FTP server on STM32 with w5500, enc28j60, SD Card, and SPI Flash
Finally, I’m going to upgrade the SimpleFTPServer with complete and tested support for STM32 devices. For the test, I use a w5500 device and an enc28j60. For the storage, I’m going to use a simple SD card adapter and external SPI Flash.
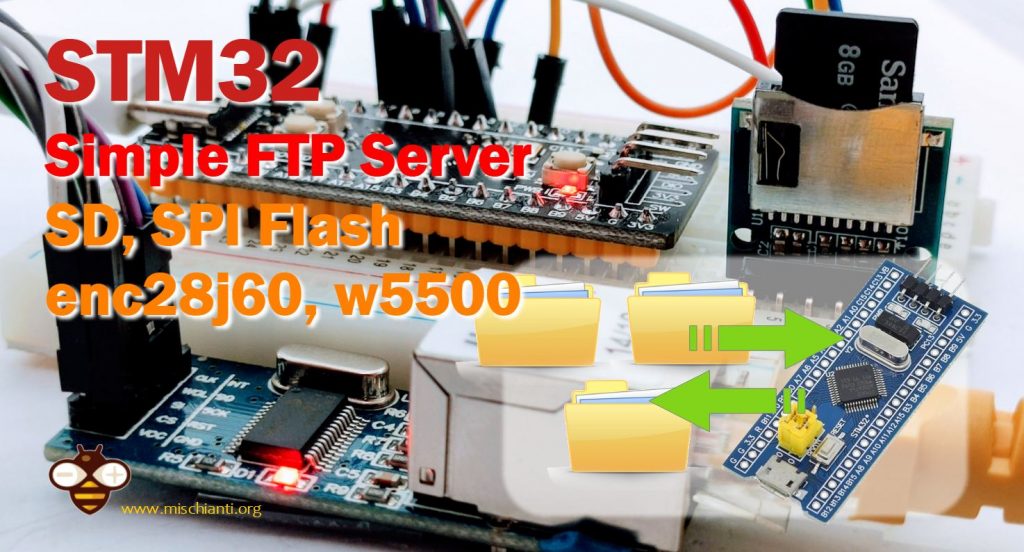
You can refer to the article “How to use SD card with stm32 and SdFat library” for the SD wiring and configuration, the “STM32: SPI flash memory FAT FS” for the SPI Flash, and you can refer “STM32: ethernet w5500 with plain (HTTP) and SSL (HTTPS)” and “STM32: ethernet enc28j60 with plain (HTTP) and SSL (HTTPS)” for the Ethernet devices configuration.
Here a selection of STM32 STM32F103C8T6 STM32F401 STM32F411 ST-Link v2 ST-Link v2 official
Library
The library is available directly from the Library Manager of Arduino IDE.
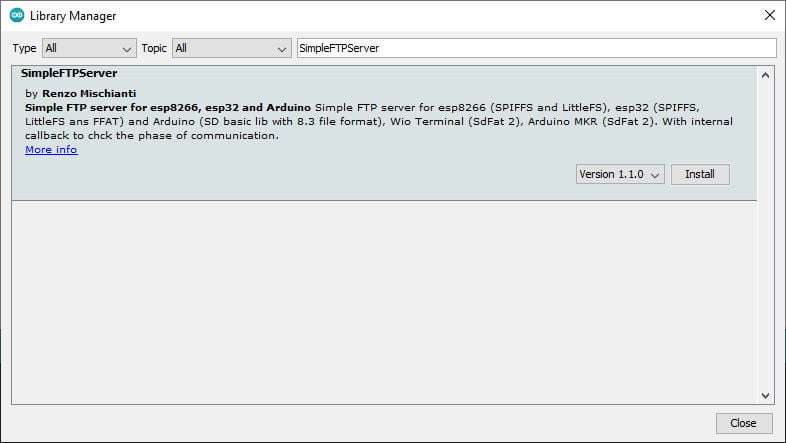
Or the source code from GitHub.
Configuration
For STM32 I already tested 2 network types, one with w5500 and one with enc28j60, and 2 storage solutions, the SD and external SPIFlash.
// STM32 configuration
#ifndef DEFAULT_FTP_SERVER_NETWORK_TYPE_STM32
#define DEFAULT_FTP_SERVER_NETWORK_TYPE_STM32 NETWORK_W5100
#define DEFAULT_STORAGE_TYPE_STM32 STORAGE_SDFAT2
#endif
As you can see the default configuration wants an Ethernet adapter like w5500 (Standard Arduino Ethernet library), and an SD managed by SdFat 2.x library.
But you can choose a wide variety of network devices:
#define NETWORK_ESP8266_ASYNC (1)
#define NETWORK_ESP8266 (2) // Standard ESP8266WiFi
#define NETWORK_ESP8266_242 (3) // ESP8266WiFi before 2.4.2 core
#define NETWORK_W5100 (4) // Standard Arduino Ethernet library
#define NETWORK_ENC28J60 (5) // UIPEthernet library
#define NETWORK_ESP32 (6) // Standard WiFi library
#define NETWORK_ESP32_ETH (7) // Standard ETH library
#define NETWORK_WiFiNINA (8) // Standard WiFiNINA library
#define NETWORK_SEEED_RTL8720DN (9) // Standard SEED WiFi library
#define NETWORK_ETHERNET_LARGE (10)
#define NETWORK_ETHERNET_ENC (11) // EthernetENC library (evolution of UIPEthernet
#define NETWORK_ETHERNET_STM (12)
#define NETWORK_UIPETHERNET (13) // UIPEthernet library same of NETWORK_ENC28J60
In particular, we are going to see the NETWORK_W5100
and NETWORK_ETHERNET_ENC
that is an evolution of the UIPEthernet library.
