EByte LoRa E32 & MicroPython: a deep dive into transmission types – 4
In our continuous journey to unveil the intricate layers of IoT technology, we shift our focus to a key aspect of interconnected systems: data transmission.
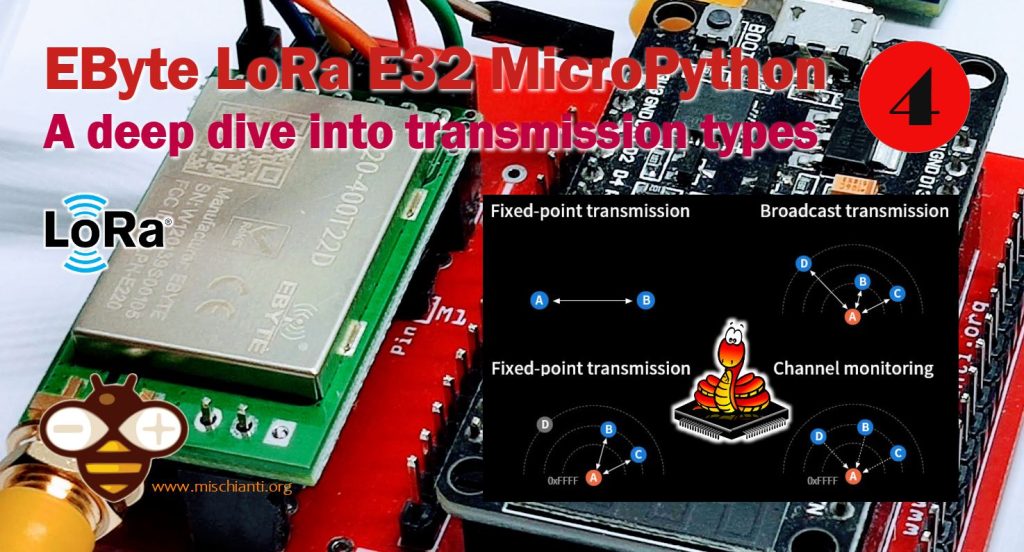
Data transmission forms the circulatory system of any IoT configuration, delivering information from one node to another. In this context, we bring into the limelight two powerful tools – the EByte’s LoRa E32 modules and the MicroPython programming language.
This article, the fourth in our series, provides an in-depth analysis of the different transmission types that can be realized with the EByte LoRa E32 module utilizing MicroPython. By understanding these transmission modalities, developers can effectively harness the power of these tools and tailor their applications to meet specific needs.
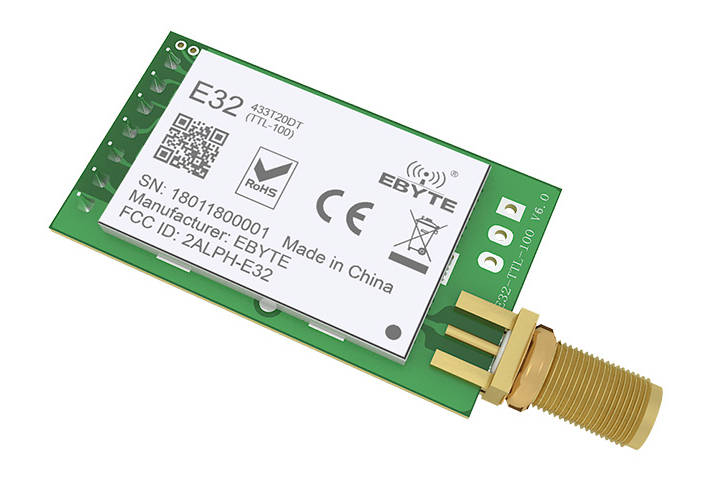
Here my selection of modules AliExpress (433MHz 5Km) - AliExpress (433MHz 8Km) - AliExpress (433MHz 16Km) - AliExpress (868MHz 915MHz 5.5Km) - AliExpress (868MHz 915MHz 8Km)
In the first part, we’ve used a transparent transmission, so we send to all and receive from all with the same address and channel.
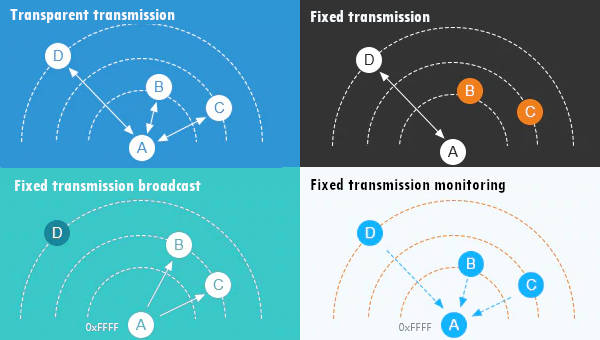
But It isn’t a standard scenario, and we usually want to send it to a specified point and receive a response.
