannot know if other interrupts are raised, so I get all bits in one read, and then I print the status of all interrupts.
Thanks
- BNO055 accelerometer, gyroscope, magnetometer with basic Adafruit library
- BNO055 for esp32, esp8266, and Arduino: wiring and advanced Bosch library
- BNO055 for esp32, esp8266, and Arduino: features, configuration, and axes remap
- BNO055: power modes, accelerometer, and motion interrupt
- BNO055 for esp32, esp8266, and Arduino: enable INT pin and accelerometer High G Interrupt
- BNO055 for esp32, esp8266, and Arduino: Gyroscope High Rate and Any Motion Interrupt
I get an error while trying your example ( interupt )
src\imuInterruptGyro.cpp:234:85: error: invalid cast from type ‘getInterruptStatusEnabled(bool)::StatusInterrupt’ to type ‘unsigned char*’
234 | BNO055_RETURN_FUNCTION_TYPE res = bno055_read_register(BNO055_INT_STA_ADDR, (unsigned char *)(registerByte), 1);
Hi,
yes, there was a copy/paste error, now I fixed it.
Thanks Renzo
Thank you for the speedy reply.
in the last section(6) there is still the same mistake.
Also in line90 of the “anymotion for gyro” the Z axis is not enabled ( twice the Y axis )
Hi i m using your second sketch to put BNO055 to LowPower mode and it s working fine but after shaking there is no signal on interupt pin i want to use it for wake up esp32 do you know where mistake can be ?
Hi Adam,
you can find the information you need in the next article.
BNO055 for esp32, esp8266 and Arduino: enable INT pin and accelerometer High G Interrupt – 5
Bye Renzo