STM32: programming (STM32F1) via USB with STM32duino boot-loader – 2
We continue exploring the STM32 family of 32-bit microcontrollers based on the Arm® Cortex®-M processor.
STM32duino-bootloader is created for STM32F103 boards to use with the Arduino_Core_STM32 repo and the Arduino IDE; very useful to program the microcontroller directly via USB.
First of all, read the previous article, “STM32F1: pinout, specs, and Arduino IDE configuration (STM32duino and STMicroelectronics)” where you can find out how to configure your Arduino IDE, and pay attention: this bootloader wants the STM32duino URL descriptor (first part of the previous article).
Here the most commons STM32 and ST-Link STM32F103C8T6 STM32F401 STM32F411 ST-Link v2 ST-Link v2 official
As already described, you need an FTDI programmer or ST-Link. I usually use a basic model, but in this case, It’s more simple to use a module with integrated power output.
The more expensive FT232RL or FT232 module can power the microcontroller, but a CH340G or CH340 works well.
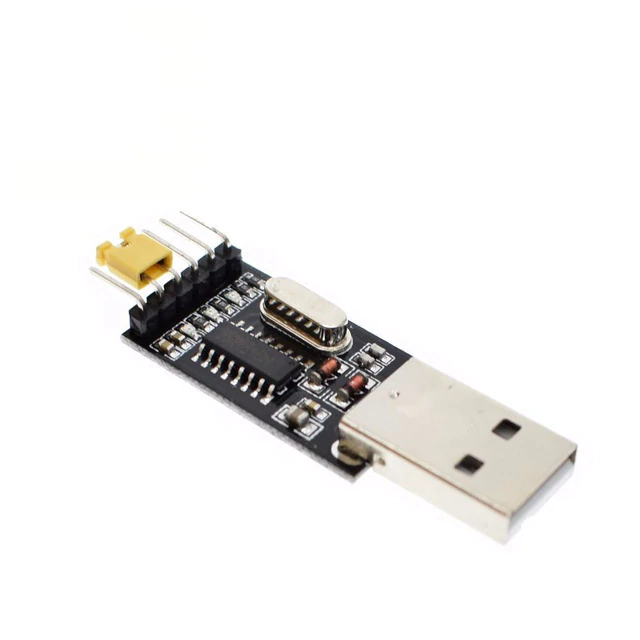
Here the two model USB to TTL CH340G - USB to TTL FT232RL
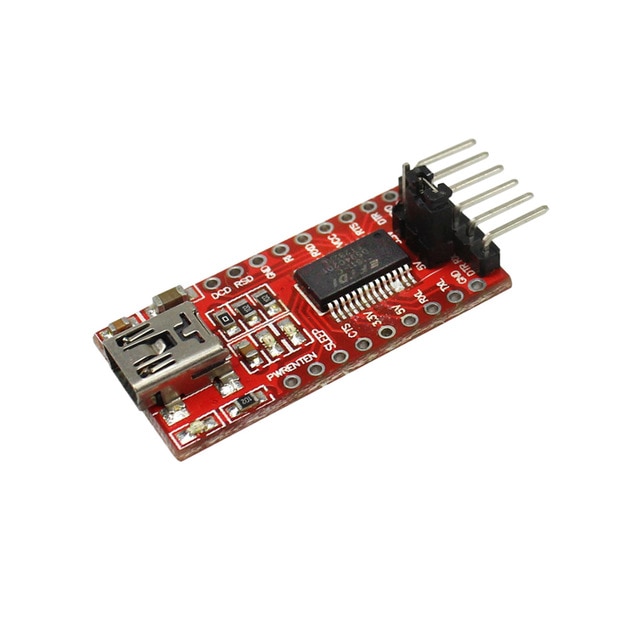
Connection schema with FTDI
To upload the bootloader, you need to connect the FTDI, as explained in this image.
You can power the stm32 with 3.3v or 5v in the respective pin, and you must connect FTDI TX to PA10 and RX to PA9.