If you have trouble with the device’s freeze, you must put a pull-up 4.7k resistor or better connect to the device’s AUX pin.
Connection schemas
For basic usage, we used this configuration. Still, you are working only in “Normal mode” in this configuration, and we will manage only the needed pin dynamic (RX, TX) to simplify the process.
Normal configuration (transparent)
esp32
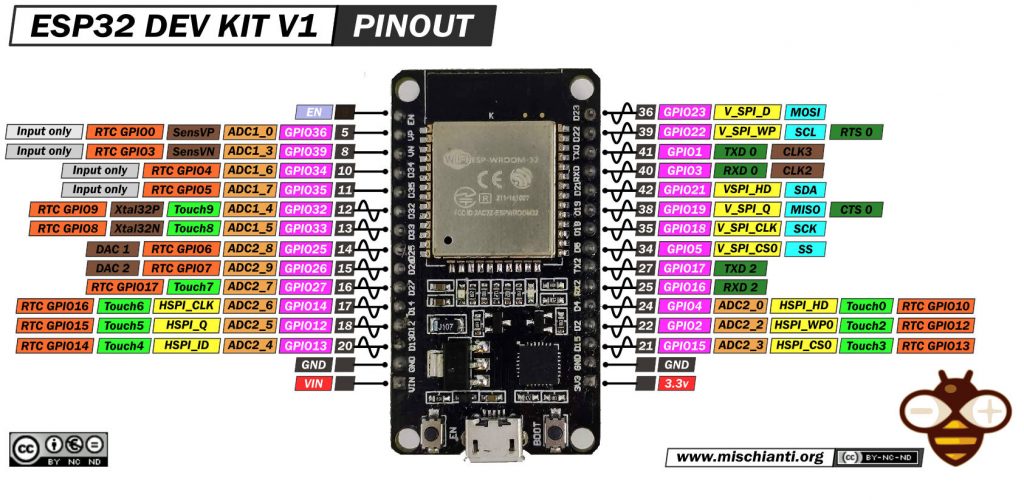
Here my selection of esp32 ESP32 Dev Kit v1 - TTGO T-Display 1.14 ESP32 - NodeMCU V3 V2 ESP8266 Lolin32 - NodeMCU ESP-32S - WeMos Lolin32 - WeMos Lolin32 mini - ESP32-CAM programmer - ESP32-CAM bundle - ESP32-WROOM-32 - ESP32-S