And here are the storage devices:
#define STORAGE_SDFAT1 1 // Library SdFat version 1.4.x
#define STORAGE_SDFAT2 2 // Library SdFat version >= 2.0.2
#define STORAGE_SPIFM 3 // Libraries Adafruit_SPIFlash and SdFat-Adafruit-Fork
#define STORAGE_FATFS 4 // Library FatFs
#define STORAGE_SD 5 // Standard SD library (suitable for Arduino esp8266 and esp32
#define STORAGE_SPIFFS 6 // SPIFFS
#define STORAGE_LITTLEFS 7 // LITTLEFS
#define STORAGE_SEEED_SD 8 // Seeed_SD library
#define STORAGE_FFAT 9 // ESP32 FFAT
I use and tested STORAGE_SDFAT2
the SD and STORAGE_SPIFM
for the external SPI Flash.
SD card and ethernet w5500
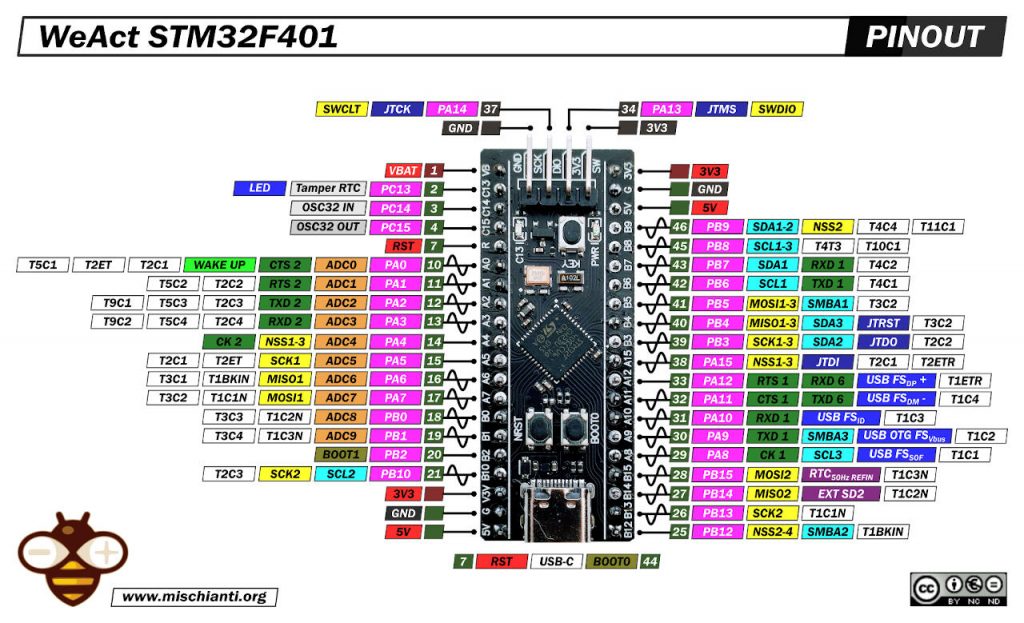
Here my selection of STM32 devices STM32F103C8T6 STM32F401 STM32F411 ST-Link v2 ST-Link v2 official
w5500 is one of the most efficient ethernet devices, we start with this.
STM32F4 with w5500 and SD on the secondary SPI interface
This is the first example, I think It’s the most common situation with a w5500 ethernet controller on primary SPI and SD on the dedicated secondary SPI interface.
