ESP32: flash compiled firmware and filesystem (.bin) with GUI tools – 2
The ESP32 boasts a crucial capability that streamlines the sharing of firmware, which most manufacturers widely utilize. This capability involves creating a pre-compiled binary file containing the sketch portion (or filesystem).
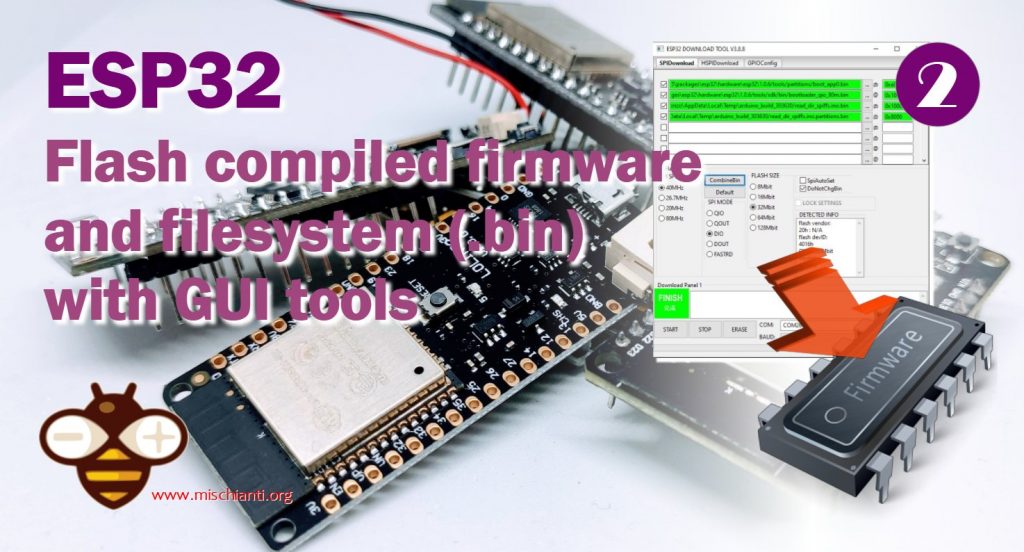
You can compile and generate source code for your device, but you can generate a binary file for the filesystem, also.
Espressif offers a GUI tool also to simplify the upload process.
Here some common esp32 device I use ESP32 Dev Kit v1 - TTGO T-Display 1.14 ESP32 - NodeMCU V3 V2 ESP8266 Lolin32 - NodeMCU ESP-32S - WeMos Lolin32 - WeMos Lolin32 mini - ESP32-CAM programmer - ESP32-CAM bundle - ESP32-WROOM-32 - ESP32-S
Basic tools
First, we look that the core component of ESP32 core needs python installed, and when installing It remember to add to the path (for windows)
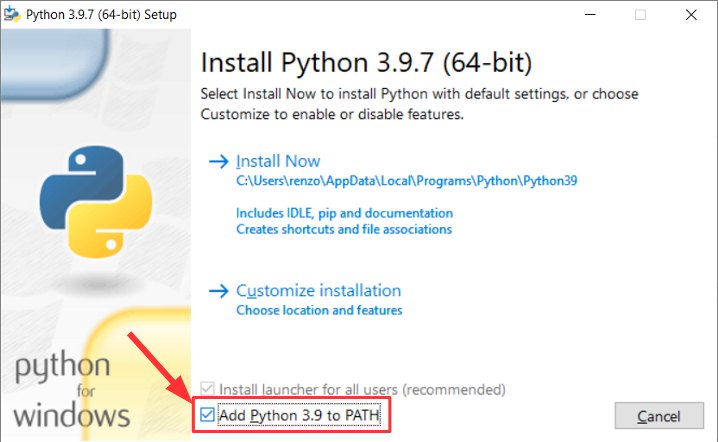
upload.py or esptool.py
In your installation of Arduino IDE, you can find upload.py, the file that is the same of esptool.py
.
You can install only esptool.py
for python with a simple command
pip install esptool
or you can download from here the compiled version; remember to add the executable to the path.
Flash with Espressif download tool
The same operation (after installing python and esptool) can be done with the Espressif Download Tool, which you can download from here.
Export the sketch binary only
To use the exported binary file, you must remember the address 0x10000, which identifies the writing address of the firmware.
You must link the bin file (check to the left), set the correct address and select the specs of your microcontroller.