The wiring diagram is quite simple, and for now, I put M0 and M1 directly to GND for the test.
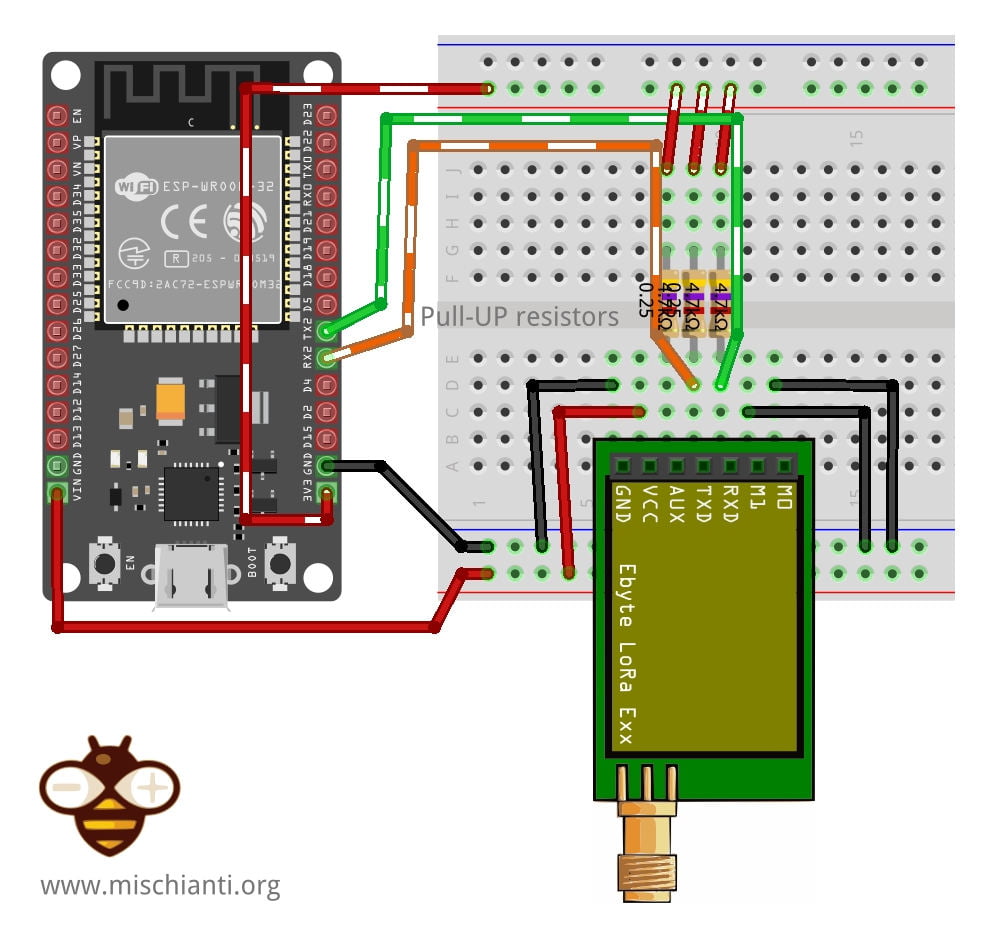
E32 | esp32 |
---|---|
M0 | GND (Set normal mode) |
M1 | GND (Set normal mode) |
RX | TX2 (PullUP 4,7KΩ) |
TX | RX2 (PullUP 4,7KΩ) |
AUX | Not connected (better if you set a pin, but not needed for this test) |
VCC | 5v |
GND | GND |
Raspberry Pi Pico
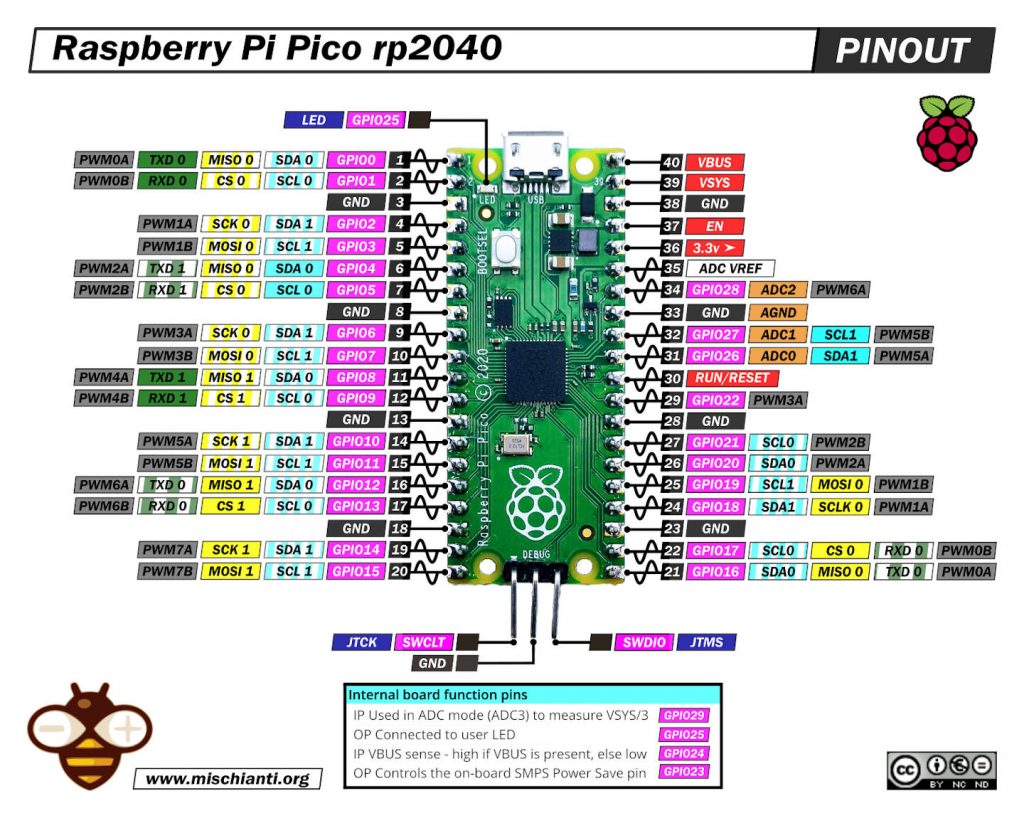
And here is the connection diagram, for this test, you can remove the AUX pin connection. You can also see that I use a different default Serial port because It differs from the Arduino environment.