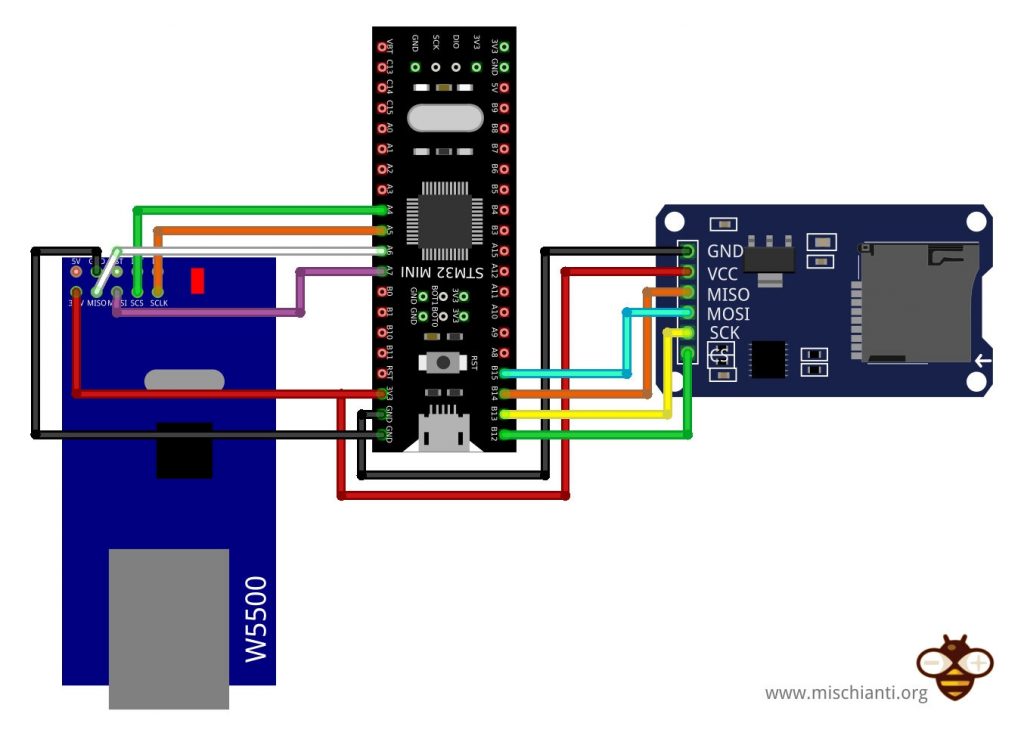
Here my tested ethernet devices w5500 lite - w5500 - enc26j60 mini - enc26j60 - lan8720
STM32 | w5500 SPI1 |
---|---|
PA4 | CS |
PA5 | SCK |
PA6 | MISO |
PA7 | MOSI |
3.3v | 3.3v |
GND | GND |
SD card adapters AliExpress
STM32 | SD card SPI2 |
---|---|
PA12 | CS |
PA13 | SCK |
PA14 | MISO |
PA15 | MOSI |
3.3v | VCC |
GND | GND |
Sketch Example
We are going to create a primary example with w5500 attached to the primary SPI interface and standard SS, and the SD card on the secondary SPI interface.
SimpleFTPServer configuration
As described for the SD card we use SdFat 2.x library and standard Arduino Ethernet for w5500.
#ifndef DEFAULT_FTP_SERVER_NETWORK_TYPE_STM32
#define DEFAULT_FTP_SERVER_NETWORK_TYPE_STM32 NETWORK_W5100
#define DEFAULT_STORAGE_TYPE_STM32 STORAGE_SDFAT2
#endif
Full code
/**
* SimpleFTPServer ^1.3.0 on STM32 (need FLASH > 64K)
* and ethernet w5500
* SD connected on secondary SPI or primary
*
#ifndef DEFAULT_FTP_SERVER_NETWORK_TYPE_STM32
#define DEFAULT_FTP_SERVER_NETWORK_TYPE_STM32 NETWORK_W5100
#define DEFAULT_STORAGE_TYPE_STM32 STORAGE_SDFAT2
#endif
*
* @author Renzo Mischianti <www.mischianti.org>
* @details www.mischianti.org
* @version 0.1
* @date 2022-03-22
*
* @copyright Copyright (c) 2022
*
*/
#include <SdFat.h>
#include <sdios.h>
#include <Ethernet.h>
#include <SimpleFtpServer.h>
// To use SD with primary SPI
// #define SD_CS_PIN PA4
// To use SD with secondary SPI
#define SD_CS_PIN PB12
static SPIClass mySPI2(PB15, PB14, PB13, SD_CS_PIN);
#define SD2_CONFIG SdSpiConfig(SD_CS_PIN, DEDICATED_SPI, SD_SCK_MHZ(18), &mySPI2)
SdFat sd;
FtpServer ftpSrv; //set #define FTP_DEBUG in ESP8266FtpServer.h to see ftp verbose on serial
// Enter a MAC address for your controller below.