STM32F103C8T6 | FTDI |
---|---|
5v or 3.3v | VCC 5v or 3.3v |
GND | GND |
A9 | RX |
A10 | TX |
Connection schema with ST-Link
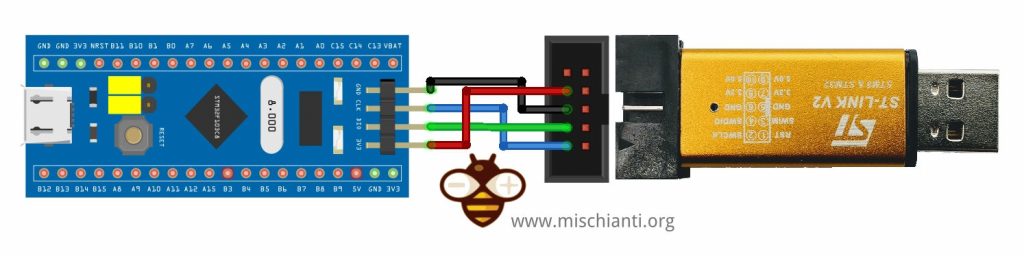
STM32 | ST-Link v2 |
---|---|
GND | GND |
SCK | SWCLK |
DIO | SWDIO |
3V3 | 3V3 |
Boot modalities
You can select three types of boot mode:
- Boot from System Memory: invokes the on-chip bootloader, which is present in the chip directly from the factory before you’ve programmed anything into the on-chip flash. This allows you to load (program) code into the device from an external interface such as UART or USB.
- Main flash memory: this is where your code typically goes. In a typical operation, your code will reside in a flash, and on Power-On Reset (POR), the CPU will fetch the reset vector and initial stack pointer (SP) from flash. You can load flash via JTAG, the on-chip bootloader (above), etc.
- Load code into RAM (JTAG, runtime) and then boot/run from there. This isn’t often used; usually, you’re doing something tricky like a temporary bootloader or the like.
Here is the table with jumper configuration:
BOOT0 | BOOT1 | Modality |
---|---|---|
0 | X | Main flash memory |
1 | 0 | System Memory |
1 | 1 | Embedded SRAM |
Upload STM32duino bootloader with STM32 Flash loader
To upload the bootloader, we must put the device in “System Memory”.
- We are going to use the STM32 Flash loader demonstrator (UM0462) that is now replaced by STM32CubeProgrammer, but It’s very simple and fast. You can download It from here.
- Then you need the bootloader; we download It from this repository, in my case, I need the
STM32duino-bootloader/binaries/generic_boot20_pc13.bin
You must select the firmware generic_boot20 and the correct LED pin, for me, the PC13 or PA13 pin. - Start the Flash Loader Demonstrator and select the correct COM port.
- In the next step, you can verify the size of your device’s flash
- Next, you must select the correct device (very important).
- It’s time to choose the bootloader file yet downloaded.
- And finally, the upload.
Now restore the jumper to normal position and restart. At this time, if you connect the device via USB, It isn’t recognized; you must install the drivers.
Upload STM32duino bootloader with STM32CubeProgrammer
You can flash the previous STM32duino bootloader with the STM32CubeProgrammer.
For ST-Link, you must select ST-LINK instead of UART, nothing more.
- Install the STM32CubeProgrammer released from STMicroelectronics. You can download It from here;
- Then you need the bootloader; we download It from this repository, in my case, I need the STM32duino-bootloader/binaries/generic_boot20_pc13.bin
You must select the firmware generic_boot20 and the correct LED pin, for me, the PC13 or PA13 pin. - Start the program;
- Select UART connection type;
- Select the correct COM port;
- Click Connect;
- Click on Erase & Programming icon (green arrow);
- Select the correct STM32duino bootloader (as described previously);
- Start programming
Driver installation
To get the device recognized from Windows, you must install the relative driver.
From: Arduino_STM32/drivers/win/ you must download these files:
and execute the first 2. After that, if all It’s ok, you get the device in your COM port.
You get these messages if you open the serial monitor on the new COM port.
Congratulations, you have installed the STM32duino bootloader
See https://github.com/rogerclarkmelbourne/STM32duino-bootloader
For more information about Arduino on STM32
See https://www.stm32duino.com
Upload the sketch
Here is a simple Blink sketch for the devices with LED pin of PA13.
/*
Blink for STM32F1xx
Turns an LED on for one second, then off for one second, repeatedly.
http://www.mischianti.org
*/
void setup() {
// Open serial communications and wait for port to open:
Serial.begin(115200);
while (!Serial) {
; // wait for serial port to connect. Needed for native USB port only
}
Serial.print(F("Serial OK!"));
pinMode(PC13, OUTPUT);
}
void loop() {
digitalWrite(PC13, HIGH);
delay(1000);
digitalWrite(PC13, LOW);
delay(1000);
}
We must select the SMT32duino bootloader.
Here is the output of the sketch upload.
C:\Users\renzo\AppData\Local\Arduino15\packages\stm32duino\tools\stm32tools\2021.5.31/win/maple_upload.bat COM27 2 1EAF:0003 C:\Users\renzo\AppData\Local\Temp\arduino_build_965206/Blink.ino.bin C:\Program Files (x86)\Arduino
maple_loader v0.1
Resetting to bootloader via DTR pulse
Reset via USB Serial Failed! Did you select the right serial port?