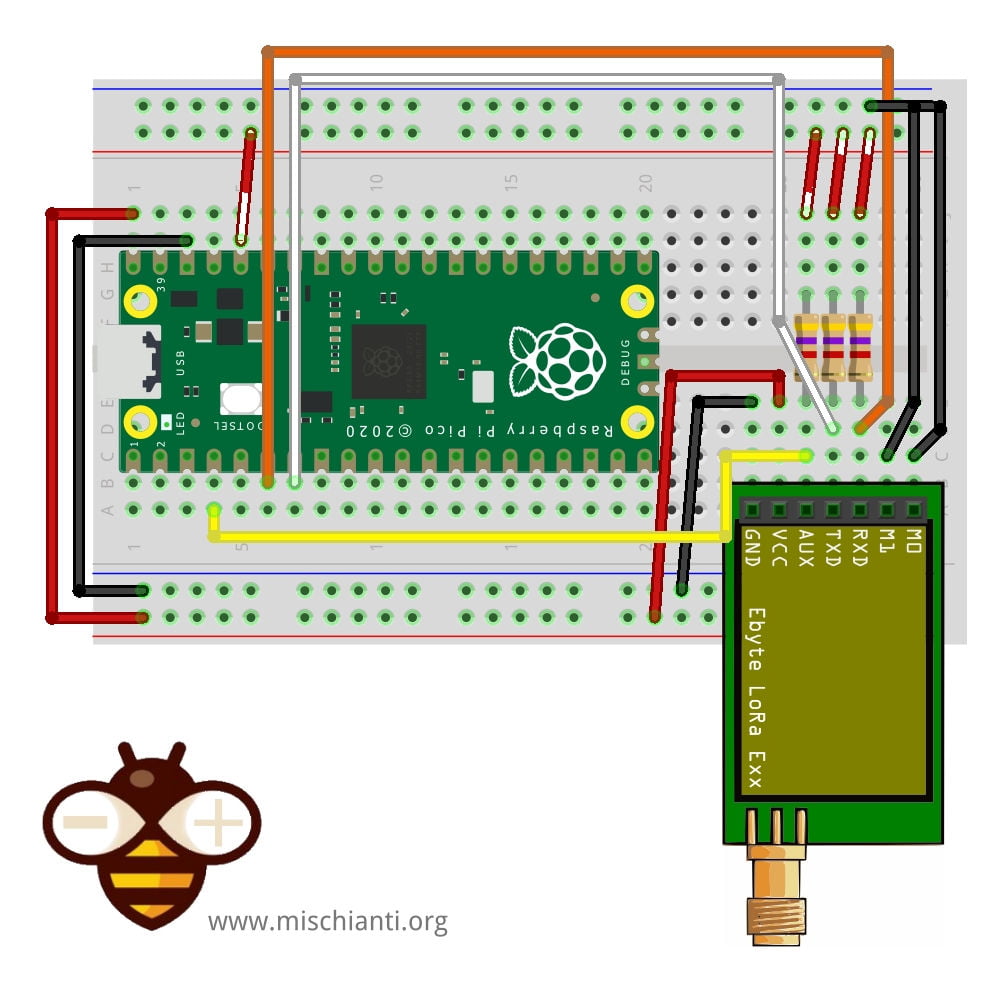
Pay attention UART(1) use different pin in MicroPython than Arduino environment Serial1
E32 | esp32 |
---|---|
M0 | GND (Set normal mode) |
M1 | GND (Set normal mode) |
RX | TX1 GPIO4 (PullUP 4,7KΩ) |
TX | RX1 GPIO5 (PullUP 4,7KΩ) |
AUX | 2 (better if you set a pin, but not needed for this test) |
VCC | 5v |
GND | GND |
For the Arduino standard pinout, you must change the UART declaration so uart2 = UART(1, rx=Pin(9), tx=Pin(8))
Transparent transmission
Transparent transmission is a mode of data transmission where data is sent and received without any filtering based on the address. This means that the data sent by the transmitting device is available to be received by any device within its range, without consideration for the unique address of the receiving device.
In the context of the EByte LoRa E32 module, when operating in transparent transmission mode, the module sends out data that can be picked up by all other E32 devices within its range. This is akin to a broadcast, where the same message is sent out to all devices in a particular area.
This mode of transmission is particularly useful when there is a need to disseminate the same piece of information to multiple devices at the same time. For example, in an IoT application like a weather monitoring system, a sensor node could use transparent transmission to send weather data to all other nodes in the network simultaneously.
However, it’s important to note that while transparent transmission can be effective for broadcasting information, it may not be suitable for situations that require secure or targeted data exchange as it lacks the specificity of address-based communication.
Send a string message
# Author: Renzo Mischianti
# Website: www.mischianti.org
#
# Description:
# This script demonstrates how to use the E32 LoRa module with MicroPython.
# Sending string
#
# Note: This code was written and tested using MicroPython on an ESP32 board.
# It works with other boards, but you may need to change the UART pins.
from lora_e32 import LoRaE32, Configuration
from machine import UART
from lora_e32_operation_constant import ResponseStatusCode
# Create a UART object to communicate with the LoRa module with ESP32
uart2 = UART(2)
# Create a LoRaE32 object, passing the UART object and pin configurations
lora = LoRaE32('433T20D', uart2, aux_pin=15, m0_pin=21, m1_pin=19)
# Create a UART object to communicate with the LoRa module with Raspberry Pi Pico
# uart2 = UART(1)
# Use the Serial1 pins of Arduino env on the Raspberry Pi Pico
# uart2 = UART(1, rx=Pin(9), tx=Pin(8))
# lora = LoRaE32('433T20D', uart2, aux_pin=2, m0_pin=10, m1_pin=11)
code = lora.begin()
print("Initialization: {}", ResponseStatusCode.get_description(code))
# Set the configuration to default values and print the updated configuration to the console
# Not needed if already configured
# configuration_to_set = Configuration('433T20D')
# code, confSetted = lora.set_configuration(configuration_to_set)
# print("Set configuration: {}", ResponseStatusCode.get_description(code))
# Send a string message (transparent)
message = 'Hello, world!'