// Newer Ethernet shields have a MAC address printed on a sticker on the shield
byte mac[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xED };
// Set the static IP address to use if the DHCP fails to assign
#define MYIPADDR 192,168,1,28
#define MYIPMASK 255,255,255,0
#define MYDNS 192,168,1,1
#define MYGW 192,168,1,1
// Initialize the Ethernet client library
// with the IP address and port of the server
// that you want to connect to (port 80 is default for HTTP):
EthernetClient client;
void setup() {
Serial.begin( 115200 );
while (!Serial) { delay(100); }
Serial.print("\nInitializing SD card...");
// Secondary SPI for SD
if (!sd.begin(SD2_CONFIG)) {
// Primary SPI for SD
// if (!SD.begin(SD_CS_PIN)) {
Serial.println(F("initialization failed. Things to check:"));
Serial.println(F("* is a card inserted?"));
Serial.println(F("* is your wiring correct?"));
Serial.println(F("* did you change the chipSelect pin to match your shield or module?"));
while (1);
} else {
Serial.println(F("Wiring is correct and a card is present."));
}
// Show capacity and free space of SD card
Serial.print(F("Capacity of card: ")); Serial.print(long( sd.card()->sectorCount() >> 1 )); Serial.println(F(" kBytes"));
// You can use Ethernet.init(pin) to configure the CS pin
Ethernet.init(PA4);
if (Ethernet.begin(mac)) { // Dynamic IP setup
Serial.println(F("DHCP OK!"));
}else{
Serial.println(F("Failed to configure Ethernet using DHCP"));
// Check for Ethernet hardware present
if (Ethernet.hardwareStatus() == EthernetNoHardware) {
Serial.println(F("Ethernet shield was not found. Sorry, can't run without hardware. :("));
while (true) {
delay(1); // do nothing, no point running without Ethernet hardware
}
}
if (Ethernet.linkStatus() == LinkOFF) {
Serial.println(F("Ethernet cable is not connected."));
}
IPAddress ip(MYIPADDR);
IPAddress dns(MYDNS);
IPAddress gw(MYGW);
IPAddress sn(MYIPMASK);
Ethernet.begin(mac, ip, dns, gw, sn);
Serial.println("STATIC OK!");
}
delay(5000);
Serial.print("Local IP : ");
Serial.println(Ethernet.localIP());
Serial.print("Subnet Mask : ");
Serial.println(Ethernet.subnetMask());
Serial.print("Gateway IP : ");
Serial.println(Ethernet.gatewayIP());
Serial.print("DNS Server : ");
Serial.println(Ethernet.dnsServerIP());
Serial.println("Ethernet Successfully Initialized");
Serial.println();
// Initialize the FTP server
ftpSrv.begin("user","password");
Serial.println("Ftp server started!");
}
void loop()
{
ftpSrv.handleFTP();
// more processes...
}
You can also use anonymous login with
ftpSrv.begin();
SD Card and ethernet enc28j60
Another popular ethernet device is the enc28j60, very cheap but with some limit on memory, but more than sufficient for our purpose.
STM32F4 with enc28j60 and SD on the primary SPI interface
Another classic situation is the use of all devices on the same SPI interface with the selection of CS. In this configuration, you are going to use a limited set of pins: 5 for all devices.
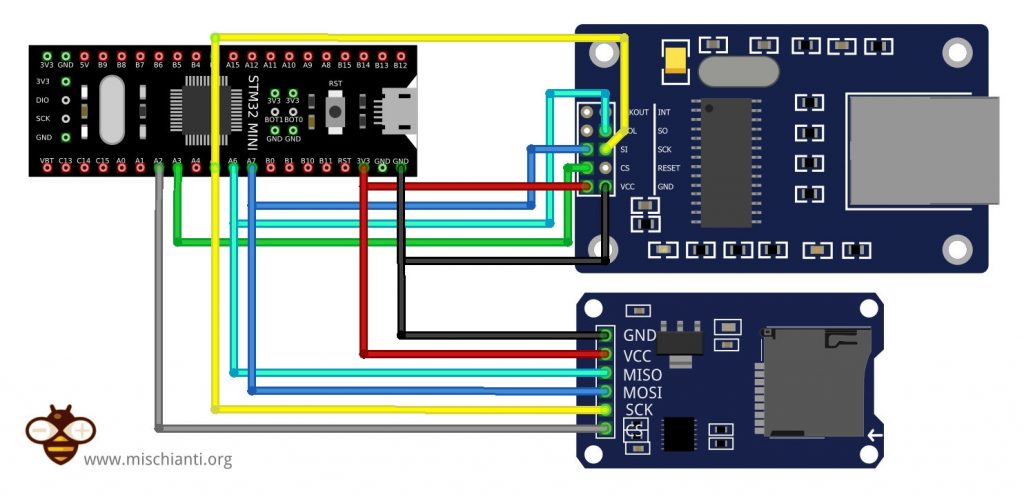
Here my tested ethernet devices w5500 lite - w5500 - enc26j60 mini - enc26j60 - lan8720
Here the SD module I use AliExpress
STM32 | enc28j60 SPI1 |
---|---|
PA3 | CS |
PA5 | SCK |
PA6 | MISO |
PA7 | MOSI |
3.3v | 3.3v |
GND | GND |
SD card adapters AliExpress
STM32 | SD card SPI2 |
---|---|
PA2 | CS |
PA5 | SCK |
PA6 | MISO |
PA7 | MOSI |
3.3v | VCC |
GND | GND |
Sketch example
We are going to use enc28j60 and SD card adapter on the primary SPI device, we change the default CS of Ethernet to PA2 and CS of SD to PA3.
SimpleFTPServer configuration
For SD cards we use SdFat 2.x library and EthernetENC for enc28j60 an evolution of UIPEthernet.