Searching for DFU device [1EAF:0003]...
Assuming the board is in perpetual bootloader mode and continuing to attempt dfu programming...
Found it!
Opening USB Device 0x1eaf:0x0003...
Found Runtime: [0x1eaf:0x0003] devnum=1, cfg=0, intf=0, alt=2, name="STM32duino bootloader v1.0 Upload to Flash 0x8002000"
Setting Configuration 1...
Claiming USB DFU Interface...
Setting Alternate Setting ...
Determining device status: state = dfuIDLE, status = 0
dfuIDLE, continuing
Transfer Size = 0x0400
bytes_per_hash=287
Starting download: [##################################################] finished!
state(8) = dfuMANIFEST-WAIT-RESET, status(0) = No error condition is present
error resetting after download: usb_reset: could not reset device, win error: � stato specificato un dispositivo inesistente.
Done!
Resetting USB to switch back to runtime mode
You must connect the Serial monitor to start the sketch.
Here is the Serial output.
Serial OK!
Thanks
- STM32F1 Blue-Pill: pinout, specs, and Arduino IDE configuration (STM32duino and STMicroelectronics)
- STM32: program (STM32F1) via USB with STM32duino bootloader
- STM32: programming (STM32F1 STM32F4) via USB with HID boot-loader
- STM32F4 Black-Pill: pinout, specs, and Arduino IDE configuration
- STM32: ethernet w5500 with plain HTTP and SSL (HTTPS)
- STM32: ethernet enc28j60 with plain HTTP and SSL (HTTPS)
- STM32: WiFiNINA with ESP32 WiFi Co-Processor
- How to use SD card with stm32 and SdFat library
- \STM32: SPI flash memory FAT FS
- STM32: internal RTC, clock, and battery backup (VBAT)
- STM32 LoRa
- STM32 Power saving
- STM32F1 Blue-Pill clock and frequency management
- STM32F4 Black-Pill clock and frequency management
- Intro and Arduino vs STM framework
- Library LowPower, wiring, and Idle (STM Sleep) mode
- Sleep, deep sleep, shutdown, and power consumption
- Wake up from RTC alarm and Serial
- Wake up from the external source
- Backup domain intro and variable preservation across reset
- RTC backup register and SRAM preservation
- STM32 send emails with attachments and SSL (like Gmail): w5500, enc28j60, SD, and SPI Fash
Thank you for this tutorial.
After connecting Blue Pill and running Flash Loader, when I click on Next Button
I get this communicate:
“No response from the targer, the Boot loader can not be started.
Please, verify the boot mode configuration and the flash protection status.
Reset your device then try again…”
Boot0 Jumper is on position 1
Hi Pawel,
It’s very strange, try to do It with STM32CubeProg, you can select various upload mode and connection.
Bye Renzo
It was my mistake 🙁
I changed TX with RX on BluePill.
I have another question.
I’ve bought Blue Pill to install RotorHazard FW on it https://github.com/RotorHazard/RotorHazard/tree/v4.0.0/firmware
Should I install STM3232duino bootloader first or just flash it with RH_S32_BPill_node.bin with BOOT0 jumper on position 1?
Is Boot loader only required for Blue Pill to be visible as COM port while connected with USB port?
Or maybe bootloader is required for all operations?
Hi Pawel,
boot loader is needed only to program the device via USB, but if you use ST-Link you don’t need It.
Bye Renzo
One more question 😉
Do I always need BOOT0 jumper to position 1 for flashing BP?
Or it is only required to upload bootloader?
You can find more info here.
However, the answer is negative. When the jumpers are set to position 0, you update the main flash memory where the sketch/program is uploaded. When boot0 is set to 1, you write to the system memory where the bootloader resides.
Bye Renzo
Sorry for bothering 😉
Does the method of uploading Application to BPill:
a. Arduino IDE/ USB Connection to onboard microUSB)/ BOOT0 on position 0
b. STM32CubeProgramer/ USB to UART Converter (FT232)/ BOOT0 on position 1
have any impact for the application?
I understand that option b) will put Application to the lower ROM region and overwrite Bootloader, but will it have any influence for use of appliaction?
With option a) Arduino IDE uploads the Application to upper ROM region and leaves Bootloader untouched.
Can we assume that one option is better for any reason than another?
(ie. with option b. we have more space to fill, while option a. gaves possibility to programm BPill with simple USB Cable)
Hi Pawel,
don’t worry It’s my pleasure.
I’m not sure (I don’t test It directly) but basically:
In summary, the choice between these methods depends on your specific needs and preferences:
Bye Renzo
Thanks Renzo,
for now I know eoungh I need.
But I will come back if I encounter any problems with my build 😉
Thank you for your help!