code = lora.send_transparent_message(message)
print("Send message: {}", ResponseStatusCode.get_description(code))
If you have already changed the configuration, you must restore the base parameter:
configuration_to_set = Configuration('433T20D')
code, confSetted = lora.set_configuration(configuration_to_set)
print("Set configuration: {}", ResponseStatusCode.get_description(code))
Send a dictionary
# Author: Renzo Mischianti
# Website: www.mischianti.org
#
# Description:
# This script demonstrates how to use the E32 LoRa module with MicroPython.
# Sending dictionary
#
# Note: This code was written and tested using MicroPython on an ESP32 board.
# It works with other boards, but you may need to change the UART pins.
from lora_e32 import LoRaE32, Configuration
from machine import UART
from lora_e32_operation_constant import ResponseStatusCode
# Create a UART object to communicate with the LoRa module with ESP32
uart2 = UART(2)
# Create a LoRaE32 object, passing the UART object and pin configurations
lora = LoRaE32('433T20D', uart2, aux_pin=15, m0_pin=21, m1_pin=19)
# Create a UART object to communicate with the LoRa module with Raspberry Pi Pico
# uart2 = UART(1)
# Use the Serial1 pins of Arduino env on the Raspberry Pi Pico
# uart2 = UART(1, rx=Pin(9), tx=Pin(8))
# lora = LoRaE32('433T20D', uart2, aux_pin=2, m0_pin=10, m1_pin=11)
code = lora.begin()
print("Initialization: {}", ResponseStatusCode.get_description(code))
# Set the configuration to default values and print the updated configuration to the console
# Not needed if already configured
# configuration_to_set = Configuration('433T20D')
# code, confSetted = lora.set_configuration(configuration_to_set)
# print("Set configuration: {}", ResponseStatusCode.get_description(code))
# Send a dictionary message (transparent)
data = {'key1': 'value1', 'key2': 'value2'}
code = lora.send_transparent_dict(data)
print("Send message: {}", ResponseStatusCode.get_description(code))
Fixed transmission
Fixed transmission is a mode of data transmission that uses a specific device’s hardware address for communication. This means that the transmitting device sends data specifically to a receiving device with a known address. It’s akin to sending a letter through the mail where the sender writes the recipient’s address on the envelope to ensure that it reaches the intended destination.
In the context of the EByte LoRa E32 module, when operating in fixed transmission mode, the module sends data specifically to another E32 module with a designated address. This means that even if there are other E32 modules within range, only the one with the specific address will receive the data.
In essence, fixed transmission provides a more secure and targeted method of communication compared to modes like transparent transmission, as it ensures that only the intended recipient receives the transmitted data.
Fixed transmission: point to point
This transmission mode is particularly useful when there is a need to establish secure, one-to-one communication between two devices. For instance, in an IoT application such as a home security system, a door sensor might use the fixed transmission to send alarm data specifically to the main security system control panel.
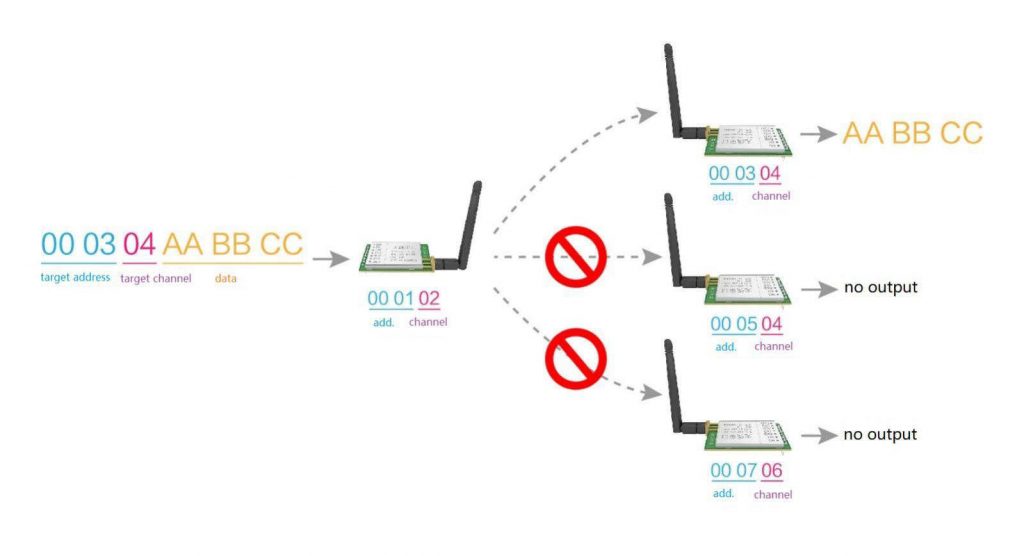
So first, we must set M0 and M1 pin to HIGH to enter on program/sleep mode and set correct address and fixed transmission flag.