#ifndef DEFAULT_FTP_SERVER_NETWORK_TYPE_STM32
#define DEFAULT_FTP_SERVER_NETWORK_TYPE_STM32 NETWORK_ETHERNET_ENC
#define DEFAULT_STORAGE_TYPE_STM32 STORAGE_SDFAT2
#endif
Full code
/**
* SimpleFTPServer ^1.3.0 on STM32 (need FLASH > 64K)
* and ethernet enc28j60
* SD connected on secondary SPI or primary
*
#ifndef DEFAULT_FTP_SERVER_NETWORK_TYPE_STM32
#define DEFAULT_FTP_SERVER_NETWORK_TYPE_STM32 NETWORK_W5100
#define DEFAULT_STORAGE_TYPE_STM32 STORAGE_SDFAT2
#endif
*
* @author Renzo Mischianti <www.mischianti.org>
* @details www.mischianti.org
* @version 0.1
* @date 2022-03-22
*
* @copyright Copyright (c) 2022
*
*/
#include <SdFat.h>
#include <sdios.h>
#include <SimpleFtpServer.h>
#include <EthernetEnc.h>
// Ethernet CS
#define ETHERNET_CS_PIN PA3
// To use SD with primary SPI
#define SD_CS_PIN PA2
// To use SD with secondary SPI
// #define SD_CS_PIN PB12
// static SPIClass mySPI2(PB15, PB14, PB13, SD_CS_PIN);
// #define SD2_CONFIG SdSpiConfig(SD_CS_PIN, DEDICATED_SPI, SD_SCK_MHZ(18), &mySPI2)
SdFat sd;
FtpServer ftpSrv; //set #define FTP_DEBUG in ESP8266FtpServer.h to see ftp verbose on serial
// Enter a MAC address for your controller below.
// Newer Ethernet shields have a MAC address printed on a sticker on the shield
byte mac[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xED };
// Set the static IP address to use if the DHCP fails to assign
#define MYIPADDR 192,168,1,28
#define MYIPMASK 255,255,255,0
#define MYDNS 192,168,1,1
#define MYGW 192,168,1,1
// Initialize the Ethernet client library
// with the IP address and port of the server
// that you want to connect to (port 80 is default for HTTP):
EthernetClient client;
void setup() {
Serial.begin( 115200 );
while (!Serial) { delay(100); }
pinMode( SD_CS_PIN, OUTPUT );
digitalWrite( SD_CS_PIN, HIGH );
pinMode( ETHERNET_CS_PIN, OUTPUT );
digitalWrite( ETHERNET_CS_PIN, HIGH );
Serial.print("\nInitializing SD card...");
// To use SD with secondary SPI
// if (!sd.begin(SD2_CONFIG)) {
// To use SD with primary SPI
if (!sd.begin(SD_CS_PIN)) {
Serial.println(F("initialization failed. Things to check:"));
Serial.println(F("* is a card inserted?"));
Serial.println(F("* is your wiring correct?"));
Serial.println(F("* did you change the chipSelect pin to match your shield or module?"));
while (1);
} else {
Serial.println(F("Wiring is correct and a card is present."));
}
// Show capacity and free space of SD card
Serial.print(F("Capacity of card: ")); Serial.print(long( sd.card()->sectorCount() >> 1 )); Serial.println(F(" kBytes"));
// You can use Ethernet.init(pin) to configure the CS pin
Ethernet.init(ETHERNET_CS_PIN);
if (Ethernet.begin(mac)) { // Dynamic IP setup
Serial.println(F("DHCP OK!"));
}else{
Serial.println(F("Failed to configure Ethernet using DHCP"));
// Check for Ethernet hardware present
if (Ethernet.hardwareStatus() == EthernetNoHardware) {
Serial.println(F("Ethernet shield was not found. Sorry, can't run without hardware. :("));
while (true) {
delay(1); // do nothing, no point running without Ethernet hardware
}
}
if (Ethernet.linkStatus() == LinkOFF) {
Serial.println(F("Ethernet cable is not connected."));
}
IPAddress ip(MYIPADDR);
IPAddress dns(MYDNS);
IPAddress gw(MYGW);
IPAddress sn(MYIPMASK);
Ethernet.begin(mac, ip, dns, gw, sn);
Serial.println("STATIC OK!");
}
delay(5000);
Serial.print("Local IP : ");
Serial.println(Ethernet.localIP());
Serial.print("Subnet Mask : ");
Serial.println(Ethernet.subnetMask());
Serial.print("Gateway IP : ");
Serial.println(Ethernet.gatewayIP());
Serial.print("DNS Server : ");
Serial.println(Ethernet.dnsServerIP());
Serial.println("Ethernet Successfully Initialized");
Serial.println();
// Initialize the FTP server
ftpSrv.begin("user","password");
Serial.println("Ftp server started!");
}
void loop()
{
ftpSrv.handleFTP();
// more processes...
}
SPI Flash and Ethernet w5500
For these tests, you can follow the relative SPI Flash article “STM32: SPI flash memory FAT FS”, and we use the embedded footprint of SPI Flash of STM32F4 Black-pill.
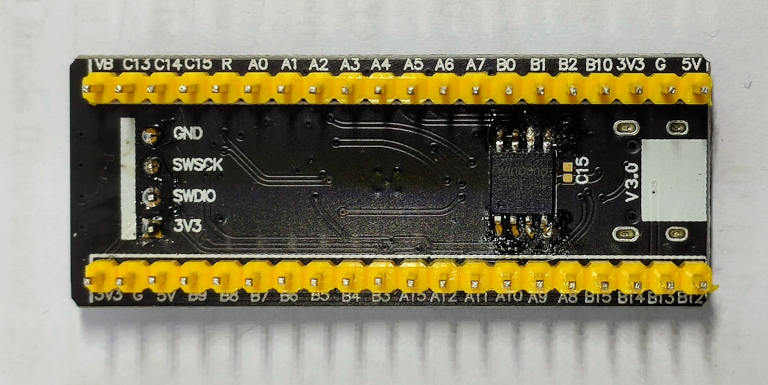
Here a set of tested SPI Flash w25q16 SMD 2Mb - w25q16 Discrete 2Mb - w25q32 SMD 4Mb - w25q32 Discrete 4Mb - w25q64 SMD 8Mb - w25q64 Discrete 8Mb - w25q128 SMD 16Mb - w25q128 Discrete 16Mb W25Q32 W25Q64 w25q128 module 4Mb 8Mb 16Mb
Wiring w5500
Now we use only the Ethernet w5500 on primary SPI, but pay attention you can’t use the PA4 pin like the schema, because It’s used by SPI Flash on the footprint.