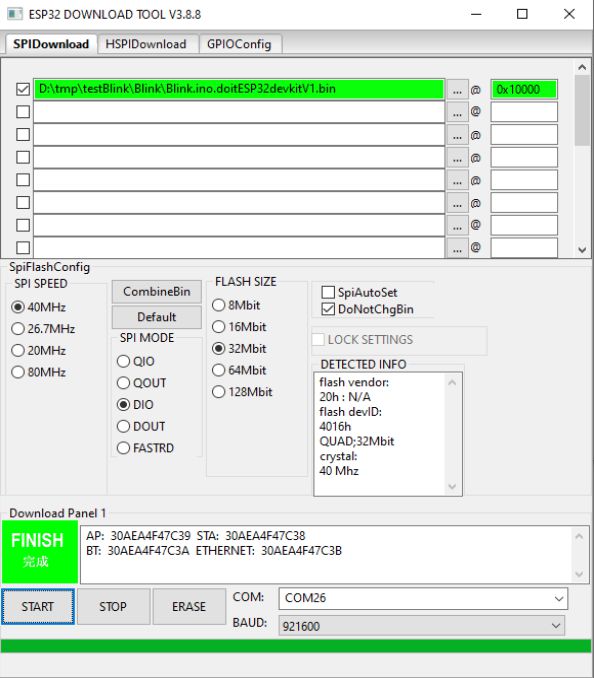
Compiled and filesystem binary
To learn more about the ESP32 filesystem, It’s important that you read
- ESP32: integrated SPIFFS FileSystem
- ESP32: integrated LittleFS FileSystem
- ESP32: integrated FFat (FAT/exFAT) FileSystem
We are going to do a test. I got a sketch from the tutorial “ESP32: integrated SPIFFS FileSystem“, and I created a data folder with file1.txt
and file2.txt
.
/*
* ESP32
* SPIFFS get info, read dir and show all file uploaded
* add a data folder to use with ESP32 Sketch data uploader
* by Mischianti Renzo <https://mischianti.org>
*
* https://mischianti.org
*
*/
#include "Arduino.h"
#include "SPIFFS.h"
void printDirectory(File dir, int numTabs = 3);
void setup()
{
Serial.begin(115200);
delay(500);
Serial.println(F("Inizializing FS..."));
if (SPIFFS.begin()){
Serial.println(F("done."));
}else{
Serial.println(F("fail."));
}
// To format all space in SPIFFS
// SPIFFS.format()
// Get all information of your SPIFFS
unsigned int totalBytes = SPIFFS.totalBytes();
unsigned int usedBytes = SPIFFS.usedBytes();
Serial.println("File sistem info.");
Serial.print("Total space: ");
Serial.print(totalBytes);
Serial.println("byte");
Serial.print("Total space used: ");
Serial.print(usedBytes);
Serial.println("byte");
Serial.println();
// Open dir folder
File dir = SPIFFS.open("/");
// Cycle all the content
printDirectory(dir);
}
void loop()
{
}
void printDirectory(File dir, int numTabs) {
while (true) {
File entry = dir.openNextFile();
if (! entry) {
// no more files
break;
}
for (uint8_t i = 0; i < numTabs; i++) {
Serial.print('\t');
}
Serial.print(entry.name());
if (entry.isDirectory()) {
Serial.println("/");
printDirectory(entry, numTabs + 1);
} else {
// files have sizes, directories do not
Serial.print("\t\t");
Serial.println(entry.size(), DEC);
}
entry.close();
}
}
This sketch gets the list of files and some SPIFFS information; here is the upload command from the console.
C:\Users\renzo\AppData\Local\Arduino15\packages\esp32\tools\esptool_py\3.0.0/esptool.exe --chip esp32 --port COM26 --baud 921600 --before default_reset --after hard_reset write_flash -z --flash_mode dio --flash_freq 80m --flash_size detect 0xe000 C:\Users\renzo\AppData\Local\Arduino15\packages\esp32\hardware\esp32\1.0.6/tools/partitions/boot_app0.bin 0x1000 C:\Users\renzo\AppData\Local\Arduino15\packages\esp32\hardware\esp32\1.0.6/tools/sdk/bin/bootloader_qio_80m.bin 0x10000 C:\Users\renzo\AppData\Local\Temp\arduino_build_303630/read_dir_spiffs.ino.bin 0x8000 C:\Users\renzo\AppData\Local\Temp\arduino_build_303630/read_dir_spiffs.ino.partitions.bin
and the uploading of SPIFFS,
[SPIFFS] data : D:\tmp\testSPIFFS\read_dir_spiffs\data
[SPIFFS] offset : 0
[SPIFFS] start : 1376256
[SPIFFS] size : 704
[SPIFFS] page : 256
[SPIFFS] block : 4096
->/file1.txt
->/file2.txt
[SPIFFS] upload : C:\Users\renzo\AppData\Local\Temp\arduino_build_303630/read_dir_spiffs.spiffs.bin
[SPIFFS] address: 1376256
[SPIFFS] port : COM26
[SPIFFS] speed : 921600
[SPIFFS] mode : dio
[SPIFFS] freq : 80m
Pay attention address 1376256 in HEX is 0x150000
the serial output is now
Inizializing FS...
done.
File sistem info.
Total space: 655361byte
Total space used: 1004byte
/file1.txt 5
/file2.txt 5
Now we change it a little the sketch:
Serial.println(F("Inizializing FS 2..."));
and I add file3.txt to spiffs, disconnected the device and regenerated all.