If we want to replicate the condition of the sender in the upper image, we must do this configuration.
# Set the configuration to default values and print the updated configuration to the console
# Not needed if already configured
configuration_to_set = Configuration('433T20D')
configuration_to_set.ADDL = 0x02 # Address of this sender no receiver
configuration_to_set.OPTION.fixedTransmission = FixedTransmission.FIXED_TRANSMISSION
code, confSetted = lora.set_configuration(configuration_to_set)
print("Set configuration: {}", ResponseStatusCode.get_description(code))
Then for the receiver device, we must set this configuration.
# Set the configuration to default values and print the updated configuration to the console
# Not needed if already configured
configuration_to_set = Configuration('433T20D')
configuration_to_set.ADDL = 0x01 # Address of this receive no sender
configuration_to_set.OPTION.fixedTransmission = FixedTransmission.FIXED_TRANSMISSION
code, confSetted = lora.set_configuration(configuration_to_set)
print("Set configuration: {}", ResponseStatusCode.get_description(code))
Now we just sent a message to the specified device.
# Send a string message (fixed)
message = 'Hello, world!'
code = lora.send_fixed_message(0, 0x01, 23, message)
The receiver is more transparent because the device manages the address and channel.
print("Waiting for messages...")
while True:
if lora.available() > 0:
code, value = lora.receive_message()
print(ResponseStatusCode.get_description(code))
print(value)
utime.sleep_ms(2000)
So, you can check that no data has arrived.
Send sketch (If you don’t attach all pins first, you must put on program mode and then restore normal mode):
# Author: Renzo Mischianti
# Website: www.mischianti.org
#
# Description:
# This script demonstrates how to use the E32 LoRa module with MicroPython.
# Sending string to a specified address (receiver)
# ADDH = 0x00
# ADDL = 0x02
# CHAN = 23
#
# Note: This code was written and tested using MicroPython on an ESP32 board.
# It works with other boards, but you may need to change the UART pins.
from lora_e32 import LoRaE32, Configuration
from machine import UART
from lora_e32_constants import FixedTransmission
from lora_e32_operation_constant import ResponseStatusCode
# Create a UART object to communicate with the LoRa module with ESP32
uart2 = UART(2)
# Create a LoRaE32 object, passing the UART object and pin configurations
lora = LoRaE32('433T20D', uart2, aux_pin=15, m0_pin=21, m1_pin=19)
# Create a UART object to communicate with the LoRa module with Raspberry Pi Pico
# uart2 = UART(1)
# Use the Serial1 pins of Arduino env on the Raspberry Pi Pico
# uart2 = UART(1, rx=Pin(9), tx=Pin(8))
# lora = LoRaE32('433T20D', uart2, aux_pin=2, m0_pin=10, m1_pin=11)
code = lora.begin()
print("Initialization: {}", ResponseStatusCode.get_description(code))
# Set the configuration to default values and print the updated configuration to the console
# Not needed if already configured
configuration_to_set = Configuration('433T20D')
configuration_to_set.ADDL = 0x02 # Address of this sender no receiver
configuration_to_set.OPTION.fixedTransmission = FixedTransmission.FIXED_TRANSMISSION
code, confSetted = lora.set_configuration(configuration_to_set)
print("Set configuration: {}", ResponseStatusCode.get_description(code))
# Send a string message (fixed)
message = 'Hello, world!'
code = lora.send_fixed_message(0, 0x01, 23, message)
# The receiver must be configured with ADDH = 0x00, ADDL = 0x01, CHAN = 23
print("Send message: {}", ResponseStatusCode.get_description(code))
Receiver code (If you don’t attach all pins first, you must put on program mode than reset in normal mode) :