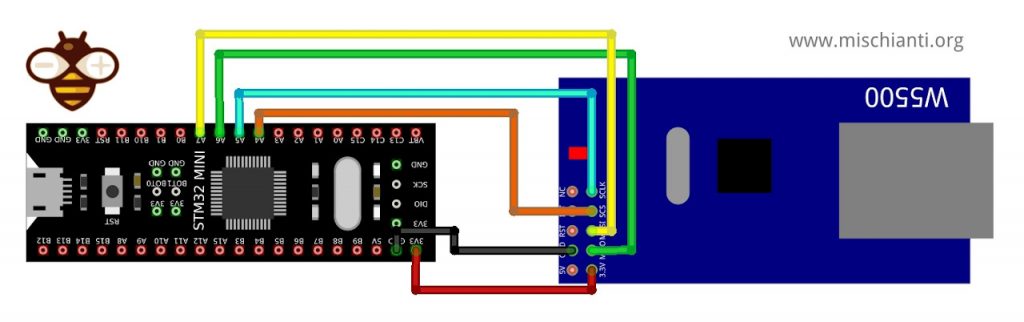
Here my tested ethernet devices w5500 lite - w5500 - enc26j60 mini - enc26j60 - lan8720
STM32 | w5500 SPI1 |
---|---|
PA3 | CS |
PA5 | SCK |
PA6 | MISO |
PA7 | MOSI |
3.3v | 3.3v |
GND | GND |
STM32 | SPI Flash |
---|---|
PA4 | CS |
PA5 | SCK |
PA6 | MISO |
PA7 | MOSI |
3.3v | VCC |
GND | GND |
Sketch example
Pay attention to the CS pins. PA4 for SPI Flash and PA3 on Ethernet w5500.
SimpleFTPServer configuration
For SPI Flash, as described in the relative SPI Flash article we use Adafruit_SPIFlash and SdFat-Adafruit-Fork library and standard Arduino Ethernet for w5500.
#ifndef DEFAULT_FTP_SERVER_NETWORK_TYPE_STM32
#define DEFAULT_FTP_SERVER_NETWORK_TYPE_STM32 NETWORK_W5100
#define DEFAULT_STORAGE_TYPE_STM32 STORAGE_SPIFM
#endif
Full code
/**
* SimpleFTPServer ^1.3.0 on STM32 (need FLASH > 64K)
* and ethernet w5500
* SPI Flash with Adafruit_SPIFlash and SdFat-Adafruit-Fork library
*
#ifndef DEFAULT_FTP_SERVER_NETWORK_TYPE_STM32
#define DEFAULT_FTP_SERVER_NETWORK_TYPE_STM32 NETWORK_W5100
#define DEFAULT_STORAGE_TYPE_STM32 STORAGE_SPIFM
#endif
*
* @author Renzo Mischianti <www.mischianti.org>
* @details www.mischianti.org
* @version 0.1
* @date 2022-03-22
*
* @copyright Copyright (c) 2022
*
*/
#include <Arduino.h>
#include <Ethernet.h>
#include "SdFat.h"
#include "Adafruit_SPIFlash.h"
#include <SimpleFTPServer.h>
Adafruit_FlashTransport_SPI flashTransport(SS, SPI); // Set CS and SPI interface
Adafruit_SPIFlash flash(&flashTransport);
// file system object from SdFat
FatFileSystem fatfs;
#define ETHERNET_CS_PIN PA3
FtpServer ftpSrv; //set #define FTP_DEBUG in ESP8266FtpServer.h to see ftp verbose on serial
// Enter a MAC address for your controller below.
// Newer Ethernet shields have a MAC address printed on a sticker on the shield
byte mac[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xED };
// Set the static IP address to use if the DHCP fails to assign
#define MYIPADDR 192,168,1,28
#define MYIPMASK 255,255,255,0
#define MYDNS 192,168,1,1
#define MYGW 192,168,1,1
// Initialize the Ethernet client library
// with the IP address and port of the server
// that you want to connect to (port 80 is default for HTTP):
EthernetClient client;
void setup() {
// Initialize serial port and wait for it to open before continuing.