Pay attention LittleFS and SPIFFS have the same partition table, FFat no.
Now upload with Espressif Download Tool
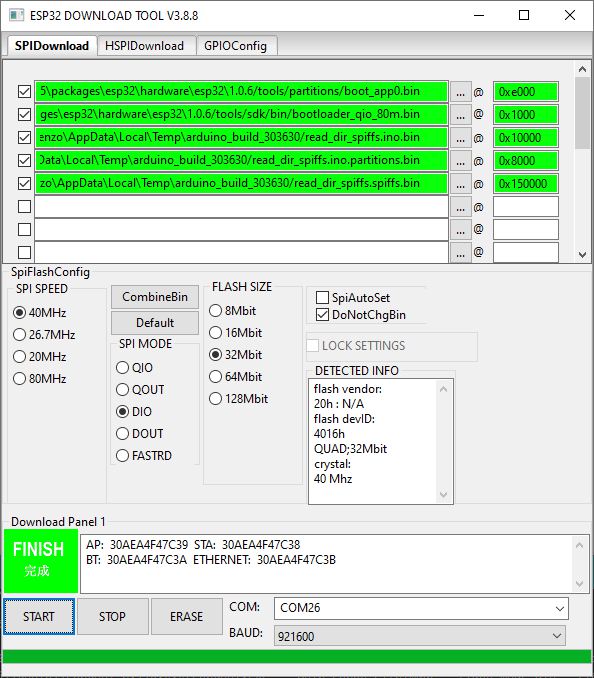
We can check that the sketch is updated.
Combine all binary in one file
Now we are going to combine all files. This generates a taget.bin
file, erases the microcontroller firmware with the tool, and we are going to upload the combined file.
Pay attention the start address for combined file is 0x0.
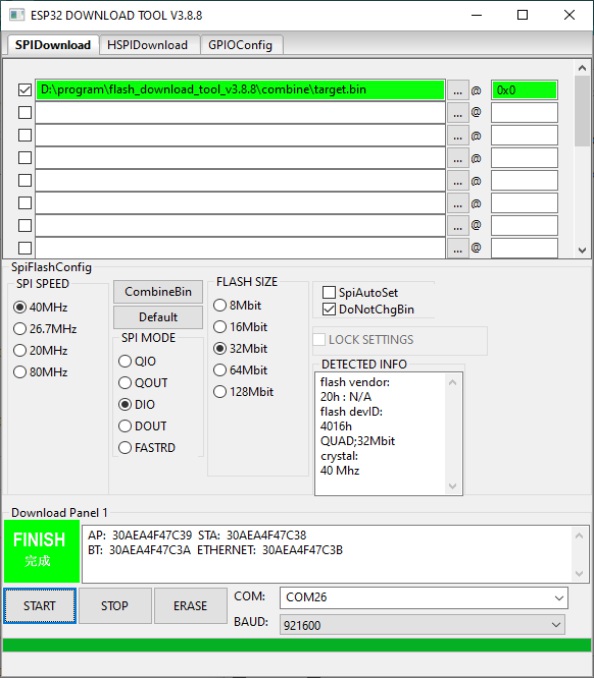
Thanks
- ESP32: pinout, specs and Arduino IDE configuration
- ESP32: integrated SPIFFS Filesystem
- ESP32: manage multiple Serial and logging
- ESP32 practical power saving
- ESP32 practical power saving: manage WiFi and CPU
- ESP32 practical power saving: modem and light sleep
- ESP32 practical power saving: deep sleep and hibernation
- ESP32 practical power saving: preserve data, timer and touch wake up
- ESP32 practical power saving: external and ULP wake up
- ESP32 practical power saving: UART and GPIO wake up
- ESP32: integrated LittleFS FileSystem
- ESP32: integrated FFat (Fat/exFAT) FileSystem
- ESP32-wroom-32
- ESP32-CAM
- ESP32: use ethernet w5500 with plain (HTTP) and SSL (HTTPS)
- ESP32: use ethernet enc28j60 with plain (HTTP) and SSL (HTTPS)
- How to use SD card with esp32
- esp32 and esp8266: FAT filesystem on external SPI flash memory
- Firmware and OTA update management
- Firmware management
- OTA update with Arduino IDE
- OTA update with Web Browser
- Self OTA uptate from HTTP server
- Non-standard Firmware update
- Integrating LAN8720 with ESP32 for Ethernet Connectivity with plain (HTTP) and SSL (HTTPS)
- Connecting the EByte E70 to ESP32 c3/s3 devices and a simple sketch example
- ESP32-C3: pinout, specs and Arduino IDE configuration
I made many test to create an export apps using your solution to export app.
It work fine if no file system is used. I implement the LittleFs in my projets.
The informations about this second phase is not clear for me.
Do you have a post link to share details about my projets?
Hi Alain,
you can use the forum.
Bye Renzo
Hi Renzo,
thk for suggestion.
I will later
and nice application .
My need is exact the same in the forum. I wlll work on my side to do it: include LittelFs in export app.