# Author: Renzo Mischianti
# Website: www.mischianti.org
#
# Description:
# This script demonstrates how to use the E32 LoRa module with MicroPython.
# It includes examples of sending and receiving string using both transparent and fixed transmission modes.
# The code also configures the module's address and channel for fixed transmission mode.
# Address and channel of this receiver:
# ADDH = 0x00
# ADDL = 0x01
# CHAN = 23
#
# Can be used with the send_fixed_string and send_transparent_string scripts
#
# Note: This code was written and tested using MicroPython on an ESP32 board.
# It works with other boards, but you may need to change the UART pins.
from lora_e32 import LoRaE32, Configuration
from machine import UART
import utime
from lora_e32_constants import FixedTransmission
from lora_e32_operation_constant import ResponseStatusCode
# Create a UART object to communicate with the LoRa module with ESP32
uart2 = UART(2)
# Create a LoRaE32 object, passing the UART object and pin configurations
lora = LoRaE32('433T20D', uart2, aux_pin=15, m0_pin=21, m1_pin=19)
# Create a UART object to communicate with the LoRa module with Raspberry Pi Pico
# uart2 = UART(1)
# Use the Serial1 pins of Arduino env on the Raspberry Pi Pico
# uart2 = UART(1, rx=Pin(9), tx=Pin(8))
# lora = LoRaE32('433T20D', uart2, aux_pin=2, m0_pin=10, m1_pin=11)
code = lora.begin()
print("Initialization: {}", ResponseStatusCode.get_description(code))
# Set the configuration to default values and print the updated configuration to the console
# Not needed if already configured
configuration_to_set = Configuration('433T20D')
configuration_to_set.ADDL = 0x01 # Address of this receive no sender
configuration_to_set.OPTION.fixedTransmission = FixedTransmission.FIXED_TRANSMISSION
code, confSetted = lora.set_configuration(configuration_to_set)
print("Set configuration: {}", ResponseStatusCode.get_description(code))
print("Waiting for messages...")
while True:
if lora.available() > 0:
code, value = lora.receive_message()
print(ResponseStatusCode.get_description(code))
print(value)
utime.sleep_ms(2000)
If you change the address or channel to the receiver sketch, you no longer receive messages.
Fixed transmission: broadcast
In light of this, Fixed Transmission: Broadcast is a specialized mode of transmission in which data is specifically broadcast from one device to all others within its range, similar to Transparent Transmission. However, the crucial difference lies in the level of control over the recipient of the message.
In Fixed Transmission: Broadcast, although the data is being sent to all devices within range, the sender can specifically determine and control the recipient by using the unique hardware address. In contrast, Transparent Transmission broadcasts data to all devices within range without any filtering or control over the recipient.
This distinction provides an additional layer of flexibility to the sender in Fixed Transmission: Broadcast, allowing it to direct the transmission to a specific device, even when broadcasting to all devices in range. This is not possible in Transparent Transmission, which can only broadcast to all devices without any control over the recipient.
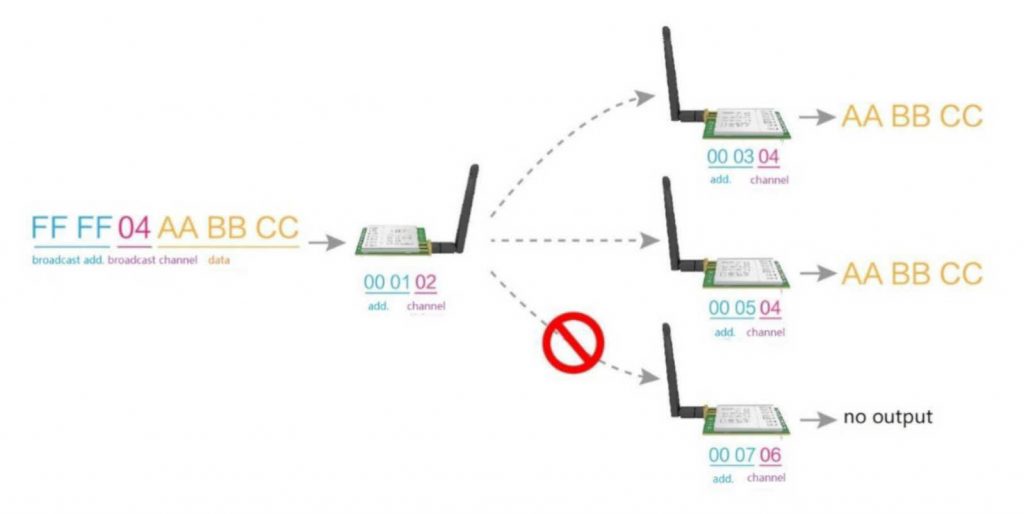
With my library, you can use this transmission method.
message = 'Hello, world!'
code = lora.send_broadcast_message(23, message)
print("Send message: {}", ResponseStatusCode.get_description(code))
The receiver, as described, has the same code because the device manages the preamble with Address and Channel.