Serial.begin(115200);
while (!Serial) {
delay(100);
}
Serial.println("Adafruit SPI Flash FatFs Full Usage Example");
// Initialize flash library and check its chip ID.
if (!flash.begin()) {
Serial.println("Error, failed to initialize flash chip!");
while(1) yield();
}
Serial.print("JEDEC ID: "); Serial.println(flash.getJEDECID(), HEX);
Serial.print("Flash size: "); Serial.println(flash.size());
Serial.flush();
// First call begin to mount the filesystem. Check that it returns true
// to make sure the filesystem was mounted.
if (!fatfs.begin(&flash)) {
Serial.println("Error, failed to mount newly formatted filesystem!");
Serial.println("Was the flash chip formatted with the SdFat_format example?");
while(1) yield();
}
Serial.println("Mounted filesystem!");
Serial.println();
// You can use Ethernet.init(pin) to configure the CS pin
Ethernet.init(ETHERNET_CS_PIN);
if (Ethernet.begin(mac)) { // Dynamic IP setup
Serial.println(F("DHCP OK!"));
}else{
Serial.println(F("Failed to configure Ethernet using DHCP"));
// Check for Ethernet hardware present
if (Ethernet.hardwareStatus() == EthernetNoHardware) {
Serial.println(F("Ethernet shield was not found. Sorry, can't run without hardware. :("));
while (true) {
delay(1); // do nothing, no point running without Ethernet hardware
}
}
if (Ethernet.linkStatus() == LinkOFF) {
Serial.println(F("Ethernet cable is not connected."));
}
IPAddress ip(MYIPADDR);
IPAddress dns(MYDNS);
IPAddress gw(MYGW);
IPAddress sn(MYIPMASK);
Ethernet.begin(mac, ip, dns, gw, sn);
Serial.println("STATIC OK!");
}
delay(5000);
Serial.print("Local IP : ");
Serial.println(Ethernet.localIP());
Serial.print("Subnet Mask : ");
Serial.println(Ethernet.subnetMask());
Serial.print("Gateway IP : ");
Serial.println(Ethernet.gatewayIP());
Serial.print("DNS Server : ");
Serial.println(Ethernet.dnsServerIP());
Serial.println("Ethernet Successfully Initialized");
Serial.println();
// Initialize the FTP server
ftpSrv.begin("user","password");
Serial.println("Ftp server started!");
}
void loop()
{
ftpSrv.handleFTP();
// more processes...
}
SPI Flash and Ethernet enc28j60
Wiring enc28j60
We use the same wiring of w5500 for SPI and CS.
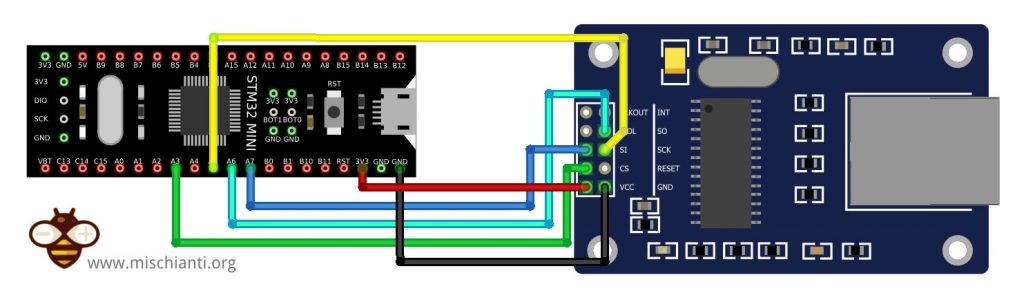
STM32 | enc28j60 SPI1 |
---|---|
PA3 | CS |
PA5 | SCK |
PA6 | MISO |
PA7 | MOSI |
3.3v | 3.3v |
GND | GND |
STM32 | SPI Flash |
---|---|
PA4 | CS |
PA5 | SCK |
PA6 | MISO |
PA7 | MOSI |
3.3v | VCC |
GND | GND |
Sketch example
Pay attention to the CS pins. PA4 for SPI Flash and PA3 on Ethernet enc28j60.
SimpleFTPServer configuration
For SPI Flash, as described in the relative SPI Flash article we use Adafruit_SPIFlash and SdFat-Adafruit-Fork library and EthernetENC for Ethernet enc28j60.
#ifndef DEFAULT_FTP_SERVER_NETWORK_TYPE_STM32
#define DEFAULT_FTP_SERVER_NETWORK_TYPE_STM32 NETWORK_ETHERNET_ENC
#define DEFAULT_STORAGE_TYPE_STM32 STORAGE_SPIFM
#endif
Full code
/**
* SimpleFTPServer ^1.3.0 on STM32 (need FLASH > 64K)
* and ethernet enc28j60 with EthernetENC
* SPI Flash with Adafruit_SPIFlash and SdFat-Adafruit-Fork library
*
#ifndef DEFAULT_FTP_SERVER_NETWORK_TYPE_STM32
#define DEFAULT_FTP_SERVER_NETWORK_TYPE_STM32 NETWORK_ETHERNET_ENC
#define DEFAULT_STORAGE_TYPE_STM32 STORAGE_SPIFM
#endif
*
* @author Renzo Mischianti <www.mischianti.org>
* @details www.mischianti.org
* @version 0.1
* @date 2022-03-22
*
* @copyright Copyright (c) 2022
*
*/
#include <Arduino.h>
#include <EthernetENC.h>
#include "SdFat.h"
#include "Adafruit_SPIFlash.h"
#include <SimpleFTPServer.h>
Adafruit_FlashTransport_SPI flashTransport(SS, SPI); // Set CS and SPI interface
Adafruit_SPIFlash flash(&flashTransport);
// file system object from SdFat
FatFileSystem fatfs;
#define ETHERNET_CS_PIN PA3
FtpServer ftpSrv; //set #define FTP_DEBUG in ESP8266FtpServer.h to see ftp verbose on serial
// Enter a MAC address for your controller below.
// Newer Ethernet shields have a MAC address printed on a sticker on the shield
byte mac[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xED };
// Set the static IP address to use if the DHCP fails to assign
#define MYIPADDR 192,168,1,28
#define MYIPMASK 255,255,255,0
#define MYDNS 192,168,1,1
#define MYGW 192,168,1,1
// Initialize the Ethernet client library
// with the IP address and port of the server
// that you want to connect to (port 80 is default for HTTP):
EthernetClient client;
void setup() {
// Initialize serial port and wait for it to open before continuing.
Serial.begin(115200);
while (!Serial) {
delay(100);
}
Serial.println("Adafruit SPI Flash FatFs Full Usage Example");
// Initialize flash library and check its chip ID.
if (!flash.begin()) {
Serial.println("Error, failed to initialize flash chip!");
while(1) yield();
}
Serial.print("JEDEC ID: "); Serial.println(flash.getJEDECID(), HEX);
Serial.print("Flash size: "); Serial.println(flash.size());
Serial.flush();
// First call begin to mount the filesystem. Check that it returns true
// to make sure the filesystem was mounted.
if (!fatfs.begin(&flash)) {
Serial.println("Error, failed to mount newly formatted filesystem!");
Serial.println("Was the flash chip formatted with the SdFat_format example?");
while(1) yield();
}
Serial.println("Mounted filesystem!");
Serial.println();
// You can use Ethernet.init(pin) to configure the CS pin
Ethernet.init(ETHERNET_CS_PIN);
if (Ethernet.begin(mac)) { // Dynamic IP setup
Serial.println(F("DHCP OK!"));
}else{
Serial.println(F("Failed to configure Ethernet using DHCP"));
// Check for Ethernet hardware present
if (Ethernet.hardwareStatus() == EthernetNoHardware) {
Serial.println(F("Ethernet shield was not found. Sorry, can't run without hardware. :("));
while (true) {
delay(1); // do nothing, no point running without Ethernet hardware
}
}
if (Ethernet.linkStatus() == LinkOFF) {
Serial.println(F("Ethernet cable is not connected."));
}
IPAddress ip(MYIPADDR);
IPAddress dns(MYDNS);
IPAddress gw(MYGW);
IPAddress sn(MYIPMASK);
Ethernet.begin(mac, ip, dns, gw, sn);
Serial.println("STATIC OK!");
}
delay(5000);
Serial.print("Local IP : ");
Serial.println(Ethernet.localIP());
Serial.print("Subnet Mask : ");
Serial.println(Ethernet.subnetMask());
Serial.print("Gateway IP : ");
Serial.println(Ethernet.gatewayIP());
Serial.print("DNS Server : ");
Serial.println(Ethernet.dnsServerIP());
Serial.println("Ethernet Successfully Initialized");
Serial.println();
// Initialize the FTP server
ftpSrv.begin("user","password");
Serial.println("Ftp server started!");
}
void loop()
{
ftpSrv.handleFTP();
// more processes...
}
Thanks
- STM32F1 Blue-Pill: pinout, specs, and Arduino IDE configuration (STM32duino and STMicroelectronics)
- STM32: program (STM32F1) via USB with STM32duino bootloader
- STM32: programming (STM32F1 STM32F4) via USB with HID boot-loader
- STM32F4 Black-Pill: pinout, specs, and Arduino IDE configuration
- STM32: ethernet w5500 with plain HTTP and SSL (HTTPS)
- STM32: ethernet enc28j60 with plain HTTP and SSL (HTTPS)
- STM32: WiFiNINA with ESP32 WiFi Co-Processor
- How to use SD card with stm32 and SdFat library
- \STM32: SPI flash memory FAT FS
- STM32: internal RTC, clock, and battery backup (VBAT)
- STM32 LoRa
- STM32 Power saving
- STM32F1 Blue-Pill clock and frequency management
- STM32F4 Black-Pill clock and frequency management
- Intro and Arduino vs STM framework
- Library LowPower, wiring, and Idle (STM Sleep) mode
- Sleep, deep sleep, shutdown, and power consumption
- Wake up from RTC alarm and Serial
- Wake up from the external source
- Backup domain intro and variable preservation across reset
- RTC backup register and SRAM preservation
- STM32 send emails with attachments and SSL (like Gmail): w5500, enc28j60, SD, and SPI Fash
- FTP server on STM32 with w5500, enc28j60, SD Card, and SPI Flash
- Connecting the EByte E70 to STM32 (black/blue pill) devices and a simple sketch example
- SimpleFTPServer library: esp32 and esp8266 how to
- SimpleFTPServer library: WioTerminal how to
- FTP server on STM32 with w5500, enc28j60, SD Card, and SPI Flash