Here is the sender sketch (If you don’t attach all pins first, you must put on program mode then reset in normal mode) :
# Author: Renzo Mischianti
# Website: www.mischianti.org
#
# Description:
# This script demonstrates how to use the E32 LoRa module with MicroPython.
# Sending string to all address of the same channel we are using
#
# Note: This code was written and tested using MicroPython on an ESP32 board.
# It works with other boards, but you may need to change the UART pins.
from lora_e32 import LoRaE32, Configuration
from machine import UART
from lora_e32_constants import FixedTransmission
from lora_e32_operation_constant import ResponseStatusCode
# Initialize the LoRaE32 module
uart2 = UART(2)
lora = LoRaE32('433T20D', uart2, aux_pin=15, m0_pin=19, m1_pin=21)
code = lora.begin()
print("Initialization: {}", ResponseStatusCode.get_description(code))
# Set the configuration to default values and print the updated configuration to the console
# Not needed if already configured
# configuration_to_set = Configuration('433T20D')
# configuration_to_set.ADDL = 0x02 # Address of this sender no receiver
# configuration_to_set.OPTION.fixedTransmission = FixedTransmission.FIXED_TRANSMISSION
# code, confSetted = lora.set_configuration(configuration_to_set)
# print("Set configuration: {}", ResponseStatusCode.get_description(code))
# Send a string message (fixed)
message = 'Hello, world!'
code = lora.send_broadcast_message(23, message)
# The receiver must be configured with ADDH = 0x00, ADDL = 0x01, CHAN = 23
print("Send message: {}", ResponseStatusCode.get_description(code))
Fixed transmission: monitoring
Fixed Transmission: Monitoring is a specialized mode of data transmission that provides one-way communication from a specific transmitting device to a designated receiver. This mode focuses on the constant surveillance and reporting of data from the sender to the receiver.
In the context of the EByte LoRa E32 module, when operating in the fixed transmission: monitoring mode, the module sends data that is intended for a specific E32 module (the monitor). This ensures a dedicated data stream from the transmitter to the monitor. Other devices within the range, even if capable of receiving the transmission, are essentially ignored.
This mode of transmission is particularly useful in scenarios where real-time monitoring and tracking of data is required from a particular device or sensor. For example, in an industrial IoT setting, a sensor placed in a critical machine might use fixed transmission: monitoring to send real-time data to a central monitoring system.
Overall, fixed transmission: monitoring provides a secure and uninterrupted line of communication from a transmitter to a designated receiver, making it ideal for applications that require dedicated, one-way data streaming for monitoring purposes.
You must set the ADDL and ADDH like so:
# Set the configuration to default values and print the updated configuration to the console
# Not needed if already configured
configuration_to_set = Configuration('433T20D')
# With BROADCASS ADDRESS we receive all message
configuration_to_set.ADDL = BROADCAST_ADDRESS
configuration_to_set.ADDH = BROADCAST_ADDRESS
configuration_to_set.OPTION.fixedTransmission = FixedTransmission.FIXED_TRANSMISSION
code, confSetted = lora.set_configuration(configuration_to_set)
print("Set configuration: {}", ResponseStatusCode.get_description(code))
In the beginning, you receive all messages in a specified CHANNEL.
And the received message becomes (If you don’t attach all pins first, you must put on program mode then reset in normal mode) :
# Author: Renzo Mischianti
# Website: www.mischianti.org
#
# Description:
# This script demonstrates how to use the E32 LoRa module with MicroPython.
# Receiving string from all address by setting BROADCAST ADDRESS
#
# Note: This code was written and tested using MicroPython on an ESP32 board.
# It works with other boards, but you may need to change the UART pins.
from lora_e32 import LoRaE32, Configuration, BROADCAST_ADDRESS
from machine import UART
import utime
from lora_e32_constants import FixedTransmission
from lora_e32_operation_constant import ResponseStatusCode
# Initialize the LoRaE32 module
uart2 = UART(2)
lora = LoRaE32('433T20D', uart2, aux_pin=15, m0_pin=19, m1_pin=21)
code = lora.begin()
print("Initialization: {}", ResponseStatusCode.get_description(code))
# Set the configuration to default values and print the updated configuration to the console
# Not needed if already configured
configuration_to_set = Configuration('433T20D')
# With BROADCASS ADDRESS we receive all message
configuration_to_set.ADDL = BROADCAST_ADDRESS
configuration_to_set.ADDH = BROADCAST_ADDRESS
configuration_to_set.OPTION.fixedTransmission = FixedTransmission.FIXED_TRANSMISSION
code, confSetted = lora.set_configuration(configuration_to_set)
print("Set configuration: {}", ResponseStatusCode.get_description(code))
print("Waiting for messages...")
while True:
if lora.available() > 0:
code, value = lora.receive_message()
print(ResponseStatusCode.get_description(code))
print(value)
utime.sleep_ms(2000)
Thanks
- EByte LoRa E32 & MicroPython: specifications, overview and first use
- EByte LoRa E32 & MicroPython: exploring MicroPython library
- EByte LoRa E32 & MicroPython: detailed look at the configuration
- EByte LoRa E32 & MicroPython: a deep dive into transmission types